最新revisionだとなんかerrorになるので、暫定的に rev 111にrevert。ごめんなさい。こういうときどういうふうにcommitすればいいのか分からなかったので。
Fork of BLE_API by
GattServer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GATT_SERVER_H__ 00018 #define __GATT_SERVER_H__ 00019 00020 #include "mbed.h" 00021 #include "blecommon.h" 00022 #include "GattService.h" 00023 #include "GattServerEvents.h" 00024 00025 /**************************************************************************/ 00026 /*! 00027 \brief 00028 The base class used to abstract GATT Server functionality to a specific 00029 radio transceiver, SOC or BLE Stack. 00030 */ 00031 /**************************************************************************/ 00032 class GattServer 00033 { 00034 public: 00035 /* These functions must be defined in the sub-class */ 00036 virtual ble_error_t addService(GattService &) = 0; 00037 virtual ble_error_t readValue(uint16_t handle, uint8_t buffer[], uint16_t *const lengthP) = 0; 00038 virtual ble_error_t updateValue(uint16_t, uint8_t[], uint16_t, bool localOnly = false) = 0; 00039 virtual ble_error_t setDeviceName(const uint8_t *deviceName) = 0; 00040 virtual ble_error_t getDeviceName(uint8_t *deviceName, unsigned *lengthP) = 0; 00041 virtual ble_error_t setAppearance(uint16_t appearance) = 0; 00042 virtual ble_error_t getAppearance(uint16_t *appearanceP) = 0; 00043 00044 // ToDo: For updateValue, check the CCCD to see if the value we are 00045 // updating has the notify or indicate bits sent, and if BOTH are set 00046 // be sure to call sd_ble_gatts_hvx() twice with notify then indicate! 00047 // Strange use case, but valid and must be covered! 00048 00049 /* Event callback handlers. */ 00050 typedef void (*EventCallback_t)(uint16_t attributeHandle); 00051 typedef void (*ServerEventCallback_t)(void); /* independent of any particular attribute */ 00052 void setOnDataSent(ServerEventCallback_t callback) { 00053 onDataSent = callback; 00054 } 00055 void setOnDataWritten(EventCallback_t callback) { 00056 onDataWritten = callback; 00057 } 00058 void setOnUpdatesEnabled(EventCallback_t callback) { 00059 onUpdatesEnabled = callback; 00060 } 00061 void setOnUpdatesDisabled(EventCallback_t callback) { 00062 onUpdatesDisabled = callback; 00063 } 00064 void setOnConfirmationReceived(EventCallback_t callback) { 00065 onConfirmationReceived = callback; 00066 } 00067 00068 void handleEvent(GattServerEvents::gattEvent_e type, uint16_t charHandle) { 00069 switch (type) { 00070 case GattServerEvents::GATT_EVENT_DATA_WRITTEN: 00071 if (onDataWritten) { 00072 onDataWritten(charHandle); 00073 } 00074 break; 00075 case GattServerEvents::GATT_EVENT_UPDATES_ENABLED: 00076 if (onUpdatesEnabled) { 00077 onUpdatesEnabled(charHandle); 00078 } 00079 break; 00080 case GattServerEvents::GATT_EVENT_UPDATES_DISABLED: 00081 if (onUpdatesDisabled) { 00082 onUpdatesDisabled(charHandle); 00083 } 00084 break; 00085 case GattServerEvents::GATT_EVENT_CONFIRMATION_RECEIVED: 00086 if (onConfirmationReceived) { 00087 onConfirmationReceived(charHandle); 00088 } 00089 break; 00090 } 00091 } 00092 00093 void handleEvent(GattServerEvents::gattEvent_e type) { 00094 switch (type) { 00095 case GattServerEvents::GATT_EVENT_DATA_SENT: 00096 if (onDataSent) { 00097 onDataSent(); 00098 } 00099 break; 00100 default: 00101 break; 00102 } 00103 } 00104 00105 protected: 00106 GattServer() : serviceCount(0), characteristicCount(0), onDataSent(NULL), onDataWritten(NULL), onUpdatesEnabled(NULL), onUpdatesDisabled(NULL), onConfirmationReceived(NULL) { 00107 /* empty */ 00108 } 00109 00110 protected: 00111 uint8_t serviceCount; 00112 uint8_t characteristicCount; 00113 00114 private: 00115 ServerEventCallback_t onDataSent; 00116 EventCallback_t onDataWritten; 00117 EventCallback_t onUpdatesEnabled; 00118 EventCallback_t onUpdatesDisabled; 00119 EventCallback_t onConfirmationReceived; 00120 }; 00121 00122 #endif // ifndef __GATT_SERVER_H__
Generated on Tue Jul 12 2022 20:47:07 by
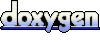