
Microduino的cube小车。
Embed:
(wiki syntax)
Show/hide line numbers
Microduino_Protocol_HardSer.cpp
00001 #include "Microduino_Protocol_HardSer.h" 00002 00003 //Timer _timer; 00004 extern Timer g_cubeTimer; 00005 uint8_t getChecksum(uint8_t length, uint8_t cmd, uint8_t mydata[]) 00006 { 00007 //三个参数分别为: 数据长度 , 指令代码 , 实际数据数组 00008 uint8_t checksum = 0; 00009 checksum ^= (length & 0xFF); 00010 checksum ^= (cmd & 0xFF); 00011 for (int i = 0; i < length; i++) checksum ^= (mydata[i] & 0xFF); 00012 return checksum; 00013 } 00014 00015 /* Protocol::Protocol(PRO_PORT *ser , byte _channel) { 00016 // common_init(); // Set everything to common state, then... 00017 this->channel = _channel; 00018 this->num = 0; 00019 this->sta = false; 00020 this->error = false; 00021 P_Serial = ser; // ...override P_Serial with value passed. 00022 } */ 00023 00024 void Protocol::begin(uint16_t _baud) 00025 { 00026 //_timer.start(); 00027 //P_Serial->begin(_baud); 00028 P_Serial->baud(_baud); 00029 //delay(20); 00030 //wait_ms(20); 00031 } 00032 00033 bool Protocol::available(bool _sta) 00034 { 00035 //if (P_Serial->available() > 0) { 00036 if (P_Serial->readable() > 0) { 00037 if (_sta) { 00038 this->inCache = this->inChar; 00039 //this->inChar = P_Serial->read(); 00040 this->inChar = P_Serial->getc(); 00041 this->buffer[num] = this->inChar; 00042 00043 if (this->num > BUFFER_MAX - 1) { 00044 this->num = 0; 00045 return false; 00046 } else { 00047 this->num++; 00048 } 00049 } 00050 return true; 00051 } 00052 return false; 00053 } 00054 00055 uint8_t Protocol::parse(uint16_t* _data, bool _mod) 00056 { 00057 if (available(!_mod)) { 00058 //time = millis(); 00059 //time = _timer.read_ms(); 00060 time = g_cubeTimer.read_ms(); 00061 do { 00062 if (this->sta) { 00063 this->sta = false; 00064 this->num = 0; 00065 if (this->inChar == this->channel) { 00066 this->error = false; 00067 if (!_mod) { 00068 return P_BUSY; 00069 } 00070 } else { 00071 this->error = true; 00072 return P_ERROR; 00073 } 00074 } 00075 00076 if (this->inChar == 0xBB && this->inCache == 0xAA) { 00077 this->sta = true; 00078 if (!_mod) { 00079 return P_BUSY; 00080 } 00081 } 00082 00083 if (this->num == (CHANNEL_NUM * 2 + 1) && !this->error) { 00084 this->inCache = this->buffer[CHANNEL_NUM * 2]; 00085 this->buffer[CHANNEL_NUM * 2] = NULL; 00086 this->inChar = getChecksum(CHANNEL_NUM * 2, 200, this->buffer); 00087 00088 this->num = 0; 00089 if (!this->error && this->inCache == this->inChar) { 00090 for (uint8_t a = 0; a < CHANNEL_NUM; a++) { 00091 _data[a] = ((uint16_t)(this->buffer[a * 2])) | ((uint16_t)this->buffer[a * 2 + 1] << 8); 00092 } 00093 return P_FINE; 00094 } else { 00095 return P_ERROR; 00096 } 00097 } else if (!_mod) { 00098 return P_BUSY; 00099 } 00100 } while (_mod && (available(true) && g_cubeTimer.read_ms() - time < 100)); 00101 00102 if (_mod) { 00103 return P_TIMEOUT; 00104 } 00105 } else { 00106 return P_NONE; 00107 } 00108 }
Generated on Tue Jul 12 2022 20:44:32 by
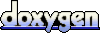