
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
nsdynmemLIB_stub.c
00001 /* 00002 * Copyright (c) 2014-2015 ARM Limited. All rights reserved. 00003 */ 00004 #include "nsdynmemLIB_stub.h" 00005 #include <stdint.h> 00006 #include <string.h> 00007 #include <nsdynmemLIB.h> 00008 #include "platform/arm_hal_interrupt.h" 00009 #ifdef STANDARD_MALLOC 00010 #include <stdlib.h> 00011 #endif 00012 00013 nsdynmemlib_stub_data_t nsdynmemlib_stub; 00014 00015 void ns_dyn_mem_init(uint8_t *heap, uint16_t h_size, void (*passed_fptr)(heap_fail_t), mem_stat_t *info_ptr) 00016 { 00017 } 00018 00019 void *ns_dyn_mem_alloc(int16_t alloc_size) 00020 { 00021 if (nsdynmemlib_stub.returnCounter > 0) 00022 { 00023 nsdynmemlib_stub.returnCounter--; 00024 return malloc(alloc_size); 00025 } 00026 else 00027 { 00028 return(nsdynmemlib_stub.expectedPointer); 00029 } 00030 } 00031 00032 void *ns_dyn_mem_temporary_alloc(int16_t alloc_size) 00033 { 00034 if (nsdynmemlib_stub.returnCounter > 0) 00035 { 00036 nsdynmemlib_stub.returnCounter--; 00037 return malloc(alloc_size); 00038 } 00039 else 00040 { 00041 return(nsdynmemlib_stub.expectedPointer); 00042 } 00043 } 00044 00045 void ns_dyn_mem_free(void *block) 00046 { 00047 free(block); 00048 }
Generated on Sun Jul 17 2022 08:25:29 by
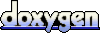