Dependents: SNMPAgent HTTPServer think_speak_a cyassl-client ... more
host.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef HOST_H 00025 #define HOST_H 00026 00027 #include "ipaddr.h" 00028 #include <string.h> 00029 00030 ///Host information container 00031 /** 00032 This class is a container for data relative to a connection: 00033 - IP Address 00034 - Port number 00035 - Host Name 00036 */ 00037 class Host 00038 { 00039 public: 00040 ///Initiliazes host with null values 00041 Host() : m_ip(0,0,0,0), m_port(0), m_name(NULL) 00042 { 00043 00044 } 00045 00046 ///Initializes host 00047 Host(const IpAddr& ip, const int& port, const char* name="" ) : m_ip(ip), m_port(port), m_name(NULL) 00048 { 00049 setName(name); 00050 } 00051 00052 ~Host() 00053 { 00054 if(m_name) 00055 { 00056 delete[] m_name; 00057 } 00058 } 00059 00060 ///Returns IP address 00061 const IpAddr& getIp() const 00062 { 00063 return m_ip; 00064 } 00065 00066 ///Returns port number 00067 const int& getPort() const 00068 { 00069 return m_port; 00070 } 00071 00072 ///Returns host name 00073 const char* getName() const 00074 { 00075 return m_name; 00076 } 00077 00078 ///Sets IP address 00079 void setIp(const IpAddr& ip) 00080 { 00081 m_ip = ip; 00082 } 00083 00084 ///Sets port number 00085 void setPort(int port) 00086 { 00087 m_port = port; 00088 } 00089 00090 ///Sets host name 00091 void setName(const char* name) 00092 { 00093 if(m_name) 00094 delete[] m_name; 00095 int len = strlen(name); 00096 if(len) 00097 { 00098 m_name = new char[len+1]; 00099 strcpy(m_name, name); 00100 } 00101 } 00102 00103 private: 00104 IpAddr m_ip; 00105 int m_port; 00106 char* m_name; 00107 }; 00108 00109 #endif
Generated on Tue Jul 12 2022 21:45:25 by
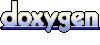