+++
Fork of Watchdog by
Embed:
(wiki syntax)
Show/hide line numbers
Watchdog.h
Go to the documentation of this file.
00001 /// @file Watchdog.h provides the interface to the Watchdog module 00002 /// 00003 /// This provides basic Watchdog service for the mbed. You can configure 00004 /// various timeout intervals that meet your system needs. Additionally, 00005 /// it is possible to identify if the Watchdog was the cause of any 00006 /// system restart, permitting the application code to take appropriate 00007 /// behavior. 00008 /// 00009 /// Adapted from Simon's Watchdog code from http://mbed.org/forum/mbed/topic/508/ 00010 /// 00011 /// @note Copyright © 2011,2015 by Smartware Computing, all rights reserved. 00012 /// This software may be used to derive new software, as long as 00013 /// this copyright statement remains in the source file. 00014 /// @author David Smart 00015 /// 00016 /// History 00017 /// \li v2.00 - 20150315: Enhanced beyond TARGET_LPC1768 to TARGET_LPC4088, TARGET_STM 00018 /// \li v1.00 - 20110616: initial release with some documentation improvements 00019 /// 00020 #ifndef WATCHDOG_H 00021 #define WATCHDOG_H 00022 #include "mbed.h" 00023 00024 /// The Watchdog class provides the interface to the Watchdog feature 00025 /// 00026 /// Embedded programs, by their nature, are usually unattended. If things 00027 /// go wrong, it is usually important that the system attempts to recover. 00028 /// Aside from robust software, a hardware watchdog can monitor the 00029 /// system and initiate a system reset when appropriate. 00030 /// 00031 /// This Watchdog is patterned after one found elsewhere on the mbed site, 00032 /// however this one also provides a method for the application software 00033 /// to determine the cause of the reset - watchdog or otherwise. 00034 /// 00035 /// Supports: 00036 /// \li TARGET_LPC1768 00037 /// \li TARGET_LPC4088 00038 /// \li TARGET_STM 00039 /// 00040 /// example: 00041 /// @code 00042 /// Watchdog wd; 00043 /// 00044 /// ... 00045 /// main() { 00046 /// if (wd.WatchdogCausedReset()) 00047 /// pc.printf("Watchdog caused reset.\r\n"); 00048 /// 00049 /// wd.Configure(3.0); // sets the timeout interval 00050 /// for (;;) { 00051 /// wd.Service(); // kick the dog before the timeout 00052 /// // do other work 00053 /// } 00054 /// } 00055 /// @endcode 00056 /// 00057 class Watchdog { 00058 public: 00059 /// Create a Watchdog object 00060 /// 00061 /// example: 00062 /// @code 00063 /// Watchdog wd; // placed before main 00064 /// @endcode 00065 Watchdog(); 00066 00067 /// Configure the timeout for the Watchdog 00068 /// 00069 /// This configures the Watchdog service and starts it. It must 00070 /// be serviced before the timeout, or the system will be restarted. 00071 /// 00072 /// example: 00073 /// @code 00074 /// ... 00075 /// wd.Configure(1.4); // configure for a 1.4 second timeout 00076 /// ... 00077 /// @endcode 00078 /// 00079 /// @param[in] timeout in seconds, as a floating point number 00080 /// @returns none 00081 /// 00082 void Configure(float timeout); 00083 00084 /// Service the Watchdog so it does not cause a system reset 00085 /// 00086 /// example: 00087 /// @code 00088 /// wd.Service(); 00089 /// @endcode 00090 /// @returns none 00091 void Service(); 00092 00093 /// WatchdogCausedReset identifies if the cause of the system 00094 /// reset was the Watchdog 00095 /// 00096 /// example: 00097 /// @code 00098 /// if (wd.WatchdogCausedReset())) { 00099 /// @endcode 00100 /// 00101 /// @returns true if the Watchdog was the cause of the reset 00102 bool WatchdogCausedReset(); 00103 private: 00104 bool wdreset; 00105 }; 00106 00107 #endif // WATCHDOG_H
Generated on Wed Jul 13 2022 02:27:12 by
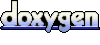