Extended library to include a screensaver
Fork of 4DGL-uLCD-SE by
Embed:
(wiki syntax)
Show/hide line numbers
uLCD_4DGL_Graphics.cpp
00001 // 00002 // uLCD_4DGL is a class to drive 4D Systems LCD screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // Modifed for Goldelox processor <2013> Jim Hamblen 00006 // 00007 // uLCD_4DGL is free software: you can redistribute it and/or modify 00008 // it under the terms of the GNU General Public License as published by 00009 // the Free Software Foundation, either version 3 of the License, or 00010 // (at your option) any later version. 00011 // 00012 // uLCD_4DGL is distributed in the hope that it will be useful, 00013 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 // GNU General Public License for more details. 00016 // 00017 // You should have received a copy of the GNU General Public License 00018 // along with uLCD_4DGL. If not, see <http://www.gnu.org/licenses/>. 00019 00020 #include "mbed.h" 00021 #include "uLCD_4DGL.h" 00022 00023 #define ARRAY_SIZE(X) sizeof(X)/sizeof(X[0]) 00024 00025 //**************************************************************************************************** 00026 void uLCD_4DGL :: circle(int x, int y , int radius, int color, bool isScreenSaverCommand) // draw a circle in (x,y) 00027 { 00028 char command[9]= ""; 00029 00030 command[0] = CIRCLE; 00031 00032 command[1] = (x >> 8) & 0xFF; 00033 command[2] = x & 0xFF; 00034 00035 command[3] = (y >> 8) & 0xFF; 00036 command[4] = y & 0xFF; 00037 00038 command[5] = (radius >> 8) & 0xFF; 00039 command[6] = radius & 0xFF; 00040 00041 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00042 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00043 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00044 00045 command[7] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00046 command[8] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00047 00048 writeCOMMAND(command, 9); 00049 if (!isScreenSaverCommand) reportScreenInteraction(); 00050 } 00051 //**************************************************************************************************** 00052 void uLCD_4DGL :: filled_circle(int x, int y , int radius, int color, bool isScreenSaverCommand) // draw a circle in (x,y) 00053 { 00054 char command[9]= ""; 00055 00056 command[0] = FCIRCLE; 00057 00058 command[1] = (x >> 8) & 0xFF; 00059 command[2] = x & 0xFF; 00060 00061 command[3] = (y >> 8) & 0xFF; 00062 command[4] = y & 0xFF; 00063 00064 command[5] = (radius >> 8) & 0xFF; 00065 command[6] = radius & 0xFF; 00066 00067 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00068 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00069 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00070 00071 command[7] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00072 command[8] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00073 00074 writeCOMMAND(command, 9); 00075 if (!isScreenSaverCommand) reportScreenInteraction(); 00076 } 00077 00078 //**************************************************************************************************** 00079 void uLCD_4DGL :: triangle(int x1, int y1 , int x2, int y2, int x3, int y3, int color, bool isScreenSaverCommand) // draw a triangle 00080 { 00081 char command[15]= ""; 00082 00083 command[0] = TRIANGLE; 00084 00085 command[1] = (x1 >> 8) & 0xFF; 00086 command[2] = x1 & 0xFF; 00087 00088 command[3] = (y1 >> 8) & 0xFF; 00089 command[4] = y1 & 0xFF; 00090 00091 command[5] = (x2 >> 8) & 0xFF; 00092 command[6] = x2 & 0xFF; 00093 00094 command[7] = (y2 >> 8) & 0xFF; 00095 command[8] = y2 & 0xFF; 00096 00097 command[9] = (x3 >> 8) & 0xFF; 00098 command[10] = x3 & 0xFF; 00099 00100 command[11] = (y3 >> 8) & 0xFF; 00101 command[12] = y3 & 0xFF; 00102 00103 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00104 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00105 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00106 00107 command[13] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00108 command[14] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00109 00110 writeCOMMAND(command, 15); 00111 if (!isScreenSaverCommand) reportScreenInteraction(); 00112 } 00113 00114 //**************************************************************************************************** 00115 void uLCD_4DGL :: line(int x1, int y1 , int x2, int y2, int color, bool isScreenSaverCommand) // draw a line 00116 { 00117 char command[11]= ""; 00118 00119 command[0] = LINE; 00120 00121 command[1] = (x1 >> 8) & 0xFF; 00122 command[2] = x1 & 0xFF; 00123 00124 command[3] = (y1 >> 8) & 0xFF; 00125 command[4] = y1 & 0xFF; 00126 00127 command[5] = (x2 >> 8) & 0xFF; 00128 command[6] = x2 & 0xFF; 00129 00130 command[7] = (y2 >> 8) & 0xFF; 00131 command[8] = y2 & 0xFF; 00132 00133 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00134 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00135 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00136 00137 command[9] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00138 command[10] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00139 00140 writeCOMMAND(command, 11); 00141 if (!isScreenSaverCommand) reportScreenInteraction(); 00142 } 00143 00144 //**************************************************************************************************** 00145 void uLCD_4DGL :: rectangle(int x1, int y1 , int x2, int y2, int color, bool isScreenSaverCommand) // draw a rectangle 00146 { 00147 char command[11]= ""; 00148 00149 command[0] = RECTANGLE; 00150 00151 command[1] = (x1 >> 8) & 0xFF; 00152 command[2] = x1 & 0xFF; 00153 00154 command[3] = (y1 >> 8) & 0xFF; 00155 command[4] = y1 & 0xFF; 00156 00157 command[5] = (x2 >> 8) & 0xFF; 00158 command[6] = x2 & 0xFF; 00159 00160 command[7] = (y2 >> 8) & 0xFF; 00161 command[8] = y2 & 0xFF; 00162 00163 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00164 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00165 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00166 00167 command[9] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00168 command[10] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00169 00170 writeCOMMAND(command, 11); 00171 if (!isScreenSaverCommand) reportScreenInteraction(); 00172 } 00173 00174 //**************************************************************************************************** 00175 void uLCD_4DGL :: filled_rectangle(int x1, int y1 , int x2, int y2, int color, bool isScreenSaverCommand) // draw a rectangle 00176 { 00177 char command[11]= ""; 00178 00179 command[0] = FRECTANGLE; 00180 00181 command[1] = (x1 >> 8) & 0xFF; 00182 command[2] = x1 & 0xFF; 00183 00184 command[3] = (y1 >> 8) & 0xFF; 00185 command[4] = y1 & 0xFF; 00186 00187 command[5] = (x2 >> 8) & 0xFF; 00188 command[6] = x2 & 0xFF; 00189 00190 command[7] = (y2 >> 8) & 0xFF; 00191 command[8] = y2 & 0xFF; 00192 00193 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00194 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00195 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00196 00197 command[9] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00198 command[10] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00199 00200 writeCOMMAND(command, 11); 00201 if (!isScreenSaverCommand) reportScreenInteraction(); 00202 } 00203 00204 00205 00206 //**************************************************************************************************** 00207 void uLCD_4DGL :: pixel(int x, int y, int color, bool isScreenSaverCommand) // draw a pixel 00208 { 00209 char command[7]= ""; 00210 00211 command[0] = PIXEL; 00212 00213 command[1] = (x >> 8) & 0xFF; 00214 command[2] = x & 0xFF; 00215 00216 command[3] = (y >> 8) & 0xFF; 00217 command[4] = y & 0xFF; 00218 00219 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00220 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00221 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00222 00223 command[5] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00224 command[6] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00225 00226 writeCOMMAND(command, 7); 00227 if (!isScreenSaverCommand) reportScreenInteraction(); 00228 } 00229 //**************************************************************************************************** 00230 void uLCD_4DGL :: BLIT(int x, int y, int w, int h, int *colors, bool isScreenSaverCommand) // draw a block of pixels 00231 { 00232 int red5, green6, blue5; 00233 writeBYTEfast('\x00'); 00234 writeBYTEfast(BLITCOM); 00235 writeBYTEfast((x >> 8) & 0xFF); 00236 writeBYTEfast(x & 0xFF); 00237 writeBYTEfast((y >> 8) & 0xFF); 00238 writeBYTEfast(y & 0xFF); 00239 writeBYTEfast((w >> 8) & 0xFF); 00240 writeBYTE(w & 0xFF); 00241 writeBYTE((h >> 8) & 0xFF); 00242 writeBYTE(h & 0xFF); 00243 wait_ms(1); 00244 for (int i=0; i<w*h; i++) { 00245 red5 = (colors[i] >> (16 + 3)) & 0x1F; // get red on 5 bits 00246 green6 = (colors[i] >> (8 + 2)) & 0x3F; // get green on 6 bits 00247 blue5 = (colors[i] >> (0 + 3)) & 0x1F; // get blue on 5 bits 00248 writeBYTEfast(((red5 << 3) + (green6 >> 3)) & 0xFF); // first part of 16 bits color 00249 writeBYTEfast(((green6 << 5) + (blue5 >> 0)) & 0xFF); // second part of 16 bits color 00250 } 00251 int resp=0; 00252 while (!_cmd.readable()) wait_ms(TEMPO); // wait for screen answer 00253 if (_cmd.readable()) resp = _cmd.getc(); // read response if any 00254 switch (resp) { 00255 case ACK : // if OK return 1 00256 resp = 1; 00257 break; 00258 case NAK : // if NOK return -1 00259 resp = -1; 00260 break; 00261 default : 00262 resp = 0; // else return 0 00263 break; 00264 } 00265 #if DEBUGMODE 00266 pc.printf(" Answer received : %d\n",resp); 00267 #endif 00268 if (!isScreenSaverCommand) reportScreenInteraction(); 00269 } 00270 //****************************************************************************************************** 00271 int uLCD_4DGL :: read_pixel(int x, int y) // read screen info and populate data 00272 { 00273 00274 char command[6]= ""; 00275 command[0] = 0xFF; 00276 command[1] = READPIXEL; 00277 00278 command[2] = (x >> 8) & 0xFF; 00279 command[3] = x & 0xFF; 00280 00281 command[4] = (y >> 8) & 0xFF; 00282 command[5] = y & 0xFF; 00283 00284 int i, temp = 0, color = 0, resp = 0; 00285 char response[2] = ""; 00286 00287 freeBUFFER(); 00288 00289 for (i = 0; i < 6; i++) { // send all chars to serial port 00290 writeBYTE(command[i]); 00291 } 00292 00293 while (!_cmd.readable()) wait_ms(TEMPO); // wait for screen answer 00294 00295 while (_cmd.readable() && resp < ARRAY_SIZE(response)) { 00296 temp = _cmd.getc(); 00297 response[resp++] = (char)temp; 00298 } 00299 00300 color = ((response[0] << 8) + response[1]); 00301 00302 return color; // WARNING : this is 16bits color, not 24bits... need to be fixed 00303 } 00304 00305 00306 //**************************************************************************************************** 00307 void uLCD_4DGL :: pen_size(char mode) // set pen to SOLID or WIREFRAME 00308 { 00309 char command[2]= ""; 00310 00311 command[0] = PENSIZE; 00312 command[1] = mode; 00313 writeCOMMAND(command, 2); 00314 } 00315 00316 00317
Generated on Fri Jul 15 2022 01:44:25 by
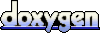