
This is a basic MBED driver for the AD9850 digital sine wave synthesizer. It uses a hacked SPI interface to drive the device,
main.cpp
00001 // MBED driver for AD9850 digital synthesizer using hacked SPI interface 00002 // Liam Goudge Sept 2013 00003 00004 #include "mbed.h" 00005 00006 SPI device (p5,p6,p7); // MOSI, MISO (not used), SCLK 00007 DigitalOut CS(p8); // Use pin 8 as a fake Chip select 00008 DigitalOut ADReset(p15); // Pin 15 is reset line for AD9850 00009 00010 Serial pc(USBTX, USBRX); // tx, rx for debug terminal 00011 00012 int reverseBits (int source) 00013 { 00014 // Unfortunately need to invert bit order of the desired frequency setting since MBED only allows for MSB first from SPI. We need LSB first for AD9850 00015 int mask=0;; 00016 int i=0; 00017 int target=0; 00018 int bitTarget=0x80000000; // Hard-wired for 32-bit inversion 00019 00020 for (i=0;i<32;i++) { // ditto 00021 mask=1<<i; 00022 bitTarget=1<<(31-i); // ditto 00023 00024 if (source & mask) 00025 target=target | bitTarget; 00026 } 00027 return target; 00028 } 00029 00030 void writeSPI(int frq, int phase) 00031 { 00032 // Send the 40-bit packet. MBED only allows max 16-bit packets so we send 40-bits as 16, 16, 8 00033 device.format(16,0); // 16-bits per packet, mode 0 (CPOL=0 CPHA=0) 00034 device.frequency(1000000); //SPI clock set to 1MHz 00035 00036 wait_ms(5); 00037 00038 // First do chip select. Need to use a GPIO to fake the chip select since MBED doesn't allow to set positive logic CS signal 00039 CS=1; // assert chip select (a.k.a FQ_UD frequency update input to AD9850) 00040 wait_ms(5); 00041 CS=0; 00042 00043 device.write(frq>>16); // Write upper 16-bits first starting with bit 31 00044 device.write(frq); // Now lower 16 bits starting with bit 15 00045 00046 device.format(8,0); // Need to reset to 8-bit data since MBED only allows max 16-bit packets. For 40-bits we do 16, 16, 8 00047 device.write(phase); // This is phase and factory settings byte 00048 00049 // Now pulse FQ_UD again to signal the end of the packet 00050 CS=0; 00051 wait_ms(5); 00052 CS=1; 00053 wait_ms(5); 00054 CS=0; 00055 } 00056 00057 00058 int main() 00059 { 00060 int targetFrq=0x147AE148; // This is desired sine wave frequency for the AD9850, here set to 10MHz 00061 int increment=0x346DC6; // This is a 100KHz frequency increment 00062 00063 // Reset the AD9850. Active high logic. Minimum reset period 5 clock cycles (5/125MHz) 00064 ADReset=0; 00065 wait_ms(5); 00066 ADReset=1; 00067 wait_ms(5); 00068 ADReset=0; 00069 00070 while(1) 00071 { 00072 00073 while (targetFrq<0x28F5C28F) // up to 20MHz 00074 { 00075 writeSPI(reverseBits(targetFrq),0); // Don't use phase so set to zero. 00076 targetFrq=targetFrq+increment; 00077 //wait_ms(100); 00078 } 00079 00080 while (targetFrq>0x147AE148) // down to 10MHz 00081 { 00082 writeSPI(reverseBits(targetFrq),0); 00083 targetFrq=targetFrq-increment; 00084 //wait_ms(100); 00085 } 00086 00087 } 00088 00089 00090 }
Generated on Wed Jul 13 2022 11:37:02 by
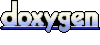