最新revisionだとなんかerrorになるので、暫定的に rev 111にrevert。ごめんなさい。こういうときどういうふうにcommitすればいいのか分からなかったので。
Fork of BLE_API by
UUID.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #include <stdio.h> 00019 #include <string.h> 00020 00021 #include "UUID.h" 00022 00023 /**************************************************************************/ 00024 /*! 00025 @brief Creates a new 128-bit UUID 00026 00027 @note The UUID is a unique 128-bit (16 byte) ID used to identify 00028 different service or characteristics on the BLE device. 00029 00030 @note When creating a UUID, the constructor will check if all bytes 00031 except bytes 2/3 are equal to 0. If only bytes 2/3 have a 00032 value, the UUID will be treated as a short/BLE UUID, and the 00033 .type field will be set to UUID::UUID_TYPE_SHORT. If any 00034 of the bytes outside byte 2/3 have a non-zero value, the UUID 00035 will be considered a 128-bit ID, and .type will be assigned 00036 as UUID::UUID_TYPE_LONG. 00037 00038 @param[in] uuid_base 00039 The 128-bit (16-byte) UUID value. For 128-bit values, 00040 assign all 16 bytes. For 16-bit values, assign the 00041 16-bits to byte 2 and 3, and leave the rest of the bytes 00042 as 0. 00043 00044 @section EXAMPLE 00045 00046 @code 00047 00048 // Create a short UUID (0x180F) 00049 uint8_t shortID[16] = { 0, 0, 0x0F, 0x18, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; 00050 UUID ble_uuid = UUID(shortID); 00051 // ble_uuid.type = UUID_TYPE_SHORT 00052 // ble_uuid.value = 0x180F 00053 00054 // Creeate a long UUID 00055 uint8_t longID[16] = { 0x00, 0x11, 0x22, 0x33, 00056 0x44, 0x55, 0x66, 0x77, 00057 0x88, 0x99, 0xAA, 0xBB, 00058 0xCC, 0xDD, 0xEE, 0xFF }; 00059 UUID custom_uuid = UUID(longID); 00060 // custom_uuid.type = UUID_TYPE_LONG 00061 // custom_uuid.value = 0x3322 00062 // custom_uuid.base = 00 11 22 33 44 55 66 77 88 99 AA BB CC DD EE FF 00063 00064 @endcode 00065 */ 00066 /**************************************************************************/ 00067 UUID::UUID(const LongUUID_t longUUID) : type(UUID_TYPE_SHORT), baseUUID(), shortUUID(0) 00068 { 00069 memcpy(baseUUID, longUUID, LENGTH_OF_LONG_UUID); 00070 shortUUID = (uint16_t)((longUUID[2] << 8) | (longUUID[3])); 00071 00072 /* Check if this is a short of a long UUID */ 00073 unsigned index; 00074 for (index = 0; index < LENGTH_OF_LONG_UUID; index++) { 00075 if ((index == 2) || (index == 3)) { 00076 continue; /* we should not consider bytes 2 and 3 because that's 00077 * where the 16-bit relative UUID is placed. */ 00078 } 00079 00080 if (baseUUID[index] != 0) { 00081 type = UUID_TYPE_LONG; 00082 00083 /* zero out the 16-bit part in the base; this will help equate long 00084 * UUIDs when they differ only in this 16-bit relative part.*/ 00085 baseUUID[2] = 0; 00086 baseUUID[3] = 0; 00087 00088 return; 00089 } 00090 } 00091 } 00092 00093 /**************************************************************************/ 00094 /*! 00095 @brief Creates a short (16-bit) UUID 00096 00097 @param[in] ble_uuid 00098 The 16-bit BLE UUID value. 00099 */ 00100 /**************************************************************************/ 00101 UUID::UUID(ShortUUID_t shortUUID) : type(UUID_TYPE_SHORT), baseUUID(), shortUUID(shortUUID) 00102 { 00103 /* empty */ 00104 } 00105 00106 /**************************************************************************/ 00107 /*! 00108 @brief UUID destructor 00109 */ 00110 /**************************************************************************/ 00111 UUID::~UUID(void) 00112 { 00113 }
Generated on Tue Jul 12 2022 20:47:07 by
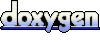