
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
APN_db.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ublox, ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /* ---------------------------------------------------------------- 00018 APN stands for Access Point Name, a setting on your modem or phone 00019 that identifies an external network your phone can access for data 00020 (e.g. 3G or 4G Internet service on your phone). 00021 00022 The APN settings can be forced when calling the join function. 00023 Below is a list of known APNs that us used if no apn config 00024 is forced. This list could be extended by other settings. 00025 00026 For further reading: 00027 wiki apn: http://en.wikipedia.org/wiki/Access_Point_Name 00028 wiki mcc/mnc: http://en.wikipedia.org/wiki/Mobile_country_code 00029 google: https://www.google.de/search?q=APN+list 00030 ---------------------------------------------------------------- */ 00031 00032 /** 00033 * Helper to generate the APN string 00034 */ 00035 #define _APN(apn,username,password) apn "\0" username "\0" password "\0" 00036 00037 /** 00038 * Helper to extract a field from the cfg string 00039 */ 00040 #define _APN_GET(cfg) \ 00041 *cfg ? cfg : NULL; \ 00042 cfg += strlen(cfg) + 1 00043 00044 /** 00045 * APN lookup struct 00046 */ 00047 typedef struct { 00048 const char* mccmnc; /**< mobile country code (MCC) and mobile network code MNC */ 00049 const char* cfg; /**< APN configuartion string, use _APN macro to generate */ 00050 } APN_t; 00051 00052 /** 00053 * Default APN settings used by many networks 00054 */ 00055 static const char* apndef = _APN("internet",,); 00056 00057 /** 00058 * List of special APNs for different network operators. 00059 * 00060 * No need to add default, "internet" will be used as a default if no entry matches. 00061 * The APN without username/password have to be listed first. 00062 */ 00063 00064 static const APN_t apnlut[] = { 00065 // MCC Country 00066 // { /* Operator */ "MCC-MNC[,MNC]" _APN(APN,USERNAME,PASSWORD) }, 00067 // MCC must be 3 digits 00068 // MNC must be either 2 or 3 digits 00069 // MCC must be separated by '-' from MNC, multiple MNC can be separated by ',' 00070 00071 // 232 Austria - AUT 00072 { /* T-Mobile */ "232-03", _APN("m2m.business",,) }, 00073 00074 // 460 China - CN 00075 { /* CN Mobile */"460-00", _APN("cmnet",,) 00076 _APN("cmwap",,) }, 00077 { /* Unicom */ "460-01", _APN("3gnet",,) 00078 _APN("uninet","uninet","uninet") }, 00079 00080 // 262 Germany - DE 00081 { /* T-Mobile */ "262-01", _APN("internet.t-mobile","t-mobile","tm") }, 00082 { /* T-Mobile */ "262-02,06", 00083 _APN("m2m.business",,) }, 00084 00085 // 222 Italy - IT 00086 { /* TIM */ "222-01", _APN("ibox.tim.it",,) }, 00087 { /* Vodafone */ "222-10", _APN("web.omnitel.it",,) }, 00088 { /* Wind */ "222-88", _APN("internet.wind.biz",,) }, 00089 00090 // 440 Japan - JP 00091 { /* Softbank */ "440-04,06,20,40,41,42,43,44,45,46,47,48,90,91,92,93,94,95" 00092 ",96,97,98" 00093 _APN("open.softbank.ne.jp","opensoftbank","ebMNuX1FIHg9d3DA") 00094 _APN("smile.world","dna1trop","so2t3k3m2a") }, 00095 { /* NTTDoCoMo */"440-09,10,11,12,13,14,15,16,17,18,19,21,22,23,24,25,26,27," 00096 "28,29,30,31,32,33,34,35,36,37,38,39,58,59,60,61,62,63," 00097 "64,65,66,67,68,69,87,99", 00098 _APN("bmobilewap",,) /*BMobile*/ 00099 _APN("mpr2.bizho.net","Mopera U",) /* DoCoMo */ 00100 _APN("bmobile.ne.jp","bmobile@wifi2","bmobile") /*BMobile*/ }, 00101 00102 // 204 Netherlands - NL 00103 { /* Vodafone */ "204-04", _APN("public4.m2minternet.com",,) }, 00104 00105 // 293 Slovenia - SI 00106 { /* Si.mobil */ "293-40", _APN("internet.simobil.si",,) }, 00107 { /* Tusmobil */ "293-70", _APN("internet.tusmobil.si",,) }, 00108 00109 // 240 Sweden SE 00110 { /* Telia */ "240-01", _APN("online.telia.se",,) }, 00111 { /* Telenor */ "240-06,08", 00112 _APN("services.telenor.se",,) }, 00113 { /* Tele2 */ "240-07", _APN("mobileinternet.tele2.se",,) }, 00114 00115 // 228 Switzerland - CH 00116 { /* Swisscom */ "228-01", _APN("gprs.swisscom.ch",,) }, 00117 { /* Orange */ "228-03", _APN("internet",,) /* contract */ 00118 _APN("click",,) /* pre-pay */ }, 00119 00120 // 234 United Kingdom - GB 00121 { /* O2 */ "234-02,10,11", 00122 _APN("mobile.o2.co.uk","faster","web") /* contract */ 00123 _APN("mobile.o2.co.uk","bypass","web") /* pre-pay */ 00124 _APN("payandgo.o2.co.uk","payandgo","payandgo") }, 00125 { /* Vodafone */ "234-15", _APN("internet","web","web") /* contract */ 00126 _APN("pp.vodafone.co.uk","wap","wap") /* pre-pay */ }, 00127 { /* Three */ "234-20", _APN("three.co.uk",,) }, 00128 { /* Jersey */ "234-50", _APN("jtm2m",,) /* as used on u-blox C030 U201 boards */ }, 00129 00130 // 310 United States of America - US 00131 { /* T-Mobile */ "310-026,260,490", 00132 _APN("epc.tmobile.com",,) 00133 _APN("fast.tmobile.com",,) /* LTE */ }, 00134 { /* AT&T */ "310-030,150,170,260,410,560,680", 00135 _APN("phone",,) 00136 _APN("wap.cingular","WAP@CINGULARGPRS.COM","CINGULAR1") 00137 _APN("isp.cingular","ISP@CINGULARGPRS.COM","CINGULAR1") }, 00138 00139 // 901 International - INT 00140 { /* Transatel */ "901-37", _APN("netgprs.com","tsl","tsl") }, 00141 }; 00142 00143 /** 00144 * Configuring APN by extraction from IMSI and matching the table. 00145 * 00146 * @param imsi strinf containing IMSI 00147 */ 00148 inline const char* apnconfig(const char* imsi) 00149 { 00150 const char* config = NULL; 00151 if (imsi && *imsi) { 00152 // many carriers use internet without username and password, os use this as default 00153 // now try to lookup the setting for our table 00154 for (size_t i = 0; i < sizeof(apnlut)/sizeof(*apnlut) && !config; i ++) { 00155 const char* p = apnlut[i].mccmnc; 00156 // check the MCC 00157 if ((0 == memcmp(imsi, p, 3))) { 00158 p += 3; 00159 // check all the MNC, MNC length can be 2 or 3 digits 00160 while (((p[0] == '-') || (p[0] == ',')) && 00161 (p[1] >= '0') && (p[1] <= '9') && 00162 (p[2] >= '0') && (p[2] <= '9') && !config) { 00163 int l = ((p[3] >= '0') && (p[3] <= '9')) ? 3 : 2; 00164 if (0 == memcmp(imsi+3,p+1,l)) 00165 config = apnlut[i].cfg; 00166 p += 1 + l; 00167 } 00168 } 00169 } 00170 } 00171 // use default if not found 00172 if (!config) { 00173 config = apndef; 00174 } 00175 return config; 00176 }
Generated on Sun Jul 17 2022 08:25:18 by
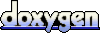