
weahter seoul
Dependencies: Adafruit_GFX WIZnetInterface mbed
Fork of Weather_Forecast_Helloworld_WIZwiki-W7500 by
main.cpp
00001 00002 #include "mbed.h" 00003 #include "EthernetInterface.h" 00004 #include "Adafruit_SSD1306.h" 00005 00006 /* 00007 * Sheild LED 00008 */ 00009 // DigitalOut rled(D9); 00010 // DigitalOut gled(D10); 00011 // DigitalOut bled(D11); 00012 00013 /* 00014 * W7500 onboard LED 00015 */ 00016 DigitalOut rled(LED1); 00017 DigitalOut gled(LED2); 00018 DigitalOut bled(LED3); 00019 00020 00021 class I2CPreInit : public I2C 00022 { 00023 public: 00024 I2CPreInit(PinName sda, PinName scl) : I2C(sda, scl) 00025 { 00026 frequency(100000); 00027 start(); 00028 }; 00029 }; 00030 00031 I2CPreInit gI2C(PA_10,PA_9); 00032 00033 /* 00034 * set OLED 00035 */ 00036 Adafruit_SSD1306_I2c gOled(gI2C,NC,0x78,64,128); 00037 00038 00039 /* 00040 * LED desplay functions 00041 */ 00042 void clean(){ 00043 rled = 0; 00044 gled = 0; 00045 bled = 1; 00046 } 00047 void clouds(){ 00048 rled = 0; 00049 gled = 0; 00050 bled = 0; 00051 } 00052 void thunderstorm(){ 00053 while(1){ 00054 rled = 0; 00055 gled = 0; 00056 bled = 0; 00057 wait(0.5); 00058 rled = 0; 00059 gled = 0; 00060 bled = 0; 00061 wait(0.5); 00062 }; 00063 } 00064 void rain(){ 00065 rled = 1; 00066 gled = 1; 00067 bled = 0; 00068 } 00069 void mist(){ 00070 while(1){ 00071 rled = 1; 00072 gled = 1; 00073 bled = 0; 00074 wait(0.5); 00075 rled = 1; 00076 gled = 1; 00077 bled = 1; 00078 wait(0.5); 00079 }; 00080 } 00081 void haze(){ 00082 rled = 0; 00083 gled = 1; 00084 bled = 0; 00085 } 00086 void fog(){ 00087 while(1){ 00088 rled = 1; 00089 gled = 0; 00090 bled = 0; 00091 wait(1.0); 00092 rled = 1; 00093 gled = 1; 00094 bled = 0; 00095 wait(1.0); 00096 }; 00097 } 00098 void error(){ 00099 rled = 1; 00100 gled = 1; 00101 bled = 1; 00102 } 00103 00104 /* 00105 * main 00106 */ 00107 int main() { 00108 00109 int phy_link; 00110 printf("Wait a second...\r\n"); 00111 uint8_t mac_addr[6] = {0x00, 0x08, 0xDC, 0xff, 0xff, 0x17}; //7500맥 구매 주 00112 00113 EthernetInterface eth; 00114 00115 eth.init(mac_addr); //Use DHCP 00116 00117 while(1){ 00118 00119 eth.connect(); 00120 00121 /* 00122 * phy link 00123 */ 00124 do{ 00125 phy_link = eth.ethernet_link(); 00126 printf("..."); 00127 wait(2); 00128 }while(!phy_link); 00129 printf("\r\n"); 00130 00131 printf("IP Address is %s\r\n\r\n", eth.getIPAddress()); 00132 00133 /* 00134 * TCP socket connect to openweather server 00135 */ 00136 TCPSocketConnection sock; 00137 sock.connect("api.openweathermap.org", 80); 00138 00139 00140 /* 00141 * GET method, to request weather forecast 00142 */ 00143 char http_cmd[] = "GET /data/2.5/weather?q=weather?q=Seoul,Kr&appid=2de143494c0b295cca9337e1e96b00e0 HTTP/1.0\n\n"; 00144 //char http_cmd[] = "GET /data/2.5/weather?q=London,uk HTTP/1.0\n\n"; 00145 //char http_cmd[] = "GET /data/2.5/weather?q=Berlin,de HTTP/1.0\n\n"; 00146 sock.send_all(http_cmd, sizeof(http_cmd)-1); 00147 00148 /* 00149 * get data into buffer 00150 */ 00151 char buffer[1024]; 00152 int ret; 00153 while (true) { 00154 ret = sock.receive(buffer, sizeof(buffer)-1); 00155 if (ret <= 0) 00156 break; 00157 buffer[ret] = '\0'; 00158 printf("Received %d chars from server: %s\n", ret, buffer); 00159 } 00160 printf("\r\n\r\n"); 00161 00162 /* 00163 * parsing current date, weather, city, tempurature 00164 */ 00165 char *date; 00166 char *weather; 00167 char *city; 00168 char *temper; 00169 00170 char cur_date[17] = {0}; 00171 char weather_con[15] = {0}; 00172 char city_name[10] = {0}; 00173 char temper_data[3] = {0}; 00174 00175 int temp; 00176 int num100, num10, num1; 00177 00178 /* 00179 * parding date 00180 */ 00181 date = strstr(buffer, "Date"); 00182 for(int x=0;x<17;x++){ 00183 cur_date[x] = date[x+6]; 00184 } 00185 00186 /* 00187 * parsing weather condition 00188 */ 00189 weather = strstr(buffer, "main"); 00190 for(int i=0; i<15;i++){ 00191 weather_con[i] = weather[i+7]; 00192 if(weather_con[i] == 34){ 00193 weather_con[i] = 0; 00194 break; 00195 } 00196 } 00197 00198 /* 00199 * parsing city name 00200 */ 00201 city = strstr(buffer, "name"); 00202 for(int j=0; j<10;j++){ 00203 city_name[j] = city[j+7]; 00204 if(city_name[j] == 34){ 00205 city_name[j] = 0; 00206 break; 00207 } 00208 } 00209 00210 /* 00211 * parsing current tempurature 00212 */ 00213 temper = strstr(buffer, "temp"); 00214 for(int k=0; k<3;k++){ 00215 temper_data[k] = temper[k+6]; 00216 } 00217 00218 /* 00219 * kelvin to celius converter 00220 */ 00221 num100 = temper_data[0] - 48; 00222 num10 = temper_data[1] - 48; 00223 num1 = temper_data[2] - 48; 00224 00225 temp = (num100*100 + num10*10 + num1) - 273; 00226 00227 /* 00228 * printf 00229 */ 00230 printf("city name : %s\r\n", city_name); 00231 printf("weather : %s\r\n", weather_con); 00232 printf("temperature : %d\r\n\r\n", temp); 00233 00234 /* 00235 * OLED Display 00236 */ 00237 gOled.begin(); 00238 gOled.clearDisplay(); 00239 00240 gOled.printf("%s\n\n", cur_date); 00241 gOled.printf("City : %s\n", city_name); 00242 gOled.printf("Weather : %s\n", weather_con); 00243 gOled.printf("Temper : %d\n", temp); 00244 gOled.display(); 00245 gOled.setTextCursor(0,0); 00246 00247 /* 00248 * LED display rely on weather condition 00249 */ 00250 if(strcmp(weather_con,"Clouds")==0) clouds(); 00251 else if(strcmp(weather_con,"Rain")==0) rain(); 00252 else if(strcmp(weather_con,"Thunderstorm")==0) thunderstorm(); 00253 else if(strcmp(weather_con,"Clean")==0) clean(); 00254 else if(strcmp(weather_con,"Mist")==0) mist(); 00255 else if(strcmp(weather_con,"Haze")==0) haze(); 00256 else if(strcmp(weather_con,"Fog")==0) fog(); 00257 else error(); 00258 00259 00260 sock.close(); 00261 00262 eth.disconnect(); 00263 00264 /* 00265 * everytime in delay, request weather forecast 00266 */ 00267 wait(60.0); 00268 00269 }; 00270 00271 }
Generated on Fri Jul 15 2022 18:35:34 by
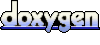