Kalman filter for Eurobot
Embed:
(wiki syntax)
Show/hide line numbers
RFSRF05.h
00001 /* mbed SRF05 Ultrasonic Rangefiner Library 00002 * Copyright (c) 2007-2010, cstyles, sford 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 00024 #ifndef MBED_RFSRF05_H 00025 #define MBED_RFSRF05_H 00026 00027 #include "mbed.h" 00028 #include "RF12B.h" 00029 00030 #define CODE0 0x22 00031 #define CODE1 0x44 00032 #define CODE2 0x88 00033 00034 /* SAMPLE IMPLEMENTATION! 00035 RFSRF05 my_srf(p13,p21,p22,p23,p24,p25,p26,p5,p6,p7,p8,p9); 00036 00037 00038 void callbinmain(int num, float dist) { 00039 //Here is where you deal with your brand new reading ;D 00040 } 00041 00042 int main() { 00043 pc.printf("Hello World of RobotSonar!\r\n"); 00044 my_srf.callbackfunc = callbinmain; 00045 00046 while (1); 00047 } 00048 00049 */ 00050 class DummyCT; 00051 00052 class RFSRF05 { 00053 public: 00054 00055 RFSRF05( 00056 PinName trigger, 00057 PinName echo0, 00058 PinName echo1, 00059 PinName echo2, 00060 PinName echo3, 00061 PinName echo4, 00062 PinName echo5, 00063 PinName SDI, 00064 PinName SDO, 00065 PinName SCK, 00066 PinName NCS, 00067 PinName NIRQ); 00068 00069 /** A non-blocking function that will return the last measurement 00070 * 00071 * @returns floating point representation of distance in cm 00072 */ 00073 float read0(); 00074 float read1(); 00075 float read2(); 00076 00077 /** A assigns a callback function when a new reading is available **/ 00078 void (*callbackfunc)(int beaconnum, float distance); 00079 DummyCT* callbackobj; 00080 void (DummyCT::*mcallbackfunc)(int beaconnum, float distance); 00081 00082 00083 00084 /** A short hand way of using the read function */ 00085 operator float(); 00086 00087 private : 00088 RF12B _rf; 00089 DigitalOut _trigger; 00090 InterruptIn _echo0; 00091 InterruptIn _echo1; 00092 InterruptIn _echo2; 00093 InterruptIn _echo3; 00094 InterruptIn _echo4; 00095 InterruptIn _echo5; 00096 Timer _timer; 00097 Ticker _ticker; 00098 void _rising (void); 00099 void _falling (void); 00100 void _startRange (void); 00101 float _dist[3]; 00102 char _code[3]; 00103 int _beacon_counter; 00104 bool ValidPulse; 00105 00106 }; 00107 00108 #endif
Generated on Wed Jul 13 2022 22:04:07 by
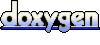