
2036 mbed lab4
Dependencies: 4DGL-uLCD-SE PinDetect
main.cpp
00001 #include "mbed.h" 00002 #define WHITE 0xFFFFFF 00003 #include <stdlib.h> 00004 #include <time.h> 00005 #include "PinDetect.h" 00006 #include "uLCD_4DGL.h" 00007 #include "TMP36.h" 00008 //#include "Die.h" 00009 #include "FarkleGame.h" 00010 #include "MMA8452.h" 00011 #include <cmath> 00012 #include "Speaker.h" 00013 #include <sstream> 00014 //------------------------Initial Declarations--------------------------------// 00015 Speaker mySpeaker(p26); 00016 PinDetect pb1(p25); 00017 PinDetect pb2(p24); 00018 PinDetect pb3(p23); 00019 Serial pc(USBTX,USBRX); 00020 MMA8452 acc(p28,p27,40000); 00021 uLCD_4DGL uLCD(p9, p10, p11); 00022 TMP36 myTMP36(p15); 00023 enum Inputs{buttonA,buttonB,buttonC,shake,none}; 00024 enum States{Initial,Display,Roll}; 00025 volatile Inputs input=none; 00026 volatile States currState=Initial; 00027 00028 void pb1_pressed(void){ 00029 input=buttonA; 00030 } 00031 void pb2_pressed(void){ 00032 input=buttonB; 00033 00034 } 00035 void pb3_pressed(void){ 00036 input=buttonC; 00037 } 00038 00039 void setupAccelerometer(){ 00040 acc.setBitDepth(MMA8452::BIT_DEPTH_12); 00041 acc.setDynamicRange(MMA8452::DYNAMIC_RANGE_4G); 00042 acc.setDataRate(MMA8452::RATE_100); 00043 } 00044 00045 00046 //---------------------------------------------------------------------------// 00047 int main() { 00048 int seedAssist; 00049 seedAssist = static_cast <int> (10000*myTMP36.read())%12344; 00050 srand(time(0)+seedAssist); 00051 //-------------------------// 00052 FarkleGame Farkle; 00053 00054 //-------------------------// 00055 pb1.mode(PullUp); 00056 wait(.01); 00057 pb1.attach_deasserted(&pb1_pressed); 00058 pb1.setSampleFrequency(); 00059 00060 pb2.mode(PullUp); 00061 wait(.01); 00062 pb2.attach_deasserted(&pb2_pressed); 00063 pb2.setSampleFrequency(); 00064 00065 pb3.mode(PullUp); 00066 wait(.01); 00067 pb3.attach_deasserted(&pb3_pressed); 00068 pb3.setSampleFrequency(); 00069 00070 //--------------------------// 00071 00072 int turnScore=0; 00073 00074 for( ; ; ){ 00075 switch(currState){ 00076 //----------------------------------------------------------------// 00077 case(Initial):{ 00078 uLCD.color(WHITE); 00079 Farkle.startGame(); 00080 //---------------Reading from accelerometer-----------------------// 00081 while(currState==Initial){ 00082 double x=0.0l; 00083 double y=0.0l; 00084 double z=0.0l; 00085 if (!acc.isXYZReady()) { 00086 wait(0.01); 00087 } else { 00088 acc.readXYZGravity(&x,&y,&z); 00089 } 00090 if ((fabs(x)>1.5)||(fabs(y)>1.5)) { 00091 input=shake; 00092 00093 00094 } 00095 //--------------Changing states based on input------------// 00096 if (input==buttonA){ 00097 currState=Initial; 00098 input=none; 00099 } 00100 else if (input==buttonB){ 00101 Farkle.changeDieArray(); 00102 Farkle.startGame(); 00103 currState=Initial; 00104 input=none; 00105 } 00106 else if (input==buttonC){ 00107 currState=Initial; 00108 input=none; 00109 } 00110 else if (input==shake){ 00111 currState=Roll; 00112 input=none; 00113 } 00114 else if (input==none){ 00115 currState=Initial; 00116 input=none; 00117 } 00118 } 00119 00120 continue; 00121 } 00122 //----------------------------------------------------------------// 00123 case(Roll):{ 00124 int numarray[7]={};//create an array keeping track of how many of each number there are for numbers on die faces 00125 uLCD.cls();//clear screen 00126 int tempScore; 00127 mySpeaker.PlayNote(300.0,0.01,0.005); //make a sound 00128 00129 for(int i=0;i<6;i++){ 00130 int number=0; 00131 if((Farkle.getDieArray())[i].getCurrDieState()==ROLL){//create the numarray based on die face numbers 00132 (Farkle.getDieArray())[i].rollDie(); 00133 (Farkle.getDieArray())[i].drawDie(); 00134 number=(Farkle.getDieArray())[i].getValue(); 00135 numarray[number]+=1; 00136 } 00137 } 00138 tempScore=Farkle.calcScore(numarray);//use calculate score function to make a tempscore that will be added to turnscore 00139 if (tempScore==0){ 00140 turnScore=0; 00141 uLCD.color(WHITE); 00142 uLCD.text_width(2); 00143 uLCD.text_height(2); 00144 uLCD.locate(1,6); 00145 uLCD.printf("FARKLE");//if score==0 we have a farkle 00146 } 00147 else{ 00148 turnScore+=tempScore; 00149 uLCD.color(WHITE); 00150 uLCD.text_width(1); 00151 uLCD.text_height(1); 00152 uLCD.locate(0,11); 00153 uLCD.printf("Roll Score: %d", tempScore); 00154 uLCD.printf("\nTurn Score: %d", turnScore); 00155 } 00156 //------------change states based on input---------------------// 00157 while(currState==Roll){ 00158 if (input==buttonC){ 00159 currState=Initial; 00160 input=none; 00161 } 00162 if (input==buttonA){ 00163 currState=Display; 00164 input=none; 00165 } 00166 } 00167 00168 continue; 00169 } 00170 case(Display):{ 00171 uLCD.cls(); 00172 Farkle.setScore(turnScore);//set farkle score data member to turnScore 00173 Farkle.printScore();//print the score 00174 for(int i=0;i<6;i++){//reset all die to be rollable if any are currently removed 00175 (Farkle.getDieArray())[i].setCurrDieState(ROLL); 00176 } 00177 //----------change state based on inputs----------------------// 00178 while(currState==Display){ 00179 if(input==buttonA){ 00180 turnScore=0; 00181 currState=Initial; 00182 input=none; 00183 } 00184 else{ 00185 currState=Display; 00186 00187 } 00188 } 00189 continue; 00190 } 00191 } 00192 } 00193 00194 00195 00196 00197 }
Generated on Sat Oct 29 2022 15:46:27 by
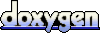