Dependents: SNMPAgent HTTPServer think_speak_a cyassl-client ... more
DNSRequest.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 /** \file 00025 DNS Request header file 00026 */ 00027 00028 #ifndef DNSREQUEST_H 00029 #define DNSREQUEST_H 00030 00031 #include "core/net.h" 00032 #include "core/ipaddr.h" 00033 #include "core/host.h" 00034 //Essentially it is a safe interface to NetDnsRequest 00035 00036 ///DNS Request error codes 00037 enum DNSRequestErr 00038 { 00039 __DNS_MIN = -0xFFFF, 00040 DNS_SETUP, ///<DNSRequest not properly configured 00041 DNS_IF, ///<Interface has problems, does not exist or is not initialized 00042 DNS_MEM, ///<Not enough mem 00043 DNS_INUSE, ///<Interface / Port is in use 00044 DNS_PROCESSING, ///<Request has not completed 00045 //... 00046 DNS_OK = 0 ///<Success 00047 }; 00048 00049 ///DNS Request Result Events 00050 enum DNSReply 00051 { 00052 DNS_PRTCL, 00053 DNS_NOTFOUND, ///Hostname is unknown 00054 DNS_ERROR, ///Problem with DNS Service 00055 //... 00056 DNS_FOUND, 00057 }; 00058 00059 class NetDnsRequest; 00060 enum NetDnsReply; 00061 00062 ///This is a simple DNS Request class 00063 /** 00064 This class exposes an API to deal with DNS Requests 00065 */ 00066 class DNSRequest 00067 { 00068 public: 00069 ///Creates a new request 00070 DNSRequest(); 00071 00072 ///Terminates and closes request 00073 ~DNSRequest(); 00074 00075 ///Resolves an hostname 00076 /** 00077 @param hostname : hostname to resolve 00078 */ 00079 DNSRequestErr resolve(const char* hostname); 00080 00081 ///Resolves an hostname 00082 /** 00083 @param host : hostname to resolve, the result will be stored in the IpAddr field of this object 00084 */ 00085 DNSRequestErr resolve(Host* pHost); 00086 00087 ///Setups callback 00088 /** 00089 The callback function will be called on result. 00090 @param pMethod : callback function 00091 */ 00092 void setOnReply( void (*pMethod)(DNSReply) ); 00093 00094 class CDummy; 00095 ///Setups callback 00096 /** 00097 The callback function will be called on result. 00098 @param pItem : instance of class on which to execute the callback method 00099 @param pMethod : callback method 00100 */ 00101 template<class T> 00102 void setOnReply( T* pItem, void (T::*pMethod)(DNSReply) ) 00103 { 00104 m_pCbItem = (CDummy*) pItem; 00105 m_pCbMeth = (void (CDummy::*)(DNSReply)) pMethod; 00106 } 00107 00108 ///Gets IP address once it has been resolved 00109 /** 00110 @param pIp : pointer to an IpAddr instance in which to store the resolved IP address 00111 */ 00112 DNSRequestErr getResult(IpAddr* pIp); 00113 00114 ///Closes DNS Request before completion 00115 DNSRequestErr close(); 00116 00117 protected: 00118 void onNetDnsReply(NetDnsReply r); 00119 DNSRequestErr checkInst(); 00120 00121 private: 00122 NetDnsRequest* m_pNetDnsRequest; 00123 00124 CDummy* m_pCbItem; 00125 void (CDummy::*m_pCbMeth)(DNSReply); 00126 00127 void (*m_pCb)(DNSReply); 00128 00129 }; 00130 00131 #endif
Generated on Tue Jul 12 2022 21:45:25 by
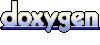