
This is my first program on mbed.org. A Small program for KL25Z that'll act as a Volume Control, thanks to samux for his code with USB keyboard support (https://mbed.org/users/samux/code/USBKeyboard_HelloWorld/) Just added functionality to let the user use the Touch Slider on the KL25Z board to control system volume.
Dependencies: mbed TSI MMA8451Q USBDevice
Fork of USBKeyboard_HelloWorld by
main.cpp
00001 /** 00002 * A simple app to make Volume Control with the KL25Z onboard Touch Slider 00003 * 00004 * Simply use the top and bottom part of the slider as buttons. 00005 * 00006 * Green led's intensity is controlled by the slider. 00007 * The Red component of the RGB led is changing intensity as you tilt the board on the X coordinate to have multiple actions at the same time. 00008 */ 00009 00010 #include "mbed.h" 00011 #include "MMA8451Q.h" 00012 #include "USBKeyboard.h" 00013 #include "TSISensor.h" 00014 00015 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00016 00017 int main(void) { 00018 ///get leds, and set def. values 00019 PwmOut bled(LED_BLUE); 00020 bled=0.8; 00021 PwmOut rled(LED_RED); 00022 rled=1; 00023 PwmOut gled(LED_GREEN); 00024 gled=1; 00025 ///initializing the accelerometer 00026 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS); 00027 bled=0.6; 00028 //USB Keyboard interface: 00029 USBKeyboard keyboard; 00030 bled=0.4; 00031 //Touch Slider: 00032 TSISensor tsi; 00033 bled=0.2; 00034 00035 ///switch on blue led 00036 bled=0.9; 00037 ///Wait until keyboard is configured 00038 while (!keyboard.configured()) { 00039 bled=0.1; 00040 wait(1); 00041 bled=0.9; 00042 wait(1); 00043 } 00044 bled=1; 00045 00046 float current=-1; //< Current state of Slider 00047 float newRead; //< New readings of the slider 00048 00049 while (true) { 00050 newRead=tsi.readPercentage(); 00051 gled=1.0 - newRead; 00052 /// only if the slider is touched 00053 if (newRead>0) 00054 if (current!=newRead) { 00055 ///and you have a different reading. 00056 ///this is basically for not letting the volume be changed in every .1 seconds, and keeping the code responsive 00057 ///you could have a counter on every 10th reading or so. 00058 current=newRead; 00059 if (current<0.4) 00060 //if touched in the bottom half lower the volume 00061 keyboard.mediaControl(KEY_VOLUME_DOWN); 00062 else 00063 if (current<0.6) 00064 //if touched in the bottom half lower the volume 00065 keyboard.mediaControl(KEY_PLAY_PAUSE); 00066 else 00067 //if touched in the top half rise the volume 00068 keyboard.mediaControl(KEY_VOLUME_UP); 00069 } 00070 rled = 1.0 - (abs(acc.getAccX())/2); 00071 wait(0.1); 00072 } 00073 }
Generated on Fri Aug 19 2022 01:11:04 by
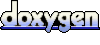