
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
rpc.h
00001 /* 00002 * Copyright (c) 2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef __UVISOR_API_RPC_H__ 00018 #define __UVISOR_API_RPC_H__ 00019 00020 #include "api/inc/rpc_exports.h" 00021 #include "api/inc/uvisor_exports.h" 00022 #include <stdint.h> 00023 #include <stddef.h> 00024 00025 00026 /** Wait for incoming RPC. 00027 * 00028 * @param fn_ptr_array an array of RPC function targets that this call to 00029 * `rpc_fncall_waitfor` should handle RPC to 00030 * @param fn_count the number of function targets in this array 00031 * @param box_id_caller[out] a memory location to store the box ID of the 00032 * calling box (the source box of the RPC). This is 00033 * set before the RPC is dispatched, so that the RPC 00034 * target function can read from this location to 00035 * determine the calling box ID. Optional. 00036 * @param timeout_ms specifies how long to wait (in ms) for an incoming 00037 * RPC message before returning 00038 */ 00039 UVISOR_EXTERN int rpc_fncall_waitfor(const TFN_Ptr fn_ptr_array[], size_t fn_count, int * box_id_caller, uint32_t timeout_ms); 00040 00041 /** Wait for an outgoing RPC to finish. 00042 * 00043 * Wait for the result of a previously started asynchronous RPC. After this 00044 * call, ret will contain the return value of the RPC. The return value of this 00045 * function may indicate that there was an error or a timeout with non-zero. 00046 * 00047 * @param result[in] The token to wait on for the result of an asynchronous RPC 00048 * @param timeout_ms[in] How long to wait (in ms) for the asynchronous RPC 00049 * message to finish before returning 00050 * @param ret[out] The return value resulting from the finished RPC to 00051 * the target function 00052 * @returns Non-zero on error or timeout, zero on successful wait 00053 */ 00054 UVISOR_EXTERN int rpc_fncall_wait(uvisor_rpc_result_t result, uint32_t timeout_ms, uint32_t * ret); 00055 00056 #endif /* __UVISOR_API_RPC_H__ */
Generated on Sun Jul 17 2022 08:25:29 by
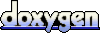