
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
GapAdvertisingParams.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_GAP_ADVERTISING_PARAMS_H__ 00018 #define MBED_GAP_ADVERTISING_PARAMS_H__ 00019 00020 /** 00021 * @addtogroup ble 00022 * @{ 00023 * @addtogroup gap 00024 * @{ 00025 */ 00026 00027 /** 00028 * Parameters defining the advertising process. 00029 * 00030 * Advertising parameters are a triplet of three value: 00031 * - The Advertising mode modeled after AdvertisingType_t. It defines 00032 * if the device is connectable and scannable. This value can be set at 00033 * construction time, updated with setAdvertisingType() and queried by 00034 * getAdvertisingType(). 00035 * - Time interval between advertisement. It can be set at construction time, 00036 * updated by setInterval() and obtained from getInterval(). 00037 * - Duration of the advertising process. As others, it can be set at 00038 * construction time, modified by setTimeout() and retrieved by getTimeout(). 00039 */ 00040 class GapAdvertisingParams { 00041 public: 00042 00043 /** 00044 * Minimum Advertising interval for connectable undirected and connectable 00045 * directed events in 625us units. 00046 * 00047 * @note Equal to 20 ms. 00048 */ 00049 static const unsigned GAP_ADV_PARAMS_INTERVAL_MIN = 0x0020; 00050 00051 /** 00052 * Minimum Advertising interval for scannable and nonconnectable 00053 * undirected events in 625us units. 00054 * 00055 * @note Equal to 100ms. 00056 */ 00057 static const unsigned GAP_ADV_PARAMS_INTERVAL_MIN_NONCON = 0x00A0; 00058 00059 /** 00060 * Maximum Advertising interval in 625us units. 00061 * 00062 * @note Equal to 10.24s. 00063 */ 00064 static const unsigned GAP_ADV_PARAMS_INTERVAL_MAX = 0x4000; 00065 00066 /** 00067 * Maximum advertising timeout allowed; in seconds. 00068 */ 00069 static const unsigned GAP_ADV_PARAMS_TIMEOUT_MAX = 0x3FFF; 00070 00071 /** 00072 * Encapsulates the peripheral advertising modes. 00073 * 00074 * It determine how the device appears to other scanner and peripheral 00075 * devices in the scanning range. 00076 */ 00077 enum AdvertisingType_t { 00078 /** 00079 * Device is connectable, scannable and doesn't expect connection from a 00080 * specific peer. 00081 * 00082 * @see Vol 3, Part C, Section 9.3.4 and Vol 6, Part B, Section 2.3.1.1. 00083 */ 00084 ADV_CONNECTABLE_UNDIRECTED, 00085 00086 /** 00087 * Device is connectable and expects connection from a specific peer. 00088 * 00089 * @see Vol 3, Part C, Section 9.3.3 and Vol 6, Part B, Section 2.3.1.2. 00090 */ 00091 ADV_CONNECTABLE_DIRECTED, 00092 00093 /** 00094 * Device is scannable but not connectable. 00095 * 00096 * @see Vol 6, Part B, Section 2.3.1.4. 00097 */ 00098 ADV_SCANNABLE_UNDIRECTED, 00099 00100 /** 00101 * Device is not connectable and not scannable. 00102 * 00103 * @see Vol 3, Part C, Section 9.3.2 and Vol 6, Part B, Section 2.3.1.3. 00104 */ 00105 ADV_NON_CONNECTABLE_UNDIRECTED 00106 }; 00107 00108 /** 00109 * Alias for GapAdvertisingParams::AdvertisingType_t. 00110 * 00111 * @deprecated Future releases will drop this type alias. 00112 */ 00113 typedef enum AdvertisingType_t AdvertisingType; 00114 00115 public: 00116 /** 00117 * Construct an instance of GapAdvertisingParams. 00118 * 00119 * @param[in] advType Type of advertising. 00120 * @param[in] interval Time interval between two advertisement in units of 00121 * 0.625ms. 00122 * @param[in] timeout Duration in seconds of the advertising process. A 00123 * value of 0 indicate that there is no timeout of the advertising process. 00124 * 00125 * @note If value in input are out of range, they will be normalized. 00126 */ 00127 GapAdvertisingParams( 00128 AdvertisingType_t advType = ADV_CONNECTABLE_UNDIRECTED, 00129 uint16_t interval = GAP_ADV_PARAMS_INTERVAL_MIN_NONCON, 00130 uint16_t timeout = 0 00131 ) : 00132 _advType(advType), 00133 _interval(interval), 00134 _timeout(timeout) 00135 { 00136 /* Interval checks. */ 00137 if (_advType == ADV_CONNECTABLE_DIRECTED) { 00138 /* Interval must be 0 in directed connectable mode. */ 00139 _interval = 0; 00140 } else if (_advType == ADV_NON_CONNECTABLE_UNDIRECTED) { 00141 /* Min interval is slightly larger than in other modes. */ 00142 if (_interval < GAP_ADV_PARAMS_INTERVAL_MIN_NONCON) { 00143 _interval = GAP_ADV_PARAMS_INTERVAL_MIN_NONCON; 00144 } 00145 if (_interval > GAP_ADV_PARAMS_INTERVAL_MAX) { 00146 _interval = GAP_ADV_PARAMS_INTERVAL_MAX; 00147 } 00148 } else { 00149 /* Stay within interval limits. */ 00150 if (_interval < GAP_ADV_PARAMS_INTERVAL_MIN) { 00151 _interval = GAP_ADV_PARAMS_INTERVAL_MIN; 00152 } 00153 if (_interval > GAP_ADV_PARAMS_INTERVAL_MAX) { 00154 _interval = GAP_ADV_PARAMS_INTERVAL_MAX; 00155 } 00156 } 00157 00158 /* Timeout checks. */ 00159 if (timeout) { 00160 /* Stay within timeout limits. */ 00161 if (_timeout > GAP_ADV_PARAMS_TIMEOUT_MAX) { 00162 _timeout = GAP_ADV_PARAMS_TIMEOUT_MAX; 00163 } 00164 } 00165 } 00166 00167 /** 00168 * Number of microseconds in 0.625 milliseconds. 00169 */ 00170 static const uint16_t UNIT_0_625_MS = 625; 00171 00172 /** 00173 * Convert milliseconds to units of 0.625ms. 00174 * 00175 * @param[in] durationInMillis Number of milliseconds to convert. 00176 * 00177 * @return The value of @p durationInMillis in units of 0.625ms. 00178 */ 00179 static uint16_t MSEC_TO_ADVERTISEMENT_DURATION_UNITS(uint32_t durationInMillis) 00180 { 00181 return (durationInMillis * 1000) / UNIT_0_625_MS; 00182 } 00183 00184 /** 00185 * Convert units of 0.625ms to milliseconds. 00186 * 00187 * @param[in] gapUnits The number of units of 0.625ms to convert. 00188 * 00189 * @return The value of @p gapUnits in milliseconds. 00190 */ 00191 static uint16_t ADVERTISEMENT_DURATION_UNITS_TO_MS(uint16_t gapUnits) 00192 { 00193 return (gapUnits * UNIT_0_625_MS) / 1000; 00194 } 00195 00196 /** 00197 * Get the advertising type. 00198 * 00199 * @return The advertising type. 00200 */ 00201 AdvertisingType_t getAdvertisingType(void) const 00202 { 00203 return _advType; 00204 } 00205 00206 /** 00207 * Get the advertising interval in milliseconds. 00208 * 00209 * @return The advertisement interval (in milliseconds). 00210 */ 00211 uint16_t getInterval(void) const 00212 { 00213 return ADVERTISEMENT_DURATION_UNITS_TO_MS(_interval); 00214 } 00215 00216 /** 00217 * Get the advertisement interval in units of 0.625ms. 00218 * 00219 * @return The advertisement interval in advertisement duration units 00220 * (0.625ms units). 00221 */ 00222 uint16_t getIntervalInADVUnits(void) const 00223 { 00224 return _interval; 00225 } 00226 00227 /** 00228 * Get the advertising timeout. 00229 * 00230 * @return The advertising timeout (in seconds). 00231 */ 00232 uint16_t getTimeout(void) const 00233 { 00234 return _timeout; 00235 } 00236 00237 /** 00238 * Update the advertising type. 00239 * 00240 * @param[in] newAdvType The new advertising type. 00241 */ 00242 void setAdvertisingType(AdvertisingType_t newAdvType) 00243 { 00244 _advType = newAdvType; 00245 } 00246 00247 /** 00248 * Update the advertising interval in milliseconds. 00249 * 00250 * @param[in] newInterval The new advertising interval in milliseconds. 00251 */ 00252 void setInterval(uint16_t newInterval) 00253 { 00254 _interval = MSEC_TO_ADVERTISEMENT_DURATION_UNITS(newInterval); 00255 } 00256 00257 /** 00258 * Update the advertising timeout. 00259 * 00260 * @param[in] newTimeout The new advertising timeout (in seconds). 00261 * 00262 * @note 0 is a special value meaning the advertising process never ends. 00263 */ 00264 void setTimeout(uint16_t newTimeout) 00265 { 00266 _timeout = newTimeout; 00267 } 00268 00269 private: 00270 /** 00271 * The advertising type. 00272 */ 00273 AdvertisingType_t _advType; 00274 00275 /** 00276 * The advertising interval in ADV duration units (in other words, 0.625ms). 00277 */ 00278 uint16_t _interval; 00279 00280 /** 00281 * The advertising timeout in seconds. 00282 */ 00283 uint16_t _timeout; 00284 }; 00285 00286 /** 00287 * @} 00288 * @} 00289 */ 00290 00291 #endif /* ifndef MBED_GAP_ADVERTISING_PARAMS_H__ */
Generated on Sun Jul 17 2022 08:25:23 by
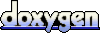