Dependents: SNMPAgent HTTPServer think_speak_a cyassl-client ... more
ppp_oe.h
00001 /***************************************************************************** 00002 * ppp_oe.h - PPP Over Ethernet implementation for lwIP. 00003 * 00004 * Copyright (c) 2006 by Marc Boucher, Services Informatiques (MBSI) inc. 00005 * 00006 * The authors hereby grant permission to use, copy, modify, distribute, 00007 * and license this software and its documentation for any purpose, provided 00008 * that existing copyright notices are retained in all copies and that this 00009 * notice and the following disclaimer are included verbatim in any 00010 * distributions. No written agreement, license, or royalty fee is required 00011 * for any of the authorized uses. 00012 * 00013 * THIS SOFTWARE IS PROVIDED BY THE CONTRIBUTORS *AS IS* AND ANY EXPRESS OR 00014 * IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES 00015 * OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00016 * IN NO EVENT SHALL THE CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00017 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 00018 * NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00019 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00020 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00021 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF 00022 * THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00023 * 00024 ****************************************************************************** 00025 * REVISION HISTORY 00026 * 00027 * 06-01-01 Marc Boucher <marc@mbsi.ca> 00028 * Ported to lwIP. 00029 *****************************************************************************/ 00030 00031 00032 00033 /* based on NetBSD: if_pppoe.c,v 1.64 2006/01/31 23:50:15 martin Exp */ 00034 00035 /*- 00036 * Copyright (c) 2002 The NetBSD Foundation, Inc. 00037 * All rights reserved. 00038 * 00039 * This code is derived from software contributed to The NetBSD Foundation 00040 * by Martin Husemann <martin@NetBSD.org>. 00041 * 00042 * Redistribution and use in source and binary forms, with or without 00043 * modification, are permitted provided that the following conditions 00044 * are met: 00045 * 1. Redistributions of source code must retain the above copyright 00046 * notice, this list of conditions and the following disclaimer. 00047 * 2. Redistributions in binary form must reproduce the above copyright 00048 * notice, this list of conditions and the following disclaimer in the 00049 * documentation and/or other materials provided with the distribution. 00050 * 3. All advertising materials mentioning features or use of this software 00051 * must display the following acknowledgement: 00052 * This product includes software developed by the NetBSD 00053 * Foundation, Inc. and its contributors. 00054 * 4. Neither the name of The NetBSD Foundation nor the names of its 00055 * contributors may be used to endorse or promote products derived 00056 * from this software without specific prior written permission. 00057 * 00058 * THIS SOFTWARE IS PROVIDED BY THE NETBSD FOUNDATION, INC. AND CONTRIBUTORS 00059 * ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED 00060 * TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR 00061 * PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE FOUNDATION OR CONTRIBUTORS 00062 * BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00063 * CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF 00064 * SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00065 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00066 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 00067 * ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00068 * POSSIBILITY OF SUCH DAMAGE. 00069 */ 00070 #ifndef PPP_OE_H 00071 #define PPP_OE_H 00072 00073 #include "lwip/opt.h" 00074 00075 #if PPPOE_SUPPORT > 0 00076 00077 #include "netif/etharp.h" 00078 00079 #ifdef PACK_STRUCT_USE_INCLUDES 00080 # include "arch/bpstruct.h" 00081 #endif 00082 PACK_STRUCT_BEGIN 00083 struct pppoehdr { 00084 PACK_STRUCT_FIELD(u8_t vertype); 00085 PACK_STRUCT_FIELD(u8_t code); 00086 PACK_STRUCT_FIELD(u16_t session); 00087 PACK_STRUCT_FIELD(u16_t plen); 00088 } PACK_STRUCT_STRUCT; 00089 PACK_STRUCT_END 00090 #ifdef PACK_STRUCT_USE_INCLUDES 00091 # include "arch/epstruct.h" 00092 #endif 00093 00094 #ifdef PACK_STRUCT_USE_INCLUDES 00095 # include "arch/bpstruct.h" 00096 #endif 00097 PACK_STRUCT_BEGIN 00098 struct pppoetag { 00099 PACK_STRUCT_FIELD(u16_t tag); 00100 PACK_STRUCT_FIELD(u16_t len); 00101 } PACK_STRUCT_STRUCT; 00102 PACK_STRUCT_END 00103 #ifdef PACK_STRUCT_USE_INCLUDES 00104 # include "arch/epstruct.h" 00105 #endif 00106 00107 00108 #define PPPOE_STATE_INITIAL 0 00109 #define PPPOE_STATE_PADI_SENT 1 00110 #define PPPOE_STATE_PADR_SENT 2 00111 #define PPPOE_STATE_SESSION 3 00112 #define PPPOE_STATE_CLOSING 4 00113 /* passive */ 00114 #define PPPOE_STATE_PADO_SENT 1 00115 00116 #define PPPOE_HEADERLEN sizeof(struct pppoehdr) 00117 #define PPPOE_VERTYPE 0x11 /* VER=1, TYPE = 1 */ 00118 00119 #define PPPOE_TAG_EOL 0x0000 /* end of list */ 00120 #define PPPOE_TAG_SNAME 0x0101 /* service name */ 00121 #define PPPOE_TAG_ACNAME 0x0102 /* access concentrator name */ 00122 #define PPPOE_TAG_HUNIQUE 0x0103 /* host unique */ 00123 #define PPPOE_TAG_ACCOOKIE 0x0104 /* AC cookie */ 00124 #define PPPOE_TAG_VENDOR 0x0105 /* vendor specific */ 00125 #define PPPOE_TAG_RELAYSID 0x0110 /* relay session id */ 00126 #define PPPOE_TAG_SNAME_ERR 0x0201 /* service name error */ 00127 #define PPPOE_TAG_ACSYS_ERR 0x0202 /* AC system error */ 00128 #define PPPOE_TAG_GENERIC_ERR 0x0203 /* gerneric error */ 00129 00130 #define PPPOE_CODE_PADI 0x09 /* Active Discovery Initiation */ 00131 #define PPPOE_CODE_PADO 0x07 /* Active Discovery Offer */ 00132 #define PPPOE_CODE_PADR 0x19 /* Active Discovery Request */ 00133 #define PPPOE_CODE_PADS 0x65 /* Active Discovery Session confirmation */ 00134 #define PPPOE_CODE_PADT 0xA7 /* Active Discovery Terminate */ 00135 00136 #ifndef ETHERMTU 00137 #define ETHERMTU 1500 00138 #endif 00139 00140 /* two byte PPP protocol discriminator, then IP data */ 00141 #define PPPOE_MAXMTU (ETHERMTU-PPPOE_HEADERLEN-2) 00142 00143 #ifndef PPPOE_MAX_AC_COOKIE_LEN 00144 #define PPPOE_MAX_AC_COOKIE_LEN 64 00145 #endif 00146 00147 struct pppoe_softc { 00148 struct pppoe_softc *next; 00149 struct netif *sc_ethif; /* ethernet interface we are using */ 00150 int sc_pd; /* ppp unit number */ 00151 void (*sc_linkStatusCB)(int pd, int up); 00152 00153 int sc_state; /* discovery phase or session connected */ 00154 struct eth_addr sc_dest; /* hardware address of concentrator */ 00155 u16_t sc_session; /* PPPoE session id */ 00156 00157 #ifdef PPPOE_TODO 00158 char *sc_service_name; /* if != NULL: requested name of service */ 00159 char *sc_concentrator_name; /* if != NULL: requested concentrator id */ 00160 #endif /* PPPOE_TODO */ 00161 u8_t sc_ac_cookie[PPPOE_MAX_AC_COOKIE_LEN]; /* content of AC cookie we must echo back */ 00162 size_t sc_ac_cookie_len; /* length of cookie data */ 00163 #ifdef PPPOE_SERVER 00164 u8_t *sc_hunique; /* content of host unique we must echo back */ 00165 size_t sc_hunique_len; /* length of host unique */ 00166 #endif 00167 int sc_padi_retried; /* number of PADI retries already done */ 00168 int sc_padr_retried; /* number of PADR retries already done */ 00169 }; 00170 00171 00172 #define pppoe_init() /* compatibility define, no initialization needed */ 00173 00174 err_t pppoe_create(struct netif *ethif, int pd, void (*linkStatusCB)(int pd, int up), struct pppoe_softc **scptr); 00175 err_t pppoe_destroy(struct netif *ifp); 00176 00177 int pppoe_connect(struct pppoe_softc *sc); 00178 void pppoe_disconnect(struct pppoe_softc *sc); 00179 00180 void pppoe_disc_input(struct netif *netif, struct pbuf *p); 00181 void pppoe_data_input(struct netif *netif, struct pbuf *p); 00182 00183 err_t pppoe_xmit(struct pppoe_softc *sc, struct pbuf *pb); 00184 00185 /** used in ppp.c */ 00186 #define PPPOE_HDRLEN (sizeof(struct eth_hdr) + PPPOE_HEADERLEN) 00187 00188 #endif /* PPPOE_SUPPORT */ 00189 00190 #endif /* PPP_OE_H */
Generated on Tue Jul 12 2022 21:45:26 by
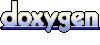