
Test for MCP4141. 7-bit digital potentiometer with SPI interface.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 // MCP4141. 2.7 to 5.5V 7-bit linear digital potentiometer with SPI interface. 00004 // Wiper commands can be write or read. This program is to write commands only. 00005 // MOSI/MISO are multiplexed in the MCP4141 side. 00006 00007 // Author: Lluis Nadal. August 2011. 00008 00009 00010 DigitalOut leds[] = {(LED1), (LED2),(LED3),(LED4)}; 00011 00012 //Define SPI mBed side: 00013 SPI spi(p5, p6, p7); // mosi, miso, sclk. To write commands only, you do not need to connect p6(miso) to MCP4141. 00014 DigitalOut cs(p8); // Chip select. Active low. 00015 00016 /* 00017 MCP4141 side: 00018 p8 = Vdd: +3.3V. p4 = Vss: GROUND. p1: cs (mBed's p8). 00019 p2: sclk (mBed's p7). p3: SDI (mBed's p5). p5: potentiometer a. p7: potentiometer b. 00020 Rab: 5k, 10k 50k or 100k options. p6: wiper (75 Ohm). 00021 Attach ohmeter to pins p6 and p7. 00022 */ 00023 00024 void write(int n) { // 0 >= n <= 128 00025 cs=1; 00026 cs=0; 00027 spi.write(0x00); // Address(volatile) 0000, write=00, data=00 = 0x00 00028 spi.write(n); // Data 00029 cs=1; 00030 } 00031 00032 void increment() { 00033 cs=1; 00034 cs=0; 00035 spi.write(0x07);// Address(volatile) 0000, increment 0100 = 0x07 00036 cs=1; 00037 } 00038 00039 void decrement() { 00040 cs=0; 00041 spi.write(0x0B);// Address(volatile) 0000, decrement 1000 = 0x0B 00042 cs=1; 00043 } 00044 00045 00046 00047 int main() { 00048 00049 cs=1; // Disables MCP4141 00050 00051 spi.format(8,0); // 8 bits , mode 0 00052 spi.frequency(1000000); // Default SPI frequency. ( 250kHz maximum for read commands). 00053 00054 while (1) { 00055 00056 leds[0]=0; 00057 leds[1]=0; 00058 leds[2]=0; 00059 leds[3]=0; // All leds off. 00060 wait(1); 00061 00062 write(32);// Set wiper to 32/128 = 1/4 Rab 00063 leds[0]=1; 00064 wait(4); 00065 00066 write(64);// Set wiper to 64/128 = 1/2 Rab 00067 leds[1]=1; 00068 wait(4); 00069 00070 write(96);// Set wiper to 96/128 = 3/4 Rab 00071 leds[2]=1; 00072 wait(4); 00073 00074 // Decrement wiper 10 times 00075 for (int i=0; i<10; i++) { 00076 decrement(); 00077 } 00078 leds[3]=1; 00079 wait(4); 00080 00081 // Increment wiper 10 times 00082 for (int i=0; i<10; i++) { 00083 increment(); 00084 } 00085 00086 leds[3]=0; 00087 wait(4); 00088 00089 } 00090 00091 }
Generated on Tue Aug 2 2022 04:14:17 by
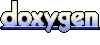