A fixed point library from Trenki http://www.trenki.net/content/view/17/1/ Slightly modified so that the fixed point data structure can automatically cast itself to float or int when used in an expression, and added the functionality to cast between precisions.
stdint.h
00001 /* ISO C9x 7.18 Integer types <stdint.h> 00002 * Based on ISO/IEC SC22/WG14 9899 Committee draft (SC22 N2794) 00003 * 00004 * THIS SOFTWARE IS NOT COPYRIGHTED 00005 * 00006 * Contributor: Danny Smith <danny_r_smith_2001@yahoo.co.nz> 00007 * 00008 * This source code is offered for use in the public domain. You may 00009 * use, modify or distribute it freely. 00010 * 00011 * This code is distributed in the hope that it will be useful but 00012 * WITHOUT ANY WARRANTY. ALL WARRANTIES, EXPRESS OR IMPLIED ARE HEREBY 00013 * DISCLAIMED. This includes but is not limited to warranties of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. 00015 * 00016 * Date: 2000-12-02 00017 */ 00018 00019 00020 #ifndef _STDINT_H 00021 #define _STDINT_H 00022 #define __need_wint_t 00023 #define __need_wchar_t 00024 #include <stddef.h> 00025 00026 /* 7.18.1.1 Exact-width integer types */ 00027 typedef signed char int8_t; 00028 typedef unsigned char uint8_t; 00029 typedef short int16_t; 00030 typedef unsigned short uint16_t; 00031 typedef int int32_t; 00032 typedef unsigned uint32_t; 00033 typedef long long int64_t; 00034 typedef unsigned long long uint64_t; 00035 00036 /* 7.18.1.2 Minimum-width integer types */ 00037 typedef signed char int_least8_t; 00038 typedef unsigned char uint_least8_t; 00039 typedef short int_least16_t; 00040 typedef unsigned short uint_least16_t; 00041 typedef int int_least32_t; 00042 typedef unsigned uint_least32_t; 00043 typedef long long int_least64_t; 00044 typedef unsigned long long uint_least64_t; 00045 00046 /* 7.18.1.3 Fastest minimum-width integer types 00047 * Not actually guaranteed to be fastest for all purposes 00048 * Here we use the exact-width types for 8 and 16-bit ints. 00049 */ 00050 typedef char int_fast8_t; 00051 typedef unsigned char uint_fast8_t; 00052 typedef short int_fast16_t; 00053 typedef unsigned short uint_fast16_t; 00054 typedef int int_fast32_t; 00055 typedef unsigned int uint_fast32_t; 00056 typedef long long int_fast64_t; 00057 typedef unsigned long long uint_fast64_t; 00058 00059 /* 7.18.1.4 Integer types capable of holding object pointers */ 00060 typedef int intptr_t; 00061 typedef unsigned uintptr_t; 00062 00063 /* 7.18.1.5 Greatest-width integer types */ 00064 typedef long long intmax_t; 00065 typedef unsigned long long uintmax_t; 00066 00067 /* 7.18.2 Limits of specified-width integer types */ 00068 #if !defined ( __cplusplus) || defined (__STDC_LIMIT_MACROS) 00069 00070 /* 7.18.2.1 Limits of exact-width integer types */ 00071 #define INT8_MIN (-128) 00072 #define INT16_MIN (-32768) 00073 #define INT32_MIN (-2147483647 - 1) 00074 #define INT64_MIN (-9223372036854775807LL - 1) 00075 00076 #define INT8_MAX 127 00077 #define INT16_MAX 32767 00078 #define INT32_MAX 2147483647 00079 #define INT64_MAX 9223372036854775807LL 00080 00081 #define UINT8_MAX 0xff /* 255U */ 00082 #define UINT16_MAX 0xffff /* 65535U */ 00083 #define UINT32_MAX 0xffffffff /* 4294967295U */ 00084 #define UINT64_MAX 0xffffffffffffffffULL /* 18446744073709551615ULL */ 00085 00086 /* 7.18.2.2 Limits of minimum-width integer types */ 00087 #define INT_LEAST8_MIN INT8_MIN 00088 #define INT_LEAST16_MIN INT16_MIN 00089 #define INT_LEAST32_MIN INT32_MIN 00090 #define INT_LEAST64_MIN INT64_MIN 00091 00092 #define INT_LEAST8_MAX INT8_MAX 00093 #define INT_LEAST16_MAX INT16_MAX 00094 #define INT_LEAST32_MAX INT32_MAX 00095 #define INT_LEAST64_MAX INT64_MAX 00096 00097 #define UINT_LEAST8_MAX UINT8_MAX 00098 #define UINT_LEAST16_MAX UINT16_MAX 00099 #define UINT_LEAST32_MAX UINT32_MAX 00100 #define UINT_LEAST64_MAX UINT64_MAX 00101 00102 /* 7.18.2.3 Limits of fastest minimum-width integer types */ 00103 #define INT_FAST8_MIN INT8_MIN 00104 #define INT_FAST16_MIN INT16_MIN 00105 #define INT_FAST32_MIN INT32_MIN 00106 #define INT_FAST64_MIN INT64_MIN 00107 00108 #define INT_FAST8_MAX INT8_MAX 00109 #define INT_FAST16_MAX INT16_MAX 00110 #define INT_FAST32_MAX INT32_MAX 00111 #define INT_FAST64_MAX INT64_MAX 00112 00113 #define UINT_FAST8_MAX UINT8_MAX 00114 #define UINT_FAST16_MAX UINT16_MAX 00115 #define UINT_FAST32_MAX UINT32_MAX 00116 #define UINT_FAST64_MAX UINT64_MAX 00117 00118 /* 7.18.2.4 Limits of integer types capable of holding 00119 object pointers */ 00120 #define INTPTR_MIN INT32_MIN 00121 #define INTPTR_MAX INT32_MAX 00122 #define UINTPTR_MAX UINT32_MAX 00123 00124 /* 7.18.2.5 Limits of greatest-width integer types */ 00125 #define INTMAX_MIN INT64_MIN 00126 #define INTMAX_MAX INT64_MAX 00127 #define UINTMAX_MAX UINT64_MAX 00128 00129 /* 7.18.3 Limits of other integer types */ 00130 #define PTRDIFF_MIN INT32_MIN 00131 #define PTRDIFF_MAX INT32_MAX 00132 00133 #define SIG_ATOMIC_MIN INT32_MIN 00134 #define SIG_ATOMIC_MAX INT32_MAX 00135 00136 #define SIZE_MAX UINT32_MAX 00137 00138 #ifndef WCHAR_MIN /* also in wchar.h */ 00139 #define WCHAR_MIN 0 00140 #define WCHAR_MAX 0xffff /* UINT16_MAX */ 00141 #endif 00142 00143 /* 00144 * wint_t is unsigned short for compatibility with MS runtime 00145 */ 00146 #define WINT_MIN 0 00147 #define WINT_MAX 0xffff /* UINT16_MAX */ 00148 00149 #endif /* !defined ( __cplusplus) || defined __STDC_LIMIT_MACROS */ 00150 00151 00152 /* 7.18.4 Macros for integer constants */ 00153 #if !defined ( __cplusplus) || defined (__STDC_CONSTANT_MACROS) 00154 00155 /* 7.18.4.1 Macros for minimum-width integer constants 00156 00157 Accoding to Douglas Gwyn <gwyn@arl.mil>: 00158 "This spec was changed in ISO/IEC 9899:1999 TC1; in ISO/IEC 00159 9899:1999 as initially published, the expansion was required 00160 to be an integer constant of precisely matching type, which 00161 is impossible to accomplish for the shorter types on most 00162 platforms, because C99 provides no standard way to designate 00163 an integer constant with width less than that of type int. 00164 TC1 changed this to require just an integer constant 00165 *expression* with *promoted* type." 00166 */ 00167 00168 #define INT8_C(val) ((int8_t) + (val)) 00169 #define UINT8_C(val) ((uint8_t) + (val##U)) 00170 #define INT16_C(val) ((int16_t) + (val)) 00171 #define UINT16_C(val) ((uint16_t) + (val##U)) 00172 00173 #define INT32_C(val) val##L 00174 #define UINT32_C(val) val##UL 00175 #define INT64_C(val) val##LL 00176 #define UINT64_C(val) val##ULL 00177 00178 /* 7.18.4.2 Macros for greatest-width integer constants */ 00179 #define INTMAX_C(val) INT64_C(val) 00180 #define UINTMAX_C(val) UINT64_C(val) 00181 00182 #endif /* !defined ( __cplusplus) || defined __STDC_CONSTANT_MACROS */ 00183 00184 #endif
Generated on Sat Jul 23 2022 12:09:06 by
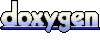