Dependents: SNMPAgent HTTPServer think_speak_a cyassl-client ... more
pbuf.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 00033 #ifndef __LWIP_PBUF_H__ 00034 #define __LWIP_PBUF_H__ 00035 00036 #include "lwip/opt.h" 00037 #include "lwip/err.h" 00038 00039 #ifdef __cplusplus 00040 extern "C" { 00041 #endif 00042 00043 /* Currently, the pbuf_custom code is only needed for one specific configuration 00044 * of IP_FRAG */ 00045 #define LWIP_SUPPORT_CUSTOM_PBUF (IP_FRAG && !IP_FRAG_USES_STATIC_BUF && !LWIP_NETIF_TX_SINGLE_PBUF) 00046 00047 #define PBUF_TRANSPORT_HLEN 20 00048 #define PBUF_IP_HLEN 20 00049 00050 typedef enum { 00051 PBUF_TRANSPORT, 00052 PBUF_IP, 00053 PBUF_LINK, 00054 PBUF_RAW 00055 } pbuf_layer; 00056 00057 typedef enum { 00058 PBUF_RAM, /* pbuf data is stored in RAM */ 00059 PBUF_ROM, /* pbuf data is stored in ROM */ 00060 PBUF_REF, /* pbuf comes from the pbuf pool */ 00061 PBUF_POOL /* pbuf payload refers to RAM */ 00062 } pbuf_type; 00063 00064 00065 /* indicates this packet's data should be immediately passed to the application */ 00066 #define PBUF_FLAG_PUSH 0x01U 00067 /* indicates this is a custom pbuf: pbuf_free and pbuf_header handle such a 00068 a pbuf differently */ 00069 #define PBUF_FLAG_IS_CUSTOM 0x02U 00070 /* indicates this pbuf is UDP multicast to be looped back */ 00071 #define PBUF_FLAG_MCASTLOOP 0x04U 00072 00073 struct pbuf { 00074 /* next pbuf in singly linked pbuf chain */ 00075 struct pbuf *next; 00076 00077 /* pointer to the actual data in the buffer */ 00078 void *payload; 00079 00080 /** 00081 * total length of this buffer and all next buffers in chain 00082 * belonging to the same packet. 00083 * 00084 * For non-queue packet chains this is the invariant: 00085 * p->tot_len == p->len + (p->next? p->next->tot_len: 0) 00086 */ 00087 u16_t tot_len; 00088 00089 /* length of this buffer */ 00090 u16_t len; 00091 00092 /* pbuf_type as u8_t instead of enum to save space */ 00093 u8_t /*pbuf_type*/ type; 00094 00095 /* misc flags */ 00096 u8_t flags; 00097 00098 /** 00099 * the reference count always equals the number of pointers 00100 * that refer to this pbuf. This can be pointers from an application, 00101 * the stack itself, or pbuf->next pointers from a chain. 00102 */ 00103 u16_t ref; 00104 }; 00105 00106 #if LWIP_SUPPORT_CUSTOM_PBUF 00107 /* Prototype for a function to free a custom pbuf */ 00108 typedef void (*pbuf_free_custom_fn)(struct pbuf *p); 00109 00110 /* A custom pbuf: like a pbuf, but following a function pointer to free it. */ 00111 struct pbuf_custom { 00112 /* The actual pbuf */ 00113 struct pbuf pbuf; 00114 /* This function is called when pbuf_free deallocates this pbuf(_custom) */ 00115 pbuf_free_custom_fn custom_free_function; 00116 }; 00117 #endif /* LWIP_SUPPORT_CUSTOM_PBUF */ 00118 00119 /* Initializes the pbuf module. This call is empty for now, but may not be in future. */ 00120 #define pbuf_init() 00121 00122 struct pbuf *pbuf_alloc(pbuf_layer l, u16_t length, pbuf_type type); 00123 #if LWIP_SUPPORT_CUSTOM_PBUF 00124 struct pbuf *pbuf_alloced_custom(pbuf_layer l, u16_t length, pbuf_type type, 00125 struct pbuf_custom *p, void *payload_mem, 00126 u16_t payload_mem_len); 00127 #endif /* LWIP_SUPPORT_CUSTOM_PBUF */ 00128 void pbuf_realloc(struct pbuf *p, u16_t size); 00129 u8_t pbuf_header(struct pbuf *p, s16_t header_size); 00130 void pbuf_ref(struct pbuf *p); 00131 u8_t pbuf_free(struct pbuf *p); 00132 u8_t pbuf_clen(struct pbuf *p); 00133 void pbuf_cat(struct pbuf *head, struct pbuf *tail); 00134 void pbuf_chain(struct pbuf *head, struct pbuf *tail); 00135 struct pbuf *pbuf_dechain(struct pbuf *p); 00136 err_t pbuf_copy(struct pbuf *p_to, struct pbuf *p_from); 00137 u16_t pbuf_copy_partial(struct pbuf *p, void *dataptr, u16_t len, u16_t offset); 00138 err_t pbuf_take(struct pbuf *buf, const void *dataptr, u16_t len); 00139 struct pbuf *pbuf_coalesce(struct pbuf *p, pbuf_layer layer); 00140 #if LWIP_CHECKSUM_ON_COPY 00141 err_t pbuf_fill_chksum(struct pbuf *p, u16_t start_offset, const void *dataptr, 00142 u16_t len, u16_t *chksum); 00143 #endif /* LWIP_CHECKSUM_ON_COPY */ 00144 00145 u8_t pbuf_get_at(struct pbuf* p, u16_t offset); 00146 u16_t pbuf_memcmp(struct pbuf* p, u16_t offset, const void* s2, u16_t n); 00147 u16_t pbuf_memfind(struct pbuf* p, const void* mem, u16_t mem_len, u16_t start_offset); 00148 u16_t pbuf_strstr(struct pbuf* p, const char* substr); 00149 00150 #ifdef __cplusplus 00151 } 00152 #endif 00153 00154 #endif /* __LWIP_PBUF_H__ */
Generated on Tue Jul 12 2022 21:45:26 by
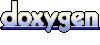