
Based on USBKeyboardMouse example. I added USB String Descriptor so mbed reports itself to host not only with VID & PID but also with name of manufacturer, product name, serial number, configuration number and interface name. These can be changed to matching Yours in USBhid.cpp file on lines 88 - 122.
usbdevice.h
00001 /* usbdevice.h */ 00002 /* Generic USB device */ 00003 /* Copyright (c) Phil Wright 2008 */ 00004 00005 #ifndef USBDEVICE_H 00006 #define USBDEVICE_H 00007 00008 #include "usbdc.h" 00009 00010 /* Endpoint packet sizes */ 00011 #define MAX_PACKET_SIZE_EP0 (64) 00012 00013 /* bmRequestType.dataTransferDirection */ 00014 #define HOST_TO_DEVICE (0) 00015 #define DEVICE_TO_HOST (1) 00016 00017 /* bmRequestType.Type*/ 00018 #define STANDARD_TYPE (0) 00019 #define CLASS_TYPE (1) 00020 #define VENDOR_TYPE (2) 00021 #define RESERVED_TYPE (3) 00022 00023 /* bmRequestType.Recipient */ 00024 #define DEVICE_RECIPIENT (0) 00025 #define INTERFACE_RECIPIENT (1) 00026 #define ENDPOINT_RECIPIENT (2) 00027 #define OTHER_RECIPIENT (3) 00028 00029 /* Descriptors */ 00030 #define DESCRIPTOR_TYPE(wValue) (wValue >> 8) 00031 #define DESCRIPTOR_INDEX(wValue) (wValue & 0xff) 00032 00033 /* Descriptor type */ 00034 #define DEVICE_DESCRIPTOR (1) 00035 #define CONFIGURATION_DESCRIPTOR (2) 00036 #define STRING_DESCRIPTOR (3) 00037 #define INTERFACE_DESCRIPTOR (4) 00038 #define ENDPOINT_DESCRIPTOR (5) 00039 00040 /*string offset*/ 00041 #define STRING_OFFSET_LANGID (0) 00042 #define STRING_OFFSET_IMANUFACTURER (1) 00043 #define STRING_OFFSET_IPRODUCT (2) 00044 #define STRING_OFFSET_ISERIAL (3) 00045 #define STRING_OFFSET_ICONFIGURATION (4) 00046 #define STRING_OFFSET_IINTERFACE (5) 00047 00048 typedef struct { 00049 struct { 00050 unsigned char dataTransferDirection; 00051 unsigned char Type; 00052 unsigned char Recipient; 00053 } bmRequestType; 00054 unsigned char bRequest; 00055 unsigned short wValue; 00056 unsigned short wIndex; 00057 unsigned short wLength; 00058 } SETUP_PACKET; 00059 00060 typedef struct { 00061 SETUP_PACKET setup; 00062 unsigned char *ptr; 00063 unsigned long remaining; 00064 unsigned char direction; 00065 bool zlp; 00066 } CONTROL_TRANSFER; 00067 00068 typedef enum {ATTACHED, POWERED, DEFAULT, ADDRESS, CONFIGURED} DEVICE_STATE; 00069 00070 typedef struct { 00071 DEVICE_STATE state; 00072 unsigned char configuration; 00073 bool suspended; 00074 } USB_DEVICE; 00075 00076 class usbdevice : public usbdc 00077 { 00078 public: 00079 usbdevice(); 00080 protected: 00081 virtual void endpointEventEP0Setup(void); 00082 virtual void endpointEventEP0In(void); 00083 virtual void endpointEventEP0Out(void); 00084 virtual bool requestSetup(void); 00085 virtual bool requestOut(void); 00086 virtual void deviceEventReset(void); 00087 virtual bool requestGetDescriptor(void); 00088 bool requestSetAddress(void); 00089 virtual bool requestSetConfiguration(void); 00090 virtual bool requestGetConfiguration(void); 00091 bool requestGetStatus(void); 00092 virtual bool requestSetInterface(void); 00093 virtual bool requestGetInterface(void); 00094 bool requestSetFeature(void); 00095 bool requestClearFeature(void); 00096 CONTROL_TRANSFER transfer; 00097 USB_DEVICE device; 00098 private: 00099 bool controlIn(void); 00100 bool controlOut(void); 00101 bool controlSetup(void); 00102 void decodeSetupPacket(unsigned char *data, SETUP_PACKET *packet); 00103 }; 00104 00105 #endif
Generated on Fri Jul 15 2022 16:43:53 by
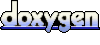