
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
mbed_trace_stub.c
00001 /* 00002 * Copyright (c) 2014-2017 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include <stdio.h> 00017 #include <string.h> 00018 #include <stdarg.h> 00019 #include <stdlib.h> 00020 00021 #ifndef YOTTA_CFG_MBED_TRACE 00022 #define YOTTA_CFG_MBED_TRACE 1 00023 #define YOTTA_CFG_MBED_TRACE_FEA_IPV6 1 00024 #endif 00025 00026 #include "mbed-trace/mbed_trace.h" 00027 #if YOTTA_CFG_MBED_TRACE_FEA_IPV6 == 1 00028 #include "mbed-client-libservice/ip6string.h" 00029 #include "mbed-client-libservice/common_functions.h" 00030 #endif 00031 00032 00033 int mbed_trace_init(void) 00034 { 00035 return 0; 00036 } 00037 void mbed_trace_free(void) 00038 { 00039 } 00040 00041 void mbed_trace_buffer_sizes(int lineLength, int tmpLength) 00042 { 00043 } 00044 00045 void mbed_trace_config_set(uint8_t config) 00046 { 00047 } 00048 00049 uint8_t mbed_trace_config_get(void) 00050 { 00051 return 0; 00052 } 00053 00054 void mbed_trace_prefix_function_set(char *(*pref_f)(size_t)) 00055 { 00056 } 00057 00058 void mbed_trace_suffix_function_set(char *(*suffix_f)(void)) 00059 { 00060 } 00061 00062 void mbed_trace_print_function_set(void (*printf)(const char *)) 00063 { 00064 } 00065 00066 void mbed_trace_cmdprint_function_set(void (*printf)(const char *)) 00067 { 00068 } 00069 00070 void mbed_trace_exclude_filters_set(char *filters) 00071 { 00072 00073 } 00074 00075 const char *mbed_trace_exclude_filters_get(void) 00076 { 00077 return NULL; 00078 } 00079 00080 const char *mbed_trace_include_filters_get(void) 00081 { 00082 return NULL; 00083 } 00084 00085 void mbed_trace_include_filters_set(char *filters) 00086 { 00087 00088 } 00089 00090 void mbed_tracef(uint8_t dlevel, const char *grp, const char *fmt, ...) 00091 { 00092 } 00093 00094 void mbed_vtracef(uint8_t dlevel, const char *grp, const char *fmt, va_list ap) 00095 { 00096 } 00097 00098 const char *mbed_trace_last(void) 00099 { 00100 return NULL; 00101 } 00102 00103 /* Helping functions */ 00104 #if YOTTA_CFG_MBED_TRACE_FEA_IPV6 == 1 00105 char *mbed_trace_ipv6(const void *addr_ptr) 00106 { 00107 return NULL; 00108 } 00109 00110 char *mbed_trace_ipv6_prefix(const uint8_t *prefix, uint8_t prefix_len) 00111 { 00112 return NULL; 00113 } 00114 #endif //YOTTA_CFG_MBED_TRACE_FEA_IPV6 00115 00116 char *mbed_trace_array(const uint8_t *buf, uint16_t len) 00117 { 00118 return NULL; 00119 }
Generated on Sun Jul 17 2022 08:25:27 by
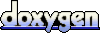