Fork of official USB device library just changed PID (Product ID) in constructor in USBMSD.h to be different for USBMSD_AT45_HelloWorld program
Dependents: USBMSD_AT45_HelloWorld
Fork of USBDevice by
USBDevice.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBDEVICE_H 00020 #define USBDEVICE_H 00021 00022 #include "mbed.h" 00023 #include "USBDevice_Types.h" 00024 #include "USBHAL.h" 00025 00026 class USBDevice: public USBHAL 00027 { 00028 public: 00029 USBDevice(uint16_t vendor_id, uint16_t product_id, uint16_t product_release); 00030 00031 /* 00032 * Check if the device is configured 00033 * 00034 * @returns true if configured, false otherwise 00035 */ 00036 bool configured(void); 00037 00038 /* 00039 * Connect a device 00040 */ 00041 void connect(void); 00042 00043 /* 00044 * Disconnect a device 00045 */ 00046 void disconnect(void); 00047 00048 /* 00049 * Add an endpoint 00050 * 00051 * @param endpoint endpoint which will be added 00052 * @param maxPacket Maximum size of a packet which can be sent for this endpoint 00053 * @returns true if successful, false otherwise 00054 */ 00055 bool addEndpoint(uint8_t endpoint, uint32_t maxPacket); 00056 00057 /* 00058 * Start a reading on a certain endpoint. 00059 * You can access the result of the reading by USBDevice_read 00060 * 00061 * @param endpoint endpoint which will be read 00062 * @param maxSize the maximum length that can be read 00063 * @return true if successful 00064 */ 00065 bool readStart(uint8_t endpoint, uint32_t maxSize); 00066 00067 /* 00068 * Read a certain endpoint. Before calling this function, USBUSBDevice_readStart 00069 * must be called. 00070 * 00071 * Warning: blocking 00072 * 00073 * @param endpoint endpoint which will be read 00074 * @param buffer buffer will be filled with the data received 00075 * @param size the number of bytes read will be stored in *size 00076 * @param maxSize the maximum length that can be read 00077 * @returns true if successful 00078 */ 00079 bool readEP(uint8_t endpoint, uint8_t * buffer, uint32_t * size, uint32_t maxSize); 00080 00081 /* 00082 * Read a certain endpoint. 00083 * 00084 * Warning: non blocking 00085 * 00086 * @param endpoint endpoint which will be read 00087 * @param buffer buffer will be filled with the data received (if data are available) 00088 * @param size the number of bytes read will be stored in *size 00089 * @param maxSize the maximum length that can be read 00090 * @returns true if successful 00091 */ 00092 bool readEP_NB(uint8_t endpoint, uint8_t * buffer, uint32_t * size, uint32_t maxSize); 00093 00094 /* 00095 * Write a certain endpoint. 00096 * 00097 * Warning: blocking 00098 * 00099 * @param endpoint endpoint to write 00100 * @param buffer data contained in buffer will be write 00101 * @param size the number of bytes to write 00102 * @param maxSize the maximum length that can be written on this endpoint 00103 */ 00104 bool write(uint8_t endpoint, uint8_t * buffer, uint32_t size, uint32_t maxSize); 00105 00106 00107 /* 00108 * Write a certain endpoint. 00109 * 00110 * Warning: non blocking 00111 * 00112 * @param endpoint endpoint to write 00113 * @param buffer data contained in buffer will be write 00114 * @param size the number of bytes to write 00115 * @param maxSize the maximum length that can be written on this endpoint 00116 */ 00117 bool writeNB(uint8_t endpoint, uint8_t * buffer, uint32_t size, uint32_t maxSize); 00118 00119 00120 /* 00121 * Called by USBDevice layer on bus reset. Warning: Called in ISR context 00122 * 00123 * May be used to reset state 00124 */ 00125 virtual void USBCallback_busReset(void) {}; 00126 00127 /* 00128 * Called by USBDevice on Endpoint0 request. Warning: Called in ISR context 00129 * This is used to handle extensions to standard requests 00130 * and class specific requests 00131 * 00132 * @returns true if class handles this request 00133 */ 00134 virtual bool USBCallback_request() { return false; }; 00135 00136 /* 00137 * Called by USBDevice on Endpoint0 request completion 00138 * if the 'notify' flag has been set to true. Warning: Called in ISR context 00139 * 00140 * In this case it is used to indicate that a HID report has 00141 * been received from the host on endpoint 0 00142 * 00143 * @param buf buffer received on endpoint 0 00144 * @param length length of this buffer 00145 */ 00146 virtual void USBCallback_requestCompleted(uint8_t * buf, uint32_t length) {}; 00147 00148 /* 00149 * Called by USBDevice layer. Set configuration of the device. 00150 * For instance, you can add all endpoints that you need on this function. 00151 * 00152 * @param configuration Number of the configuration 00153 */ 00154 virtual bool USBCallback_setConfiguration(uint8_t configuration) { return false; }; 00155 00156 /* 00157 * Called by USBDevice layer. Set interface/alternate of the device. 00158 * 00159 * @param interface Number of the interface to be configured 00160 * @param alternate Number of the alternate to be configured 00161 * @returns true if class handles this request 00162 */ 00163 virtual bool USBCallback_setInterface(uint16_t interface, uint8_t alternate) { return false; }; 00164 00165 /* 00166 * Get device descriptor. Warning: this method has to store the length of the report descriptor in reportLength. 00167 * 00168 * @returns pointer to the device descriptor 00169 */ 00170 virtual uint8_t * deviceDesc(); 00171 00172 /* 00173 * Get configuration descriptor 00174 * 00175 * @returns pointer to the configuration descriptor 00176 */ 00177 virtual uint8_t * configurationDesc(){return NULL;}; 00178 00179 /* 00180 * Get string lang id descriptor 00181 * 00182 * @return pointer to the string lang id descriptor 00183 */ 00184 virtual uint8_t * stringLangidDesc(); 00185 00186 /* 00187 * Get string manufacturer descriptor 00188 * 00189 * @returns pointer to the string manufacturer descriptor 00190 */ 00191 virtual uint8_t * stringImanufacturerDesc(); 00192 00193 /* 00194 * Get string product descriptor 00195 * 00196 * @returns pointer to the string product descriptor 00197 */ 00198 virtual uint8_t * stringIproductDesc(); 00199 00200 /* 00201 * Get string serial descriptor 00202 * 00203 * @returns pointer to the string serial descriptor 00204 */ 00205 virtual uint8_t * stringIserialDesc(); 00206 00207 /* 00208 * Get string configuration descriptor 00209 * 00210 * @returns pointer to the string configuration descriptor 00211 */ 00212 virtual uint8_t * stringIConfigurationDesc(); 00213 00214 /* 00215 * Get string interface descriptor 00216 * 00217 * @returns pointer to the string interface descriptor 00218 */ 00219 virtual uint8_t * stringIinterfaceDesc(); 00220 00221 /* 00222 * Get the length of the report descriptor 00223 * 00224 * @returns length of the report descriptor 00225 */ 00226 virtual uint16_t reportDescLength() { return 0; }; 00227 00228 00229 00230 protected: 00231 virtual void busReset(void); 00232 virtual void EP0setupCallback(void); 00233 virtual void EP0out(void); 00234 virtual void EP0in(void); 00235 virtual void connectStateChanged(unsigned int connected); 00236 virtual void suspendStateChanged(unsigned int suspended); 00237 uint8_t * findDescriptor(uint8_t descriptorType); 00238 CONTROL_TRANSFER * getTransferPtr(void); 00239 00240 uint16_t VENDOR_ID; 00241 uint16_t PRODUCT_ID; 00242 uint16_t PRODUCT_RELEASE; 00243 00244 private: 00245 bool addRateFeedbackEndpoint(uint8_t endpoint, uint32_t maxPacket); 00246 bool requestGetDescriptor(void); 00247 bool controlOut(void); 00248 bool controlIn(void); 00249 bool requestSetAddress(void); 00250 bool requestSetConfiguration(void); 00251 bool requestSetFeature(void); 00252 bool requestClearFeature(void); 00253 bool requestGetStatus(void); 00254 bool requestSetup(void); 00255 bool controlSetup(void); 00256 void decodeSetupPacket(uint8_t *data, SETUP_PACKET *packet); 00257 bool requestGetConfiguration(void); 00258 bool requestGetInterface(void); 00259 bool requestSetInterface(void); 00260 00261 CONTROL_TRANSFER transfer; 00262 USB_DEVICE device; 00263 00264 uint16_t currentInterface; 00265 uint8_t currentAlternate; 00266 }; 00267 00268 00269 #endif
Generated on Tue Jul 12 2022 21:32:43 by
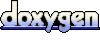