
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
GapScanningParams.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_GAP_SCANNING_PARAMS_H__ 00018 #define MBED_GAP_SCANNING_PARAMS_H__ 00019 00020 /** 00021 * @addtogroup ble 00022 * @{ 00023 * @addtogroup gap 00024 * @{ 00025 */ 00026 00027 /** 00028 * Parameters defining the scan process. 00029 * 00030 * Four distinct parameters define the scan procedure: 00031 * - Scan window: Period during which the scanner listens to advertising 00032 * channels. That value is in the range of 2.5ms to 10.24s. This value 00033 * can be set at construction time, updated by calling setWindow() and 00034 * retrieved by invoking getWindow(). 00035 * 00036 * - Scan interval: Interval between the start of two consecutive scan windows. 00037 * That value shall be greater or equal to the scan window value. The 00038 * maximum allowed value is 10.24ms. The scan interval value can be set at 00039 * construction time, updated with a call to setInterval() and queried by a 00040 * call to getInterval(). 00041 * 00042 * - Timeout: The duration of the scan procedure if any. It can be set at 00043 * construction time, updated with setTimeout() and obtained from 00044 * getTimeout(). 00045 * 00046 * - Active scanning: If set, then the scanner sends scan requests to a scannable 00047 * or connectable advertiser. Advertisers may respond to the scan request 00048 * by a scan response containing the scan response payload. If not set, then 00049 * the scanner does not send any request. This flag is set at construction 00050 * time, may be updated with the help of setActiveScanning() and retrieved 00051 * by getActiveScanning(). 00052 * 00053 * @note If the scan window's duration is equal to the scan interval, then the 00054 * device listens continuously during the scan procedure. 00055 */ 00056 class GapScanningParams { 00057 public: 00058 /** 00059 * Minimum Scan interval in 625us units - 2.5ms. 00060 */ 00061 static const unsigned SCAN_INTERVAL_MIN = 0x0004; 00062 00063 /** 00064 * Maximum Scan interval in 625us units - 10.24s. 00065 */ 00066 static const unsigned SCAN_INTERVAL_MAX = 0x4000; 00067 00068 /** 00069 * Minimum Scan window in 625us units - 2.5ms. 00070 */ 00071 static const unsigned SCAN_WINDOW_MIN = 0x0004; 00072 00073 /** 00074 * Maximum Scan window in 625us units - 10.24s. 00075 */ 00076 static const unsigned SCAN_WINDOW_MAX = 0x4000; 00077 00078 /** 00079 * Minimum Scan duration in seconds. 00080 */ 00081 static const unsigned SCAN_TIMEOUT_MIN = 0x0001; 00082 00083 /** 00084 * Maximum Scan duration in seconds. 00085 */ 00086 static const unsigned SCAN_TIMEOUT_MAX = 0xFFFF; 00087 00088 public: 00089 /** 00090 * Construct an instance of GapScanningParams. 00091 * 00092 * @param[in] interval Milliseconds interval between the start of two 00093 * consecutive scan windows. The value passed is between the scan 00094 * window value and 10.24 seconds. 00095 * 00096 * @param[in] window Milliseconds period during which the device 00097 * listens to advertising channels. The value of the scan window is in 00098 * the range of 2.5ms to 10.24s. 00099 * 00100 * @param[in] timeout Duration in seconds of the scan procedure. The special 00101 * value 0 may be used when the scan procedure is not time bounded. 00102 * 00103 * @param[in] activeScanning If true, then the scanner sends scan requests to 00104 * to scannable or connectable advertiser. Advertisers may respond to the 00105 * scan request by a scan response containing the scan response payload. If 00106 * false, the scanner does not send any request. 00107 * 00108 * @note If interval is equal to window 00109 */ 00110 GapScanningParams( 00111 uint16_t interval = SCAN_INTERVAL_MAX, 00112 uint16_t window = SCAN_WINDOW_MAX, 00113 uint16_t timeout = 0, 00114 bool activeScanning = false 00115 ); 00116 00117 /** 00118 * Number of microseconds in 0.625 milliseconds. 00119 */ 00120 static const uint16_t UNIT_0_625_MS = 625; 00121 00122 /** 00123 * Convert milliseconds to units of 0.625ms. 00124 * 00125 * @param[in] durationInMillis Milliseconds to convert. 00126 * 00127 * @return The value of @p durationInMillis in units of 0.625ms. 00128 */ 00129 static uint16_t MSEC_TO_SCAN_DURATION_UNITS(uint32_t durationInMillis) 00130 { 00131 return (durationInMillis * 1000) / UNIT_0_625_MS; 00132 } 00133 00134 /** 00135 * Update the scan interval. 00136 * 00137 * @param[in] newIntervalInMS New scan interval in milliseconds. 00138 * 00139 * @return BLE_ERROR_NONE if the new scan interval was set successfully. 00140 */ 00141 ble_error_t setInterval(uint16_t newIntervalInMS); 00142 00143 /** 00144 * Update the scan window. 00145 * 00146 * @param[in] newWindowInMS New scan window in milliseconds. 00147 * 00148 * @return BLE_ERROR_NONE if the new scan window was set successfully. 00149 */ 00150 ble_error_t setWindow(uint16_t newWindowInMS); 00151 00152 /** 00153 * Update the scan timeout. 00154 * 00155 * @param[in] newTimeout New scan timeout in seconds. 00156 * 00157 * @return BLE_ERROR_NONE if the new scan window was set successfully. 00158 */ 00159 ble_error_t setTimeout(uint16_t newTimeout); 00160 00161 /** 00162 * Update the active scanning flag. 00163 * 00164 * @param[in] activeScanning New boolean value of active scanning. 00165 */ 00166 void setActiveScanning(bool activeScanning); 00167 00168 public: 00169 /** 00170 * Get the scan interval. 00171 * 00172 * @return the scan interval in units of 0.625ms. 00173 */ 00174 uint16_t getInterval(void) const 00175 { 00176 return _interval; 00177 } 00178 00179 /** 00180 * Get the scan window. 00181 * 00182 * @return the scan window in units of 0.625ms. 00183 */ 00184 uint16_t getWindow(void) const 00185 { 00186 return _window; 00187 } 00188 00189 /** 00190 * Get the scan timeout. 00191 * 00192 * @return The scan timeout in seconds. 00193 */ 00194 uint16_t getTimeout(void) const 00195 { 00196 return _timeout; 00197 } 00198 00199 /** 00200 * Check whether active scanning is set. 00201 * 00202 * @return True if active scanning is set, false otherwise. 00203 */ 00204 bool getActiveScanning(void) const 00205 { 00206 return _activeScanning; 00207 } 00208 00209 private: 00210 /** 00211 * Scan interval in units of 625us (between 2.5ms and 10.24s). 00212 */ 00213 uint16_t _interval; 00214 00215 /** 00216 * Scan window in units of 625us (between 2.5ms and 10.24s). 00217 */ 00218 uint16_t _window; 00219 00220 /** 00221 * Scan timeout between 0x0001 and 0xFFFF in seconds; 0x0000 disables timeout. 00222 */ 00223 uint16_t _timeout; 00224 00225 /** 00226 * Obtain the peer device's advertising data and (if possible) scanResponse. 00227 */ 00228 bool _activeScanning; 00229 00230 private: 00231 /* Disallow copy constructor. */ 00232 GapScanningParams(const GapScanningParams &); 00233 GapScanningParams& operator =(const GapScanningParams &in); 00234 }; 00235 00236 /** 00237 * @} 00238 * @} 00239 */ 00240 00241 #endif /* ifndef MBED_GAP_SCANNING_PARAMS_H__ */
Generated on Sun Jul 17 2022 08:25:23 by
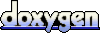