
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ChainingBlockDevice.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #ifndef MBED_CHAINING_BLOCK_DEVICE_H 00023 #define MBED_CHAINING_BLOCK_DEVICE_H 00024 00025 #include "BlockDevice.h" 00026 #include "mbed.h" 00027 00028 00029 /** Block device for chaining multiple block devices 00030 * with the similar block sizes at sequential addresses 00031 * 00032 * @code 00033 * #include "mbed.h" 00034 * #include "HeapBlockDevice.h" 00035 * #include "ChainingBlockDevice.h" 00036 * 00037 * // Create two smaller block devices with 00038 * // 64 and 32 blocks of size 512 bytes 00039 * HeapBlockDevice mem1(64*512, 512); 00040 * HeapBlockDevice mem2(32*512, 512); 00041 * 00042 * // Create a block device backed by mem1 and mem2 00043 * // contains 96 blocks of size 512 bytes 00044 * BlockDevice *bds[] = {&mem1, &mem2}; 00045 * ChainingBlockDevice chainmem(bds); 00046 * @endcode 00047 */ 00048 class ChainingBlockDevice : public BlockDevice 00049 { 00050 public: 00051 /** Lifetime of the memory block device 00052 * 00053 * @param bds Array of block devices to chain with sequential block addresses 00054 * @param bd_count Number of block devices to chain 00055 * @note All block devices must have the same block size 00056 */ 00057 ChainingBlockDevice(BlockDevice **bds, size_t bd_count); 00058 00059 /** Lifetime of the memory block device 00060 * 00061 * @param bds Array of block devices to chain with sequential block addresses 00062 * @note All block devices must have the same block size 00063 */ 00064 template <size_t Size> 00065 ChainingBlockDevice(BlockDevice *(&bds)[Size]) 00066 : _bds(bds), _bd_count(sizeof(bds) / sizeof(bds[0])) 00067 , _read_size(0), _program_size(0), _erase_size(0), _size(0) 00068 { 00069 } 00070 00071 /** Lifetime of the memory block device 00072 * 00073 */ 00074 virtual ~ChainingBlockDevice() {} 00075 00076 /** Initialize a block device 00077 * 00078 * @return 0 on success or a negative error code on failure 00079 */ 00080 virtual int init(); 00081 00082 /** Deinitialize a block device 00083 * 00084 * @return 0 on success or a negative error code on failure 00085 */ 00086 virtual int deinit(); 00087 00088 /** Read blocks from a block device 00089 * 00090 * @param buffer Buffer to write blocks to 00091 * @param addr Address of block to begin reading from 00092 * @param size Size to read in bytes, must be a multiple of read block size 00093 * @return 0 on success, negative error code on failure 00094 */ 00095 virtual int read(void *buffer, bd_addr_t addr, bd_size_t size); 00096 00097 /** Program blocks to a block device 00098 * 00099 * The blocks must have been erased prior to being programmed 00100 * 00101 * @param buffer Buffer of data to write to blocks 00102 * @param addr Address of block to begin writing to 00103 * @param size Size to write in bytes, must be a multiple of program block size 00104 * @return 0 on success, negative error code on failure 00105 */ 00106 virtual int program(const void *buffer, bd_addr_t addr, bd_size_t size); 00107 00108 /** Erase blocks on a block device 00109 * 00110 * The state of an erased block is undefined until it has been programmed 00111 * 00112 * @param addr Address of block to begin erasing 00113 * @param size Size to erase in bytes, must be a multiple of erase block size 00114 * @return 0 on success, negative error code on failure 00115 */ 00116 virtual int erase(bd_addr_t addr, bd_size_t size); 00117 00118 /** Get the size of a readable block 00119 * 00120 * @return Size of a readable block in bytes 00121 */ 00122 virtual bd_size_t get_read_size() const; 00123 00124 /** Get the size of a programable block 00125 * 00126 * @return Size of a programable block in bytes 00127 * @note Must be a multiple of the read size 00128 */ 00129 virtual bd_size_t get_program_size() const; 00130 00131 /** Get the size of a eraseable block 00132 * 00133 * @return Size of a eraseable block in bytes 00134 * @note Must be a multiple of the program size 00135 */ 00136 virtual bd_size_t get_erase_size() const; 00137 00138 /** Get the total size of the underlying device 00139 * 00140 * @return Size of the underlying device in bytes 00141 */ 00142 virtual bd_size_t size() const; 00143 00144 protected: 00145 BlockDevice **_bds; 00146 size_t _bd_count; 00147 bd_size_t _read_size; 00148 bd_size_t _program_size; 00149 bd_size_t _erase_size; 00150 bd_size_t _size; 00151 }; 00152 00153 00154 #endif
Generated on Sun Jul 17 2022 08:25:21 by
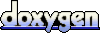