
Game for Project 2
Dependencies: 4DGL-uLCD-SE SDFileSystem mbed wave_player
main.cpp
00001 // Include header files for platform 00002 #include "mbed.h" 00003 #include "wave_player.h" 00004 #include "SDFileSystem.h" 00005 #include "Shiftbrite.h" 00006 #include <vector> 00007 00008 // Include header files for pacman project 00009 #include "globals.h" 00010 #include "map_public.h " 00011 #include "robot.h" 00012 00013 //Platform initialization 00014 DigitalIn left_pb(p24); // push bottom 00015 DigitalIn right_pb(p21); // push bottom 00016 DigitalIn up_pb(p22); // push bottom 00017 DigitalIn down_pb(p23); // push bottom 00018 uLCD_4DGL uLCD(p9,p10,p11); // LCD (serial tx, serial rx, reset pin;) 00019 00020 DigitalOut myled(LED1); 00021 00022 int main() 00023 { 00024 // Initialize the timer 00025 /// [Example of time control implementation] 00026 /// Here is a rough example to implement the timer control <br><br> 00027 int tick, pre_tick; 00028 srand (time(NULL)); 00029 Timer timer; 00030 timer.start(); 00031 tick = timer.read_ms(); 00032 pre_tick = tick; 00033 00034 // Initialize the buttons 00035 left_pb.mode(PullUp); // The variable left_pb will be zero when the pushbutton for moving the player left is pressed 00036 right_pb.mode(PullUp); // The variable rightt_pb will be zero when the pushbutton for moving the player right is pressed 00037 up_pb.mode(PullUp); //the variable fire_pb will be zero when the pushbutton for firing a missile is pressed 00038 down_pb.mode(PullUp); //the variable fire_pb will be zero when the pushbutton for firing a missile is pressed 00039 00040 00041 /// [Example of the game control implementation] 00042 /// Here is the example to initialize the game <br><br> 00043 uLCD.cls(); 00044 map_init(); 00045 robot_init(0,8); 00046 double x=0; 00047 double y=8; 00048 00049 /// 1. Begin the game loop 00050 while(1) { 00051 tick = timer.read_ms(); // Read current time 00052 00053 /// 2. Implement the code to get user input and update the Pacman 00054 /// -[Hint] Implement the code to move Pacman. You could use either push-button or accelerometer. <br> 00055 00056 // [Some hints for user-input detection] 00057 //acc.readXYZGravity(&x,&y,&z); //read accelerometer 00058 uLCD.locate(0,1); 00059 uLCD.printf("Score x%4.1f ",1); //You could remove this code if you already make the accelerometer work. 00060 /// -[Hint] Here is a simple way to utilize the readings of accelerometer: 00061 /// If x is larger than certain value (ex:0.3), then make the Pacman move right. 00062 /// If x<-0.3, then make it move left. <br> 00063 00064 00065 if((tick-pre_tick)>500) { // Time step control 00066 pre_tick = tick; // update the previous tick 00067 00068 /// 3. Update the Pacman on the screen 00069 /// -[Hint] You could update the position of Pacman (draw it on the screen) here based on the user-input at step 2. <br> 00070 if (!up_pb) { //MOVE UP 00071 robot_clear(x,y); 00072 map_draw_grid(x,y); 00073 if (y!=0) { 00074 y=y-1; 00075 } else { 00076 y=15; 00077 } 00078 wait(0.1); 00079 robot_init(x,y); 00080 } else if(!down_pb) { //MOVE DOWN 00081 robot_clear(x,y); 00082 map_draw_grid(x,y); 00083 if(y!=15) { 00084 y=y+1; 00085 } else { 00086 y=0; 00087 } 00088 wait(0.1); 00089 robot_init(x,y); 00090 } else if (!left_pb) { //MOVE LEFT 00091 robot_clear(x,y); 00092 map_draw_grid(x,y); 00093 if (x!=0) { 00094 x-=1; 00095 } 00096 wait(0.1); 00097 robot_init(x,y); 00098 00099 } else if(!right_pb) { //MOVE RIGHT 00100 robot_clear(x,y); 00101 map_draw_grid(x,y); 00102 if (x!=16) { 00103 x+=1; 00104 } 00105 wait(0.1); 00106 robot_init(x,y); 00107 } 00108 00109 00110 00111 /// 4. Implement the code to check the end of game. 00112 00113 } 00114 } 00115 }
Generated on Tue Aug 2 2022 11:28:12 by
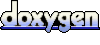