Fork of official USB device library just changed PID (Product ID) in constructor in USBMSD.h to be different for USBMSD_AT45_HelloWorld program
Dependents: USBMSD_AT45_HelloWorld
Fork of USBDevice by
USBSerial.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBSERIAL_H 00020 #define USBSERIAL_H 00021 00022 #include "USBCDC.h" 00023 #include "Stream.h" 00024 #include "CircBuffer.h" 00025 00026 00027 /** 00028 * USBSerial example 00029 * 00030 * @code 00031 * #include "mbed.h" 00032 * #include "USBSerial.h" 00033 * 00034 * //Virtual serial port over USB 00035 * USBSerial serial; 00036 * 00037 * int main(void) { 00038 * 00039 * while(1) 00040 * { 00041 * serial.printf("I am a virtual serial port\n"); 00042 * wait(1); 00043 * } 00044 * } 00045 * @endcode 00046 */ 00047 class USBSerial: public USBCDC, public Stream { 00048 public: 00049 00050 /** 00051 * Constructor 00052 * 00053 * @param vendor_id Your vendor_id (default: 0x1f00) 00054 * @param product_id Your product_id (default: 0x2012) 00055 * @param product_release Your preoduct_release (default: 0x0001) 00056 * 00057 */ 00058 USBSerial(uint16_t vendor_id = 0x1f00, uint16_t product_id = 0x2012, uint16_t product_release = 0x0001): USBCDC(vendor_id, product_id, product_release), buf(128){ }; 00059 00060 00061 /** 00062 * Send a character. You can use puts, printf. 00063 * 00064 * @param c character to be sent 00065 * @returns true if there is no error, false otherwise 00066 */ 00067 virtual int _putc(int c); 00068 00069 /** 00070 * Read a character: blocking 00071 * 00072 * @returns character read 00073 */ 00074 virtual int _getc(); 00075 00076 /** 00077 * Check the number of bytes available. 00078 * 00079 * @returns the number of bytes available 00080 */ 00081 uint8_t available(); 00082 00083 /** 00084 * Write a block of data. 00085 * 00086 * For more efficiency, a block of size 64 (maximum size of a bulk endpoint) has to be written. 00087 * 00088 * @param buf pointer on data which will be written 00089 * @param size size of the buffer. The maximum size of a block is limited by the size of the endpoint (64 bytes) 00090 * 00091 * @returns true if successfull 00092 */ 00093 bool writeBlock(uint8_t * buf, uint16_t size); 00094 00095 /** 00096 * Attach a member function to call when a packet is received. 00097 * 00098 * @param tptr pointer to the object to call the member function on 00099 * @param mptr pointer to the member function to be called 00100 */ 00101 template<typename T> 00102 void attach(T* tptr, void (T::*mptr)(void)) { 00103 if((mptr != NULL) && (tptr != NULL)) { 00104 rx.attach(tptr, mptr); 00105 } 00106 } 00107 00108 /** 00109 * Attach a callback called when a packet is received 00110 * 00111 * @param fptr function pointer 00112 */ 00113 void attach(void (*fn)(void)) { 00114 if(fn != NULL) { 00115 rx.attach(fn); 00116 } 00117 } 00118 00119 00120 protected: 00121 virtual bool EP2_OUT_callback(); 00122 00123 private: 00124 FunctionPointer rx; 00125 CircBuffer<uint8_t> buf; 00126 }; 00127 00128 #endif
Generated on Tue Jul 12 2022 21:32:44 by
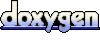