
JVM test
Embed:
(wiki syntax)
Show/hide line numbers
device_depend.cpp
00001 #include <stdio.h> 00002 #include <string.h> 00003 #include "device_depend.h" 00004 00005 #define bc_str_length 2048 // from 32 to 1024 00006 00007 BusInOut ledport(LED1, LED2, LED3, LED4); 00008 Serial pc(USBTX, USBRX); // tx, rx 00009 LocalFileSystem local("local"); 00010 FILE* ReadFile; 00011 Ticker tick; 00012 00013 volatile static unsigned long msTicks; /* counts 1ms timeTicks */ 00014 char bc_str[bc_str_length]; 00015 int last_bc_num = 0; 00016 00017 void hardware_init(void){ 00018 setup_systick(); 00019 ledport.output(); 00020 } 00021 00022 void uart_print(char *str){ 00023 pc.printf(str); 00024 return; 00025 } 00026 00027 void uart_init(int baud_rate){ 00028 00029 } 00030 00031 int uart_read(void){ 00032 return pc.getc(); 00033 } 00034 00035 int time_millis(void){ 00036 return msTicks; 00037 } 00038 00039 void port_write(int port, int bit, int value){ 00040 if((bit < 0)||(bit > 3))return; 00041 int i = ledport; 00042 if(value) 00043 i |= (1 << bit); 00044 else 00045 i &= ~(1 << bit); 00046 00047 ledport = i; 00048 return; 00049 } 00050 00051 void bytecode_read_init(void){ 00052 00053 if(NULL == (ReadFile = fopen ("/local/Test.cla","rb"))){ uart_print(" ERROR:Can't read class File.\r\n"); while(1); } 00054 fseek(ReadFile, 0, SEEK_SET); 00055 // I don't know why, but fseek does not work right. So I use fgetc instead. 00056 //fgets(bc_str, bc_str_length, ReadFile); 00057 printf("loading..."); 00058 for(int i = 0; i < bc_str_length; i++) bc_str[i] = fgetc(ReadFile); 00059 printf("end\r\n"); 00060 //last_bc_num = bc_str_length; 00061 } 00062 00063 char* bytecode_read(int bc_num, int length){ 00064 00065 if((last_bc_num <= bc_num)&&(bc_num < last_bc_num + bc_str_length)&&(length <= bc_str_length)){ // exists data in buffer 00066 return &bc_str[bc_num - last_bc_num]; 00067 }else 00068 { 00069 fseek(ReadFile, bc_num, SEEK_SET); 00070 for(int i = 0; i < bc_str_length; i++) 00071 { 00072 bc_str[i] = fgetc(ReadFile); 00073 } 00074 last_bc_num = bc_num; 00075 } 00076 return bc_str; 00077 } 00078 00079 /*---------------------------------------------------------------------------- 00080 SysTick_Handler 00081 *----------------------------------------------------------------------------*/ 00082 void SysTickCount(void) { 00083 msTicks++; /* increment counter necessary in Delay() */ 00084 } 00085 00086 void setup_systick (void) { 00087 msTicks = 0; 00088 tick.attach_us(&SysTickCount, 1000); //set 32kHz/8(word FIFO) sampling data } 00089 } 00090
Generated on Tue Jul 12 2022 21:16:03 by
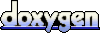