
Based on USBKeyboardMouse example. I added USB String Descriptor so mbed reports itself to host not only with VID & PID but also with name of manufacturer, product name, serial number, configuration number and interface name. These can be changed to matching Yours in USBhid.cpp file on lines 88 - 122.
USBMouse.cpp
00001 #include "USBMouse.h" 00002 00003 USBMouse::USBMouse() { 00004 _buttons = 0; 00005 } 00006 00007 void USBMouse::move(int x, int y) { 00008 while(x > 127) { 00009 mouse(127, 0, _buttons, 0); 00010 x = x - 127; 00011 } 00012 while(x < -128) { 00013 mouse(-128, 0, _buttons, 0); 00014 x = x + 128; 00015 } 00016 while(y > 127) { 00017 mouse(0, 127, _buttons, 0); 00018 y = y - 127; 00019 } 00020 while(y < -128) { 00021 mouse(0, -128, _buttons, 0); 00022 y = y + 128; 00023 } 00024 mouse(x, y, _buttons, 0); 00025 } 00026 00027 void USBMouse::scroll(int z) { 00028 while(z > 127) { 00029 mouse(0, 0, _buttons, 127); 00030 z = z - 127; 00031 } 00032 while(z < -128) { 00033 mouse(0, 0, _buttons, -128); 00034 z = z + 128; 00035 } 00036 mouse(0, 0, _buttons, z); 00037 } 00038 00039 void USBMouse::buttons(int left, int middle, int right) { 00040 int _buttons = 0; 00041 if(left) { 00042 _buttons |= MOUSE_L; 00043 } 00044 if(middle) { 00045 _buttons |= MOUSE_M; 00046 } 00047 if(right) { 00048 _buttons |= MOUSE_R; 00049 } 00050 mouse(0,0, _buttons, 0); 00051 }
Generated on Fri Jul 15 2022 16:43:53 by
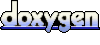