最新revisionだとなんかerrorになるので、暫定的に rev 111にrevert。ごめんなさい。こういうときどういうふうにcommitすればいいのか分からなかったので。
Fork of BLE_API by
UUID.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #ifndef __UUID_H__ 00019 #define __UUID_H__ 00020 00021 #include "blecommon.h" 00022 00023 const unsigned LENGTH_OF_LONG_UUID = 16; 00024 typedef uint16_t ShortUUID_t; 00025 typedef uint8_t LongUUID_t[LENGTH_OF_LONG_UUID]; 00026 00027 class UUID 00028 { 00029 public: 00030 enum { 00031 UUID_TYPE_SHORT = 0, // Short BLE UUID 00032 UUID_TYPE_LONG = 1 // Full 128-bit UUID 00033 }; 00034 00035 public: 00036 UUID(const LongUUID_t); 00037 UUID(ShortUUID_t); 00038 virtual ~UUID(void); 00039 00040 public: 00041 uint8_t shortOrLong(void) const { 00042 return type; 00043 } 00044 const uint8_t* getBaseUUID(void) const { 00045 return baseUUID; 00046 } 00047 ShortUUID_t getShortUUID(void) const { 00048 return shortUUID; 00049 } 00050 00051 private: 00052 uint8_t type; // UUID_TYPE_SHORT or UUID_TYPE_LONG 00053 LongUUID_t baseUUID; /* the base of the long UUID (if 00054 * used). Note: bytes 12 and 13 (counting from LSB) 00055 * are zeroed out to allow comparison with other long 00056 * UUIDs which differ only in the 16-bit relative 00057 * part.*/ 00058 ShortUUID_t shortUUID; // 16 bit uuid (byte 2-3 using with base) 00059 }; 00060 00061 #endif // ifndef __UUID_H__
Generated on Tue Jul 12 2022 20:47:07 by
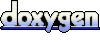