
3. An implementation of the system as a foreground0background
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 #define ERROR -1 00004 int touchSense1(void); 00005 int touchSense2(void); 00006 int getTouch(void); 00007 void hostError(void); 00008 void touchError(void); 00009 void runFunction(void); 00010 00011 DigitalOut myled1(LED1); 00012 DigitalOut myled2(LED3); 00013 00014 AnalogIn input1(p20); 00015 AnalogIn input2(p15); 00016 00017 DigitalIn charger1(p19); 00018 DigitalIn charger2(p16); 00019 00020 DigitalOut ground1(p18); 00021 DigitalOut ground2(p17); 00022 Serial pc(USBTX, USBRX); // tx, rx 00023 00024 int gInd = 0; 00025 int main() { 00026 pc.attach(runFunction); 00027 while(1) { 00028 if(gInd == 1) 00029 { 00030 pc.printf(" Reached back the main fun\n"); 00031 gInd =0; 00032 } 00033 wait(0.2); 00034 } 00035 } 00036 00037 void runFunction() { 00038 char A[1024],c='k'; 00039 int len, i, t_bit, h_bit; 00040 gInd = 1; 00041 int err_set ; 00042 pc.printf(" Entering the reading loop\n"); 00043 // if (pc.readable()) { 00044 i = 0; 00045 err_set = 0; 00046 while(c!='S' && pc.readable()) 00047 c = pc.getc(); 00048 00049 while( (c != 'E') && pc.readable()) 00050 { 00051 if(c != ' ') 00052 { 00053 //if((c == '0') || (c=='1')) 00054 A[i++] = c; 00055 if((i>1) && (c!='0') &&(c!='1')) 00056 { 00057 err_set = 1; 00058 hostError(); 00059 while(pc.readable()) //buffer out rest and return 00060 c=pc.getc(); 00061 return; 00062 } 00063 00064 } 00065 // pc.printf("READING %c \n",c); 00066 c = pc.getc(); 00067 } 00068 A[i] = c; 00069 A[i+1]='\0'; 00070 while(pc.readable()) //buffer out rest. 00071 c=pc.getc(); 00072 00073 00074 //pc.printf("DATA RECV : %s\n",A); 00075 len = strlen(A)-1; //to make it right offset 00076 if ((A[0]!='S') || (A[len] != 'E')) { 00077 hostError(); 00078 return; 00079 } 00080 i = 1; 00081 while (i<len) { 00082 t_bit = getTouch(); 00083 //pc.printf("\n MY TOKEN IS %c and touch is %d\n",A[i],t_bit); 00084 if (A[i] == '0') 00085 h_bit = 0; 00086 else if (A[i] == '1') 00087 h_bit = 1; 00088 00089 if (t_bit != h_bit) { 00090 touchError(); 00091 return; 00092 } 00093 i++; 00094 } 00095 pc.printf("MATCH\n"); 00096 //} 00097 return; 00098 } 00099 00100 int getTouch(void) { 00101 int sens; 00102 while (1) { 00103 00104 sens = 0; 00105 if (touchSense1()) { 00106 wait_ms(5); 00107 while(touchSense1()) 00108 { 00109 // pc.printf("In touchsense1\n"); 00110 sens = 1; 00111 wait_ms(100); 00112 } 00113 if(sens) 00114 return 0; 00115 } 00116 00117 if (touchSense2()) { 00118 wait_ms(5); 00119 while(touchSense2()) 00120 { 00121 // pc.printf("In touchsense2\n"); 00122 sens = 1; 00123 wait_ms(100); 00124 } 00125 if(sens) 00126 return 1; 00127 } 00128 } 00129 } 00130 00131 int touchSense1(void) { 00132 float sample; 00133 ground1 = 0; 00134 charger1.mode(PullUp); 00135 charger1.mode(PullNone); 00136 sample=input1.read(); 00137 if (sample < 0.3) { 00138 return 1; 00139 } else { 00140 return 0; 00141 } 00142 } 00143 00144 int touchSense2(void) { 00145 float sample; 00146 ground2 = 0; 00147 charger2.mode(PullUp); 00148 charger2.mode(PullNone); 00149 sample=input2.read(); 00150 if (sample < 0.3) { 00151 return 1; 00152 } else { 00153 return 0; 00154 } 00155 } 00156 00157 void hostError() { 00158 pc.printf("HOST ERROR"); 00159 } 00160 void touchError() { 00161 pc.printf("TOUCH ERROR"); 00162 }
Generated on Mon Jul 18 2022 15:27:21 by
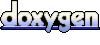