
The Nucleo Distance Calculator Simple code to use HCSR04 and nokia 5110 to display distance and ring a buzzer when the distance is lower than a certain threshold.
Dependencies: N5110 NOKIA_5110 mbed
Fork of UltraTest by
main.cpp
00001 //================================== 00002 // The Nucleo Distance Calculator 00003 // v. 1.0 00004 //================================== 00005 // by Lorenzo Arrigoni 00006 // laelectronics@libero.it 00007 //---------------------------------- 00008 // November 2015 00009 //////////////////////////////////////////// 00010 // This is a simple that measures the distance 00011 // and signals in video and with a buzzer if 00012 // the measured distance is below a certain threshold. 00013 ////////////////////////////////////////// 00014 00015 #include "mbed.h" 00016 #include "HCSR04.h" 00017 #include "NOKIA_5110.h" 00018 00019 00020 DigitalOut buzzer(D7) ; 00021 HCSR04 ultra(D3,D4); //pin trigger, pin echo 00022 00023 //treshold for buzzer 00024 #define treshold 5 // cm 00025 00026 int start_position =0.5 ; 00027 int current_position = start_position ; 00028 00029 // swith on/off for bluetooth 00030 #define BLUE 0 00031 00032 // debug mode 00033 #define DEBUG 1 00034 00035 #if DEBUG == 0 00036 //#define PC_DEBUG(args...) pc.printf(args) 00037 #else 00038 //#define PC_DEBUG(args...) 00039 #endif 00040 00041 #if BLUE == 1 00042 Serial pc(D8,D2); 00043 #else 00044 Serial pc(USBTX, USBRX); 00045 #endif 00046 00047 00048 00049 00050 float range=0; 00051 //char gg ; 00052 00053 void blink_buzz(DigitalOut name, float time) 00054 { 00055 name = 1 ; 00056 wait_ms(time); 00057 name = 0 ; 00058 } 00059 00060 int main() { 00061 00062 // initialize 00063 buzzer = 0 ; 00064 00065 LcdPins myPins; 00066 myPins.sce = D8; 00067 myPins.rst = D9; 00068 myPins.dc = D10; 00069 myPins.mosi = D11; //SPI_MOSI; 00070 myPins.miso = NC; 00071 myPins.sclk = D13; //SPI_SCK; 00072 NokiaLcd myLcd( myPins ); 00073 00074 00075 /// LCD initialization 00076 myLcd.InitLcd(); // LCD init 00077 myLcd.DrawString("Loarri - 2015"); 00078 myLcd.SetXY(0,2); 00079 myLcd.DrawString("LCD set up complete"); 00080 myLcd.SetXY(0,2); 00081 wait(2); 00082 myLcd.ClearLcdMem(); 00083 /// end LCD initialization 00084 00085 00086 //pc.printf("Program Start\n\r"); 00087 myLcd.DrawString("Program Start"); 00088 wait(1); 00089 myLcd.ClearLcdMem(); 00090 //myservo = start_position ; //set servo at 0 degree 00091 current_position = start_position ; 00092 while(1) { 00093 //myLcd.ClearLcdMem(); 00094 ultra.startMeas(); 00095 00096 wait(0.2); 00097 00098 00099 if( ultra.getMeas(range) == RANGE_MEAS_VALID) 00100 { 00101 pc.printf("Range -> %f cm\n\r", range); 00102 myLcd.SetXY(0,1); 00103 myLcd.DrawString("Range -> "); 00104 myLcd.SetXY(0,2); 00105 int i=(int)range; 00106 pc.printf("valore di i -> %i cm\n\r", i); 00107 char gg[4] ; 00108 sprintf(gg, "%f", range); 00109 pc.printf("valore di gg -> %c cm\n\r", gg); 00110 //gg = NokiaLcd::NumToStr(range) ; 00111 myLcd.DrawString(gg); 00112 myLcd.SetXY(0,3); 00113 myLcd.DrawString(" cm"); 00114 if (range < treshold ) { 00115 blink_buzz(buzzer,10); 00116 myLcd.ClearLcdMem(); 00117 myLcd.SetXY(0,1); 00118 myLcd.DrawString("Soglia superata."); 00119 wait(1); 00120 myLcd.ClearLcdMem(); 00121 } 00122 } 00123 } 00124 }
Generated on Wed Jul 20 2022 07:53:57 by
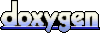