
sa
Embed:
(wiki syntax)
Show/hide line numbers
mfcc.h
00001 /* 00002 * Copyright (C) 2018 Arm Limited or its affiliates. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: Apache-2.0 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the License); you may 00007 * not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00014 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 */ 00018 00019 #ifndef __KWS_MFCC_H__ 00020 #define __KWS_MFCC_H__ 00021 00022 #include "arm_math.h" 00023 #include "string.h" 00024 00025 #define SAMP_FREQ 16000 00026 #define NUM_FBANK_BINS 40 00027 #define MEL_LOW_FREQ 20 00028 #define MEL_HIGH_FREQ 4000 00029 00030 #define M_2PI 6.283185307179586476925286766559005 00031 #define M_PI M_2PI/2 00032 00033 class MFCC{ 00034 private: 00035 int num_mfcc_features; 00036 int frame_len; 00037 int frame_len_padded; 00038 int mfcc_dec_bits; 00039 float * frame; 00040 float * buffer; 00041 float * mel_energies; 00042 float * window_func; 00043 int32_t * fbank_filter_first; 00044 int32_t * fbank_filter_last; 00045 float ** mel_fbank; 00046 float * dct_matrix; 00047 arm_rfft_fast_instance_f32 * rfft; 00048 float * create_dct_matrix(int32_t input_length, int32_t coefficient_count); 00049 float ** create_mel_fbank(); 00050 00051 static inline float InverseMelScale(float mel_freq) { 00052 return 700.0f * (expf (mel_freq / 1127.0f) - 1.0f); 00053 } 00054 00055 static inline float MelScale(float freq) { 00056 return 1127.0f * logf (1.0f + freq / 700.0f); 00057 } 00058 00059 public: 00060 MFCC(int num_mfcc_features, int frame_len, int mfcc_dec_bits); 00061 ~MFCC(); 00062 void mfcc_compute(const int16_t* data, q7_t* mfcc_out); 00063 }; 00064 00065 #endif
Generated on Sat Aug 13 2022 06:12:26 by
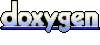