
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
BusOut.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_BUSOUT_H 00017 #define MBED_BUSOUT_H 00018 00019 #include "drivers/DigitalOut.h" 00020 #include "platform/PlatformMutex.h" 00021 #include "platform/NonCopyable.h" 00022 00023 namespace mbed { 00024 /** \addtogroup drivers */ 00025 00026 /** A digital output bus, used for setting the state of a collection of pins 00027 * @ingroup drivers 00028 */ 00029 class BusOut : private NonCopyable<BusOut> { 00030 00031 public: 00032 00033 /** Create an BusOut, connected to the specified pins 00034 * 00035 * @param p0 DigitalOut pin to connect to bus bit 00036 * @param p1 DigitalOut pin to connect to bus bit 00037 * @param p2 DigitalOut pin to connect to bus bit 00038 * @param p3 DigitalOut pin to connect to bus bit 00039 * @param p4 DigitalOut pin to connect to bus bit 00040 * @param p5 DigitalOut pin to connect to bus bit 00041 * @param p6 DigitalOut pin to connect to bus bit 00042 * @param p7 DigitalOut pin to connect to bus bit 00043 * @param p8 DigitalOut pin to connect to bus bit 00044 * @param p9 DigitalOut pin to connect to bus bit 00045 * @param p10 DigitalOut pin to connect to bus bit 00046 * @param p11 DigitalOut pin to connect to bus bit 00047 * @param p12 DigitalOut pin to connect to bus bit 00048 * @param p13 DigitalOut pin to connect to bus bit 00049 * @param p14 DigitalOut pin to connect to bus bit 00050 * @param p15 DigitalOut pin to connect to bus bit 00051 * 00052 * @note Synchronization level: Thread safe 00053 * 00054 * @note 00055 * It is only required to specify as many pin variables as is required 00056 * for the bus; the rest will default to NC (not connected) 00057 */ 00058 BusOut(PinName p0, PinName p1 = NC, PinName p2 = NC, PinName p3 = NC, 00059 PinName p4 = NC, PinName p5 = NC, PinName p6 = NC, PinName p7 = NC, 00060 PinName p8 = NC, PinName p9 = NC, PinName p10 = NC, PinName p11 = NC, 00061 PinName p12 = NC, PinName p13 = NC, PinName p14 = NC, PinName p15 = NC); 00062 00063 /** Create an BusOut, connected to the specified pins 00064 * 00065 * @param pins An array of pins to connect to bus the bit 00066 */ 00067 BusOut(PinName pins[16]); 00068 00069 virtual ~BusOut(); 00070 00071 /** Write the value to the output bus 00072 * 00073 * @param value An integer specifying a bit to write for every corresponding DigitalOut pin 00074 */ 00075 void write(int value); 00076 00077 /** Read the value currently output on the bus 00078 * 00079 * @returns 00080 * An integer with each bit corresponding to associated DigitalOut pin setting 00081 */ 00082 int read(); 00083 00084 /** Binary mask of bus pins connected to actual pins (not NC pins) 00085 * If bus pin is in NC state make corresponding bit will be cleared (set to 0), else bit will be set to 1 00086 * 00087 * @returns 00088 * Binary mask of connected pins 00089 */ 00090 int mask() { 00091 // No lock needed since _nc_mask is not modified outside the constructor 00092 return _nc_mask; 00093 } 00094 00095 /** A shorthand for write() 00096 * \sa BusOut::write() 00097 */ 00098 BusOut& operator= (int v); 00099 BusOut& operator= (BusOut& rhs); 00100 00101 /** Access to particular bit in random-iterator fashion 00102 * @param index Bit Position 00103 */ 00104 DigitalOut& operator[] (int index); 00105 00106 /** A shorthand for read() 00107 * \sa BusOut::read() 00108 */ 00109 operator int(); 00110 00111 protected: 00112 virtual void lock(); 00113 virtual void unlock(); 00114 DigitalOut* _pin[16]; 00115 00116 /* Mask of bus's NC pins 00117 * If bit[n] is set to 1 - pin is connected 00118 * if bit[n] is cleared - pin is not connected (NC) 00119 */ 00120 int _nc_mask; 00121 00122 PlatformMutex _mutex; 00123 }; 00124 00125 } // namespace mbed 00126 00127 #endif
Generated on Sun Jul 17 2022 08:25:20 by
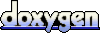