
Based on USBKeyboardMouse example. I added USB String Descriptor so mbed reports itself to host not only with VID & PID but also with name of manufacturer, product name, serial number, configuration number and interface name. These can be changed to matching Yours in USBhid.cpp file on lines 88 - 122.
USBMouse.h
00001 #include "usbhid.h" 00002 00003 #ifndef MBED_USBMOUSE_H 00004 #define MBED_USBMOUSE_H 00005 00006 /* Class: USBMouse 00007 * Emulate a USB Mouse HID device 00008 * 00009 * Example: 00010 * > #include "mbed.h" 00011 * > #include "USBMouse.h" 00012 * > 00013 * > USBMouse mouse; 00014 * > 00015 * > int main() { 00016 * > while(1) { 00017 * > mouse.move(10, 0); 00018 * > wait(2); 00019 * > } 00020 * > } 00021 */ 00022 class USBMouse : private usbhid { 00023 public: 00024 /* Constructor: USBMouse 00025 * Create a USB Mouse using the mbed USB Device interface 00026 */ 00027 USBMouse(); 00028 00029 /* Function: move 00030 * Move the mouse 00031 * 00032 * Variables: 00033 * x - Distance to move in x-axis 00034 * y - Distance to move in y-axis 00035 */ 00036 void move(int x, int y); 00037 00038 /* Function: scroll 00039 * Scroll the scroll wheel 00040 * 00041 * Variables: 00042 * z - Distance to scroll scroll wheel 00043 */ 00044 void scroll(int z); 00045 00046 /* Function: buttons 00047 * Set the state of the buttons 00048 * 00049 * Variables: 00050 * left - set the left button as down (1) or up (0) 00051 * middle - set the middle button as down (1) or up (0) 00052 * right - set the right button as down (1) or up (0) 00053 */ 00054 void buttons(int left, int middle, int right); 00055 00056 private: 00057 int _buttons; 00058 }; 00059 00060 #endif
Generated on Fri Jul 15 2022 16:43:53 by
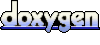