Dependents: SNMPAgent HTTPServer think_speak_a cyassl-client ... more
nettcpsocket.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef NETTCPSOCKET_H 00025 #define NETTCPSOCKET_H 00026 00027 #include "net.h" 00028 #include "host.h" 00029 00030 #include <queue> 00031 using std::queue; 00032 00033 //Implements a Berkeley-like socket if 00034 //Can be interfaced either to lwip or a Telit module 00035 00036 enum NetTcpSocketErr 00037 { 00038 __NETTCPSOCKET_MIN = -0xFFFF, 00039 NETTCPSOCKET_SETUP, //NetTcpSocket not properly configured 00040 NETTCPSOCKET_TIMEOUT, 00041 NETTCPSOCKET_IF, //If has problems 00042 NETTCPSOCKET_MEM, //Not enough mem 00043 NETTCPSOCKET_INUSE, //If/Port is in use 00044 NETTCPSOCKET_EMPTY, //Connections queue is empty 00045 NETTCPSOCKET_RST, // Connection was reset by remote host 00046 //... 00047 NETTCPSOCKET_OK = 0 00048 }; 00049 00050 enum NetTcpSocketEvent 00051 { 00052 NETTCPSOCKET_CONNECTED, //Connected to host, must call accept() if we were listening 00053 NETTCPSOCKET_ACCEPT, //Connected to client 00054 NETTCPSOCKET_READABLE, //Data in buf 00055 NETTCPSOCKET_WRITEABLE, //Can write data to buf 00056 NETTCPSOCKET_CONTIMEOUT, 00057 NETTCPSOCKET_CONRST, 00058 NETTCPSOCKET_CONABRT, 00059 NETTCPSOCKET_ERROR, 00060 NETTCPSOCKET_DISCONNECTED 00061 }; 00062 00063 00064 class NetTcpSocket 00065 { 00066 public: 00067 NetTcpSocket(); 00068 virtual ~NetTcpSocket(); //close() 00069 00070 virtual NetTcpSocketErr bind(const Host& me) = 0; 00071 virtual NetTcpSocketErr listen() = 0; 00072 virtual NetTcpSocketErr connect(const Host& host) = 0; 00073 virtual NetTcpSocketErr accept(Host* pClient, NetTcpSocket** ppNewNetTcpSocket) = 0; 00074 00075 virtual int /*if < 0 : NetTcpSocketErr*/ send(const char* buf, int len) = 0; 00076 virtual int /*if < 0 : NetTcpSocketErr*/ recv(char* buf, int len) = 0; 00077 00078 virtual NetTcpSocketErr close() = 0; 00079 00080 virtual NetTcpSocketErr poll() = 0; 00081 00082 class CDummy; 00083 //Callbacks 00084 template<class T> 00085 //Linker bug : Must be defined here :( 00086 void setOnEvent( T* pItem, void (T::*pMethod)(NetTcpSocketEvent) ) 00087 { 00088 m_pCbItem = (CDummy*) pItem; 00089 m_pCbMeth = (void (CDummy::*)(NetTcpSocketEvent)) pMethod; 00090 } 00091 00092 void resetOnEvent(); //Disable callback 00093 00094 protected: 00095 void queueEvent(NetTcpSocketEvent e); 00096 void discardEvents(); 00097 void flushEvents(); //to be called during polling 00098 00099 Host m_host; 00100 Host m_client; 00101 00102 friend class Net; 00103 int m_refs; 00104 00105 bool m_closed; 00106 bool m_removed; 00107 00108 private: 00109 //We do not want to execute user code in interrupt routines, so we queue events until the server is polled 00110 //If we port this to a multithreaded OS, we could avoid this (however some functions here are not thread-safe, so beware ;) ) 00111 void onEvent(NetTcpSocketEvent e); //To be called on poll 00112 CDummy* m_pCbItem; 00113 void (CDummy::*m_pCbMeth)(NetTcpSocketEvent); 00114 queue<NetTcpSocketEvent> m_events; 00115 00116 }; 00117 00118 #endif
Generated on Tue Jul 12 2022 21:45:26 by
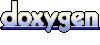