
Communication program for the chrobotics UM6 9-DOF IMU AHRS.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" // MBED LIBRARY 00002 #include "MODSERIAL.h" // MBED BUFFERED SERIAL 00003 00004 #include "UM6_usart.h" // UM6 USART HEADER 00005 #include "UM6_config.h" // UM6 CONFIG HEADER 00006 00007 ///////////////////////////////////////////////////////////////////////////////////////////// 00008 // SETUP (ASSIGN) SERIAL COMMUNICATION PINS ON MBED 00009 ///////////////////////////////////////////////////////////////////////////////////////////// 00010 MODSERIAL pc(USBTX, USBRX); // PC SERIAL OVER USB PORT ON MBED 00011 00012 //////////////////////////////////////////////////////////////////////////////////////////////// 00013 // SETUP (ASSIGN) MBED LED (1 thru 3) FOR VISUAL DEBUGGING ON MBED 00014 //////////////////////////////////////////////////////////////////////////////////////////////// 00015 DigitalOut pc_activity(LED1); // LED1 = PC SERIAL 00016 DigitalOut uart_activity(LED2); // LED2 = UM6 SERIAL 00017 00018 00019 void rxCallback(MODSERIAL_IRQ_INFO *q) { 00020 if (um6_uart.rxBufferGetCount() >= MAX_PACKET_DATA) { 00021 uart_activity = !uart_activity; // Lights LED when uart RxBuff has > 40 bytes 00022 Process_um6_packet(); 00023 } 00024 } 00025 00026 00027 int main() { 00028 00029 ///////////////////////////////////////////////////////////////////////////////////////////////////// 00030 // SET SERIAL UART BAUD RATES 00031 ///////////////////////////////////////////////////////////////////////////////////////////////////// 00032 00033 // set UM6 serial uart baud 9600 00034 um6_uart.baud(9600); 00035 pc.baud(9600); // pc baud for UM6 to pc interface 00036 00037 // attach interupt function to uart 00038 um6_uart.attach(&rxCallback, MODSERIAL::RxIrq); 00039 00040 int Roll_Counter = 0; 00041 00042 while (1) { 00043 Roll_Counter++; 00044 if (Roll_Counter > 5000000) { 00045 pc.printf("Gyro_Proc_X %f deg/s, ",data.Gyro_Proc_X); 00046 pc.printf("Gyro_Proc_Y %f deg/s, ",data.Gyro_Proc_Y); 00047 pc.printf("Gyro_Proc_Z %f deg/s, ",data.Gyro_Proc_Z); 00048 pc.printf("Roll %f deg, ",data.Roll); 00049 pc.printf("Pitch %f deg, ",data.Pitch); 00050 pc.printf("Yaw %f deg \r\n",data.Yaw); 00051 pc_activity = !pc_activity; // Lights LED when uart RxBuff has > 40 bytes 00052 Roll_Counter = 0; 00053 } 00054 00055 } // end while(1) loop 00056 00057 } // end main()
Generated on Tue Jul 12 2022 19:06:04 by
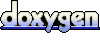