TCP Server that can be used as a debug interface
Fork of TCPDebug by
Debug.cpp
00001 /* 00002 ** File name: Debug.cpp 00003 ** Descriptions: TCP Server that sends debug messages to a connected client 00004 ** 00005 **------------------------------------------------------------------------------------------------------ 00006 ** Created by: Ivan Shindev 00007 ** Created date: 06/11/2013 00008 ** Version: 1.0 00009 ** Descriptions: The original version 00010 ** 00011 **------------------------------------------------------------------------------------------------------ 00012 ** Modified by: 00013 ** Modified date: 00014 ** Version: 00015 ** Descriptions: 00016 ********************************************************************************************************/ 00017 /* How to use the library 00018 Include Debug.h in any file that sends debugging messages 00019 00020 Start the debugging server in the main 00021 initDebug(); //initialize Debug server 00022 startDebug(debug_port); //start the Debug server 00023 while(!isDebugConnected()) //do nothing until a debug client is connected (optional) 00024 { 00025 00026 } 00027 00028 Send a debugging message to the connected client using sendDebugMessage(char *message) 00029 Example: sendDebugMessage("Initialization failed"); 00030 00031 00032 */ 00033 00034 #include "Debug.h" 00035 #include "mbed.h" 00036 00037 int _debug_socket; 00038 int _debug_connected; 00039 Mutex debug_mutex; 00040 /* sendDebugMessage(char *message) sends a message to the connected client 00041 00042 */ 00043 void sendDebugMessage(char *message) 00044 { 00045 00046 lwip_send(_debug_socket ,message,strlen(message)*sizeof(char),0); 00047 } 00048 00049 /* connectDebug(void const *port) is the main Debug server Thread 00050 00051 */ 00052 void connectDebug(void const *port) 00053 { 00054 int _port=(int) port; 00055 int socket_server; 00056 struct sockaddr_in localHost; 00057 memset(&localHost, 0, sizeof(localHost)); 00058 00059 if( (socket_server= lwip_socket(AF_INET, SOCK_STREAM, 0))<0) 00060 { 00061 // return -1; 00062 } 00063 localHost.sin_family = AF_INET; 00064 localHost.sin_port = htons(_port); //port 00065 localHost.sin_addr.s_addr = INADDR_ANY; //localhost address 00066 00067 if (lwip_bind(socket_server, (const struct sockaddr *) &localHost, sizeof(localHost)) < 0) { 00068 00069 // return -1; 00070 } 00071 if (lwip_listen(socket_server,5)<0) 00072 { 00073 00074 perror("listen"); 00075 exit(EXIT_FAILURE); 00076 } 00077 00078 socklen_t newSockRemoteHostLen = sizeof(localHost); 00079 00080 while(1) 00081 { 00082 int nn = lwip_accept(socket_server, (struct sockaddr*) &localHost, &newSockRemoteHostLen); 00083 debug_mutex.lock(); 00084 _debug_connected=1; 00085 debug_mutex.unlock(); 00086 _debug_socket=nn; 00087 sendDebugMessage("You are now connected to Debug Server"); 00088 00089 00090 00091 } 00092 } 00093 00094 osThreadDef(connectDebug, osPriorityNormal, DEFAULT_STACK_SIZE); 00095 00096 /* startDebug(int port) starts the debugging server in a new Thread 00097 00098 */ 00099 void startDebug(int port) 00100 { 00101 osThreadCreate(osThread(connectDebug), (void *) port); 00102 } 00103 00104 00105 00106 /* initDebug() initializes the _debug_connected variable 00107 00108 */ 00109 void initDebug() 00110 { 00111 debug_mutex.lock(); 00112 _debug_connected=0; 00113 debug_mutex.unlock(); 00114 } 00115 00116 /* isDebugConnected() returns 1 if a debug client is connected 00117 00118 */ 00119 int isDebugConnected() 00120 { 00121 int temp; 00122 debug_mutex.lock(); 00123 temp=_debug_connected; 00124 debug_mutex.unlock(); 00125 return temp; 00126 }
Generated on Wed Jul 13 2022 07:17:16 by
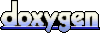