
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
FileSystemLike.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_FILESYSTEMLIKE_H 00017 #define MBED_FILESYSTEMLIKE_H 00018 00019 #include "platform/platform.h" 00020 00021 #include "platform/FileSystemHandle.h" 00022 #include "platform/FileHandle.h" 00023 #include "platform/DirHandle.h" 00024 #include "platform/NonCopyable.h" 00025 00026 namespace mbed { 00027 /** \addtogroup platform */ 00028 /** @{*/ 00029 /** 00030 * \defgroup platform_FileSystemLike FileSystemLike functions 00031 * @{ 00032 */ 00033 00034 00035 /** A filesystem-like object is one that can be used to open file-like 00036 * objects though it by fopen("/name/filename", mode) 00037 * 00038 * Implementations must define at least open (the default definitions 00039 * of the rest of the functions just return error values). 00040 * 00041 * @note Synchronization level: Set by subclass 00042 */ 00043 class FileSystemLike : public FileSystemHandle, public FileBase, private NonCopyable<FileSystemLike> { 00044 public: 00045 /** FileSystemLike lifetime 00046 */ 00047 FileSystemLike(const char *name = NULL) : FileBase(name, FileSystemPathType) {} 00048 virtual ~FileSystemLike() {} 00049 00050 // Inherited functions with name conflicts 00051 using FileSystemHandle::open; 00052 00053 /** Open a file on the filesystem 00054 * 00055 * @param path The name of the file to open 00056 * @param flags The flags to open the file in, one of O_RDONLY, O_WRONLY, O_RDWR, 00057 * bitwise or'd with one of O_CREAT, O_TRUNC, O_APPEND 00058 * @return A file handle on success, NULL on failure 00059 * @deprecated Replaced by `int open(FileHandle **, ...)` for propagating error codes 00060 */ 00061 MBED_DEPRECATED_SINCE("mbed-os-5.5", 00062 "Replaced by `int open(FileHandle **, ...)` for propagating error codes") 00063 FileHandle *open(const char *path, int flags) 00064 { 00065 FileHandle *file; 00066 int err = open(&file, path, flags); 00067 return err ? NULL : file; 00068 } 00069 00070 /** Open a directory on the filesystem 00071 * 00072 * @param path Name of the directory to open 00073 * @return A directory handle on success, NULL on failure 00074 * @deprecated Replaced by `int open(DirHandle **, ...)` for propagating error codes 00075 */ 00076 MBED_DEPRECATED_SINCE("mbed-os-5.5", 00077 "Replaced by `int open(DirHandle **, ...)` for propagating error codes") 00078 DirHandle *opendir(const char *path) 00079 { 00080 DirHandle *dir; 00081 int err = open(&dir, path); 00082 return err ? NULL : dir; 00083 } 00084 }; 00085 00086 /**@}*/ 00087 00088 /**@}*/ 00089 00090 } // namespace mbed 00091 00092 #endif
Generated on Sun Jul 17 2022 08:25:23 by
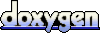