
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
DeepSleepLock.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_DEEPSLEEPLOCK_H 00017 #define MBED_DEEPSLEEPLOCK_H 00018 00019 #include <limits.h> 00020 #include "platform/mbed_sleep.h" 00021 #include "platform/mbed_critical.h" 00022 00023 namespace mbed { 00024 00025 /** \addtogroup platform */ 00026 /** @{*/ 00027 /** 00028 * \defgroup platform_DeepSleepLock DeepSleepLock functions 00029 * @{ 00030 */ 00031 00032 /** RAII object for disabling, then restoring the deep sleep mode 00033 * Usage: 00034 * @code 00035 * 00036 * void f() { 00037 * // some code here 00038 * { 00039 * DeepSleepLock lock; 00040 * // Code in this block will run with the deep sleep mode locked 00041 * } 00042 * // deep sleep mode will be restored to their previous state 00043 * } 00044 * @endcode 00045 */ 00046 class DeepSleepLock { 00047 private: 00048 uint16_t _lock_count; 00049 00050 public: 00051 DeepSleepLock(): _lock_count(1) 00052 { 00053 sleep_manager_lock_deep_sleep(); 00054 } 00055 00056 ~DeepSleepLock() 00057 { 00058 if (_lock_count) { 00059 sleep_manager_unlock_deep_sleep(); 00060 } 00061 } 00062 00063 /** Mark the start of a locked deep sleep section 00064 */ 00065 void lock() 00066 { 00067 uint16_t count = core_util_atomic_incr_u16(&_lock_count, 1); 00068 if (1 == count) { 00069 sleep_manager_lock_deep_sleep(); 00070 } 00071 if (0 == count) { 00072 error("DeepSleepLock overflow (> USHRT_MAX)"); 00073 } 00074 } 00075 00076 /** Mark the end of a locked deep sleep section 00077 */ 00078 void unlock() 00079 { 00080 uint16_t count = core_util_atomic_decr_u16(&_lock_count, 1); 00081 if (count == 0) { 00082 sleep_manager_unlock_deep_sleep(); 00083 } 00084 if (count == USHRT_MAX) { 00085 core_util_critical_section_exit(); 00086 error("DeepSleepLock underflow (< 0)"); 00087 } 00088 } 00089 }; 00090 00091 /**@}*/ 00092 00093 /**@}*/ 00094 00095 00096 } 00097 00098 #endif
Generated on Sun Jul 17 2022 08:25:21 by
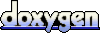