
My fully self designed first stable working Quadrocopter Software.
Embed:
(wiki syntax)
Show/hide line numbers
MPU6050.cpp
00001 #include "MPU6050.h" 00002 00003 MPU6050::MPU6050(PinName sda, PinName scl) : I2C_Sensor(sda, scl, MPU6050_I2C_ADDRESS) 00004 { 00005 // Turns on the MPU6050's gyro and initializes it 00006 // register datasheet: http://www.invensense.com/mems/gyro/documents/RM-MPU-6000A.pdf 00007 writeRegister(MPU6050_RA_PWR_MGMT_1, 0x01); // wake up from sleep and chooses Gyro X-Axis as Clock source (stadard sleeping and with inacurate clock is 0x40) 00008 writeRegister(MPU6050_RA_CONFIG, 0x03); 00009 /* 00010 last 3 Bits of |Accelerometer(Fs=1kHz) |Gyroscope 00011 MPU6050_RA_CONFIG|Bandwidth(Hz)|Delay(ms)|Bandwidth(Hz)|Delay(ms)|Fs(kHz) 00012 ------------------------------------------------------------------------- 00013 0 |260 |0 |256 |0.98 |8 00014 1 |184 |2.0 |188 |1.9 |1 00015 2 |94 |3.0 |98 |2.8 |1 00016 3 |44 |4.9 |42 |4.8 |1 00017 4 |21 |8.5 |20 |8.3 |1 00018 5 |10 |13.8 |10 |13.4 |1 00019 6 |5 |19.0 |5 |18.6 |1 00020 */ 00021 writeRegister(MPU6050_RA_GYRO_CONFIG, 0x18); // scales gyros range to +-2000dps 00022 writeRegister(MPU6050_RA_ACCEL_CONFIG, 0x00); // scales accelerometers range to +-2g 00023 } 00024 00025 void MPU6050::read() 00026 { 00027 readraw_gyro(); // read raw measurement data 00028 readraw_acc(); 00029 00030 for (int i = 0; i < 3; i++) 00031 data_gyro[i] = (raw_gyro[i] - offset_gyro[i])*0.07; // subtract offset from calibration and multiply unit factor to get degree per second (datasheet p.10) 00032 00033 for (int i = 0; i < 3; i++) 00034 data_acc[i] = raw_acc[i] - offset_acc[i]; // TODO: didn't care about units because IMU-algorithm just uses vector direction 00035 00036 // I have to swich coordinates on my board to match the ones of the other sensors (clear this part if you use the raw coordinates of the sensor) 00037 float tmp = 0; 00038 tmp = data_gyro[0]; 00039 data_gyro[0] = data_gyro[1]; 00040 data_gyro[1] = -tmp; 00041 data_gyro[2] = data_gyro[2]; 00042 tmp = data_acc[0]; 00043 data_acc[0] = data_acc[1]; 00044 data_acc[1] = -tmp; 00045 data_acc[2] = data_acc[2]; 00046 } 00047 00048 int MPU6050::readTemp() 00049 { 00050 char buffer[2]; // 8-Bit pieces of temperature data 00051 00052 readMultiRegister(MPU6050_RA_TEMP_OUT_H, buffer, 2); // read the sensors register for the temperature 00053 return (short) (buffer[0] << 8 | buffer[1]); 00054 } 00055 00056 void MPU6050::readraw_gyro() 00057 { 00058 char buffer[6]; // 8-Bit pieces of axis data 00059 00060 if(readMultiRegister(MPU6050_RA_GYRO_XOUT_H | (1 << 7), buffer, 6) != 0) return; // read axis registers using I2C // TODO: why?! | (1 << 7) 00061 00062 raw_gyro[0] = (short) (buffer[0] << 8 | buffer[1]); // join 8-Bit pieces to 16-bit short integers 00063 raw_gyro[1] = (short) (buffer[2] << 8 | buffer[3]); 00064 raw_gyro[2] = (short) (buffer[4] << 8 | buffer[5]); 00065 } 00066 00067 void MPU6050::readraw_acc() 00068 { 00069 char buffer[6]; // 8-Bit pieces of axis data 00070 00071 readMultiRegister(MPU6050_RA_ACCEL_XOUT_H | (1 << 7), buffer, 6); // read axis registers using I2C // TODO: why?! | (1 << 7) 00072 00073 raw_acc[0] = (short) (buffer[0] << 8 | buffer[1]); // join 8-Bit pieces to 16-bit short integers 00074 raw_acc[1] = (short) (buffer[2] << 8 | buffer[3]); 00075 raw_acc[2] = (short) (buffer[4] << 8 | buffer[5]); 00076 } 00077 00078 void MPU6050::calibrate(int times, float separation_time) 00079 { 00080 // calibrate sensor with an average of count samples (result of calibration stored in offset[]) 00081 // Calibrate Gyroscope ---------------------------------- 00082 float calib_gyro[3] = {0,0,0}; // temporary array for the sum of calibration measurement 00083 00084 for (int i = 0; i < times; i++) { // read 'times' times the data in a very short time 00085 readraw_gyro(); 00086 for (int j = 0; j < 3; j++) 00087 calib_gyro[j] += raw_gyro[j]; 00088 wait(separation_time); 00089 } 00090 00091 for (int i = 0; i < 3; i++) 00092 offset_gyro[i] = calib_gyro[i]/times; // take the average of the calibration measurements 00093 00094 // Calibrate Accelerometer ------------------------------- 00095 float calib_acc[3] = {0,0,0}; // temporary array for the sum of calibration measurement 00096 00097 for (int i = 0; i < times; i++) { // read 'times' times the data in a very short time 00098 readraw_acc(); 00099 for (int j = 0; j < 3; j++) 00100 calib_acc[j] += raw_acc[j]; 00101 wait(separation_time); 00102 } 00103 00104 for (int i = 0; i < 2; i++) 00105 offset_acc[i] = calib_acc[i]/times; // take the average of the calibration measurements 00106 }
Generated on Thu Jul 14 2022 18:09:09 by
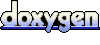