
May 2021 test
Dependencies: sx128x sx12xx_hal
main.cpp
00001 #include "mbed.h" 00002 #include "radio.h" 00003 00004 #include "mbed-trace/mbed_trace.h" 00005 #define TRACE_GROUP "slav" 00006 00007 static Mutex serialOutMutex; 00008 static void serial_out_mutex_wait() 00009 { 00010 serialOutMutex.lock(); 00011 } 00012 00013 static void serial_out_mutex_release() 00014 { 00015 osStatus s = serialOutMutex.unlock(); 00016 MBED_ASSERT(s == osOK); 00017 } 00018 00019 DigitalOut myled(LED1); 00020 00021 #if defined(SX127x_H) || defined(SX126x_H) 00022 #define BW_KHZ 500 00023 #define SPREADING_FACTOR 11 00024 #define CF_HZ 910800000 00025 #elif defined(SX128x_H) 00026 #define BW_KHZ 200 00027 #define SPREADING_FACTOR 11 00028 #define CF_HZ 2487000000 00029 #endif 00030 00031 #ifdef TARGET_DISCO_L072CZ_LRWAN1 00032 DigitalOut pinCommandExecute(PB_2); 00033 DigitalOut pin0(PB_5); 00034 DigitalOut pin1(PB_6); 00035 DigitalOut pin2(PB_8); 00036 DigitalOut pin3(PB_9); 00037 DigitalOut pin4(PB_12); 00038 DigitalOut pin5(PB_13); 00039 DigitalOut pin6(PB_14); 00040 DigitalOut pin7(PB_15); 00041 #else 00042 DigitalOut pin4(PC_3); 00043 DigitalOut pin5(PC_2); 00044 DigitalOut pin6(PC_6); 00045 DigitalOut pin7(PC_8); 00046 #endif /* !TARGET_DISCO_L072CZ_LRWAN1 */ 00047 00048 Timeout timeoutOutputDelay; 00049 Timeout timeoutCommandExecuteSignal; 00050 static uint8_t command = 0; 00051 00052 #define PIN_ASSERT_us 500000 00053 #define COMMAND_EXECUTE_SIGNAL_PERIOD 200000 00054 00055 00056 /**********************************************************************/ 00057 00058 void alarm_pin_clr() 00059 { 00060 00061 } 00062 00063 00064 static bool commandWrittenFlag = false; 00065 static bool commandAssertedFlag = false; 00066 00067 void onCommandExecuteSignalTimeout(void) 00068 { 00069 pinCommandExecute = false; 00070 commandAssertedFlag = true; 00071 } 00072 00073 void writeCommandToDigitalOuts(void) 00074 { 00075 pin0 = command & 0b00000001; 00076 pin1 = command & 0b00000010; 00077 pin2 = command & 0b00000100; 00078 pin3 = command & 0b00001000; 00079 pin4 = command & 0b00010000; 00080 pin5 = command & 0b00100000; 00081 pin6 = command & 0b01000000; 00082 pin7 = command & 0b10000000; 00083 pinCommandExecute = true; 00084 commandWrittenFlag = true; 00085 timeoutCommandExecuteSignal.attach_us(onCommandExecuteSignalTimeout, COMMAND_EXECUTE_SIGNAL_PERIOD); 00086 } 00087 00088 static uint16_t crc_ccitt( uint8_t *buffer, uint16_t length ) 00089 { 00090 tr_debug(__FUNCTION__); 00091 const uint16_t polynom = 0x1021; // The CRC calculation follows CCITT 00092 uint16_t crc = 0x0000; // CRC initial value 00093 00094 if( buffer == NULL ) 00095 { 00096 tr_debug("NULL Buffer"); 00097 return 0; 00098 } 00099 00100 for(uint16_t i = 0; i < length; ++i) 00101 { 00102 crc ^= ( uint16_t ) buffer[i] << 8; 00103 for( uint16_t j = 0; j < 8; ++j ) 00104 { 00105 crc = ( crc & 0x8000 ) ? ( crc << 1 ) ^ polynom : ( crc << 1 ); 00106 } 00107 } 00108 return crc; 00109 } 00110 00111 void get_alarm() 00112 { 00113 tr_debug(__FUNCTION__); 00114 00115 uint16_t rx_crc, crc = crc_ccitt(Radio::radio.rx_buf, 5); 00116 rx_crc = Radio::radio.rx_buf[5]; 00117 rx_crc <<= 8; 00118 rx_crc += Radio::radio.rx_buf[6]; 00119 //tr_debug("%u) crc rx:%04x, calc:%04x\r\n", lora.RegRxNbBytes, rx_crc, crc); 00120 00121 if (crc != rx_crc) 00122 { 00123 tr_debug("crc fail %04x, %04x\r\n", rx_crc, crc); 00124 return; 00125 } 00126 00127 CriticalSectionLock::enable(); 00128 command = Radio::radio.rx_buf[0]; 00129 CriticalSectionLock::disable(); 00130 00131 unsigned delay; 00132 delay = Radio::radio.rx_buf[1]; 00133 delay <<= 8; 00134 delay += Radio::radio.rx_buf[2]; 00135 delay <<= 8; 00136 delay += Radio::radio.rx_buf[3]; 00137 delay <<= 8; 00138 delay += Radio::radio.rx_buf[4]; 00139 00140 timeoutOutputDelay.attach_us(writeCommandToDigitalOuts, delay); 00141 tr_debug("output delay:%u\r\n", delay); 00142 } 00143 00144 void txDoneCB() 00145 { 00146 } 00147 00148 void rxDoneCB(uint8_t size, float Rssi, float Snr) 00149 { 00150 get_alarm(); 00151 tr_debug("%.1fdBm snr:%.1fdB ", Rssi, Snr); 00152 } 00153 00154 const RadioEvents_t rev = 00155 { 00156 /* Dio0_top_half */ NULL, 00157 /* TxDone_topHalf */ NULL, 00158 /* TxDone_botHalf */ txDoneCB, 00159 /* TxTimeout */ NULL, 00160 /* RxDone */ rxDoneCB, 00161 /* RxTimeout */ NULL, 00162 /* RxError */ NULL, 00163 /* FhssChangeChannel */NULL, 00164 /* CadDone */ NULL 00165 }; 00166 00167 00168 int main() 00169 { 00170 mbed_trace_mutex_wait_function_set( serial_out_mutex_wait ); 00171 mbed_trace_mutex_release_function_set( serial_out_mutex_release ); 00172 mbed_trace_init(); 00173 tr_debug(__FUNCTION__); 00174 00175 Radio::Init(&rev); 00176 Radio::Standby(); 00177 Radio::LoRaModemConfig(BW_KHZ, SPREADING_FACTOR, 1); 00178 Radio::LoRaPacketConfig(8, false, true, false); // preambleLen, fixLen, crcOn, invIQ 00179 Radio::SetChannel(CF_HZ); 00180 Radio::Rx(0); 00181 00182 while(true) 00183 { 00184 Radio::service(); 00185 if(commandWrittenFlag) 00186 { 00187 tr_debug("Command Written: %d", command); 00188 CriticalSectionLock::enable(); 00189 commandWrittenFlag = false; 00190 CriticalSectionLock::disable(); 00191 } 00192 if(commandAssertedFlag) 00193 { 00194 tr_debug("Command Asserted: %d", command); 00195 CriticalSectionLock::enable(); 00196 commandAssertedFlag = false; 00197 CriticalSectionLock::disable(); 00198 } 00199 } 00200 }
Generated on Sun Jul 17 2022 23:19:28 by
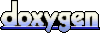