Fork of official USB device library just changed PID (Product ID) in constructor in USBMSD.h to be different for USBMSD_AT45_HelloWorld program
Dependents: USBMSD_AT45_HelloWorld
Fork of USBDevice by
USBMSD.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 00020 #ifndef USBMSD_H 00021 #define USBMSD_H 00022 00023 /* These headers are included for child class. */ 00024 #include "USBEndpoints.h" 00025 #include "USBDescriptor.h" 00026 #include "USBDevice_Types.h" 00027 00028 #include "USBDevice.h" 00029 00030 /** 00031 * USBMSD class: generic class in order to use all kinds of blocks storage chip 00032 * 00033 * Introduction 00034 * 00035 * The USBMSD implements the MSD protocol. It permits to access a memory chip (flash, sdcard,...) 00036 * from a computer over USB. But this class doesn't work standalone, you need to subclass this class 00037 * and define virtual functions which are called in USBMSD. 00038 * 00039 * How to use this class with your chip ? 00040 * 00041 * You have to inherit and define some pure virtual functions (mandatory step): 00042 * - virtual int disk_read(char * data, int block): function to read a block 00043 * - virtual int disk_write(const char * data, int block): function to write a block 00044 * - virtual int disk_initialize(): function to initialize the memory 00045 * - virtual int disk_sectors(): return the number of blocks 00046 * - virtual int disk_size(): return the memory size 00047 * - virtual int disk_status(): return the status of the storage chip (0: OK, 1: not initialized, 2: no medium in the drive, 4: write protection) 00048 * 00049 * All functions names are compatible with the fat filesystem library. So you can imagine using your own class with 00050 * USBMSD and the fat filesystem library in the same program. Just be careful because there are two different parts which 00051 * will access the sd card. You can do a master/slave system using the disk_status method. 00052 * 00053 * Once these functions defined, you can call connect() (at the end of the constructor of your class for instance) 00054 * of USBMSD to connect your mass storage device. connect() will first call disk_status() to test the status of the disk. 00055 * If disk_status() returns 1 (disk not initialized), then disk_initialize() is called. After this step, connect() will collect information 00056 * such as the number of blocks and the memory size. 00057 */ 00058 class USBMSD: public USBDevice { 00059 public: 00060 00061 /** 00062 * Constructor 00063 * 00064 * @param vendor_id Your vendor_id 00065 * @param product_id Your product_id 00066 * @param product_release Your preoduct_release 00067 */ 00068 USBMSD(uint16_t vendor_id = 0x0703, uint16_t product_id = 0x0404, uint16_t product_release = 0x0001); 00069 00070 /** 00071 * Connect the USB MSD device. Establish disk initialization before really connect the device. 00072 * 00073 * @returns true if successful 00074 */ 00075 bool connect(); 00076 00077 00078 protected: 00079 00080 /* 00081 * read a block on a storage chip 00082 * 00083 * @param data pointer where will be stored read data 00084 * @param block block number 00085 * @returns 0 if successful 00086 */ 00087 virtual int disk_read(char * data, int block) = 0; 00088 00089 /* 00090 * write a block on a storage chip 00091 * 00092 * @param data data to write 00093 * @param block block number 00094 * @returns 0 if successful 00095 */ 00096 virtual int disk_write(const char * data, int block) = 0; 00097 00098 /* 00099 * Disk initilization 00100 */ 00101 virtual int disk_initialize() = 0; 00102 00103 /* 00104 * Return the number of blocks 00105 * 00106 * @returns number of blocks 00107 */ 00108 virtual int disk_sectors() = 0; 00109 00110 /* 00111 * Return memory size 00112 * 00113 * @returns memory size 00114 */ 00115 virtual int disk_size() = 0; 00116 00117 00118 /* 00119 * To check the status of the storage chip 00120 * 00121 * @returns status: 0: OK, 1: disk not initialized, 2: no medium in the drive, 4: write protected 00122 */ 00123 virtual int disk_status() = 0; 00124 00125 /* 00126 * Get string product descriptor 00127 * 00128 * @returns pointer to the string product descriptor 00129 */ 00130 virtual uint8_t * stringIproductDesc(); 00131 00132 /* 00133 * Get string interface descriptor 00134 * 00135 * @returns pointer to the string interface descriptor 00136 */ 00137 virtual uint8_t * stringIinterfaceDesc(); 00138 00139 /* 00140 * Get configuration descriptor 00141 * 00142 * @returns pointer to the configuration descriptor 00143 */ 00144 virtual uint8_t * configurationDesc(); 00145 00146 /* 00147 * Callback called when a packet is received 00148 */ 00149 virtual bool EP2_OUT_callback(); 00150 00151 /* 00152 * Callback called when a packet has been sent 00153 */ 00154 virtual bool EP2_IN_callback(); 00155 00156 /* 00157 * Set configuration of device. Add endpoints 00158 */ 00159 virtual bool USBCallback_setConfiguration(uint8_t configuration); 00160 00161 /* 00162 * Callback called to process class specific requests 00163 */ 00164 virtual bool USBCallback_request(); 00165 00166 00167 private: 00168 00169 // MSC Bulk-only Stage 00170 enum Stage { 00171 READ_CBW, // wait a CBW 00172 ERROR, // error 00173 PROCESS_CBW, // process a CBW request 00174 SEND_CSW, // send a CSW 00175 WAIT_CSW, // wait that a CSW has been effectively sent 00176 }; 00177 00178 // Bulk-only CBW 00179 typedef __packed struct { 00180 uint32_t Signature; 00181 uint32_t Tag; 00182 uint32_t DataLength; 00183 uint8_t Flags; 00184 uint8_t LUN; 00185 uint8_t CBLength; 00186 uint8_t CB[16]; 00187 } CBW; 00188 00189 // Bulk-only CSW 00190 typedef __packed struct { 00191 uint32_t Signature; 00192 uint32_t Tag; 00193 uint32_t DataResidue; 00194 uint8_t Status; 00195 } CSW; 00196 00197 //state of the bulk-only state machine 00198 Stage stage; 00199 00200 // current CBW 00201 CBW cbw; 00202 00203 // CSW which will be sent 00204 CSW csw; 00205 00206 // addr where will be read or written data 00207 uint32_t addr; 00208 00209 // length of a reading or writing 00210 uint32_t length; 00211 00212 // memory OK (after a memoryVerify) 00213 bool memOK; 00214 00215 // cache in RAM before writing in memory. Useful also to read a block. 00216 uint8_t * page; 00217 00218 int BlockSize; 00219 int MemorySize; 00220 int BlockCount; 00221 00222 void CBWDecode(uint8_t * buf, uint16_t size); 00223 void sendCSW (void); 00224 bool inquiryRequest (void); 00225 bool write (uint8_t * buf, uint16_t size); 00226 bool readFormatCapacity(); 00227 bool readCapacity (void); 00228 bool infoTransfer (void); 00229 void memoryRead (void); 00230 bool modeSense6 (void); 00231 void testUnitReady (void); 00232 bool requestSense (void); 00233 void memoryVerify (uint8_t * buf, uint16_t size); 00234 void memoryWrite (uint8_t * buf, uint16_t size); 00235 void reset(); 00236 void fail(); 00237 }; 00238 00239 #endif
Generated on Tue Jul 12 2022 21:32:44 by
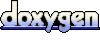