Forked mbed official WiflyInterface (interface for Roving Networks Wifly modules) which includes the possibility to use TCPSocketServer::accept as a non-blocking cal.
Dependents: WiFlyHTTPServerSample MultiThreadingHTTPServer
Fork of WiflyInterface by
Wifly.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 * @section DESCRIPTION 00019 * 00020 * Wifly RN131-C, wifi module 00021 * 00022 * Datasheet: 00023 * 00024 * http://dlnmh9ip6v2uc.cloudfront.net/datasheets/Wireless/WiFi/WiFly-RN-UM.pdf 00025 */ 00026 00027 #ifndef WIFLY_H 00028 #define WIFLY_H 00029 00030 #include "mbed.h" 00031 #include "CBuffer.h" 00032 #include <string> 00033 #include "rtos.h" 00034 00035 #define DEFAULT_WAIT_RESP_TIMEOUT 800 00036 00037 00038 enum Security { 00039 NONE = 0, 00040 WEP_128 = 1, 00041 WPA = 3 00042 }; 00043 00044 enum Protocol { 00045 UDP = (1 << 0), 00046 TCP = (1 << 1) 00047 }; 00048 00049 class Wifly 00050 { 00051 public: 00052 typedef enum 00053 { 00054 Wifly_2400, 00055 Wifly_4800, 00056 Wifly_9600, 00057 Wifly_19200, 00058 Wifly_38400, 00059 Wifly_57600, 00060 Wifly_115200, 00061 Wifly_230400, 00062 Wifly_460800, 00063 Wifly_921600 00064 } WiflyBaudrate_t; 00065 00066 00067 public: 00068 /* 00069 * Constructor 00070 * 00071 * @param tx mbed pin to use for tx line of Serial interface 00072 * @param rx mbed pin to use for rx line of Serial interface 00073 * @param reset reset pin of the wifi module () 00074 * @param tcp_status connection status pin of the wifi module (GPIO 6) 00075 * @param ssid ssid of the network 00076 * @param phrase WEP or WPA key 00077 * @param sec Security type (NONE, WEP_128 or WPA) 00078 */ 00079 Wifly( PinName tx, PinName rx, PinName reset, PinName tcp_status, const char * ssid, const char * phrase, Security sec, WiflyBaudrate_t baud = Wifly_115200); 00080 00081 /* 00082 * Connect the wifi module to the ssid contained in the constructor. 00083 * 00084 * @return true if connected, false otherwise 00085 */ 00086 virtual bool join(); 00087 00088 /* 00089 * Disconnect the wifly module from the access point 00090 * 00091 * @ returns true if successful 00092 */ 00093 bool disconnect(); 00094 00095 /* 00096 * Open a tcp connection with the specified host on the specified port 00097 * 00098 * @param host host (can be either an ip address or a name. If a name is provided, a dns request will be established) 00099 * @param port port 00100 * @ returns true if successful 00101 */ 00102 bool connect(const char * host, int port); 00103 00104 00105 /* 00106 * Set the protocol (UDP or TCP) 00107 * 00108 * @param p protocol 00109 * @ returns true if successful 00110 */ 00111 bool setProtocol(Protocol p); 00112 00113 /* 00114 * Reset the wifi module 00115 */ 00116 void reset(); 00117 00118 /* 00119 * Reboot the wifi module 00120 */ 00121 bool reboot(); 00122 00123 /* 00124 * Check if characters are available 00125 * 00126 * @return number of available characters 00127 */ 00128 int readable(); 00129 00130 /* 00131 * Check if characters are available 00132 * 00133 * @return number of available characters 00134 */ 00135 int writeable(); 00136 00137 /* 00138 * Check if a tcp link is active 00139 * 00140 * @returns true if successful 00141 */ 00142 bool is_connected(); 00143 00144 /* 00145 * Read a character 00146 * 00147 * @return the character read 00148 */ 00149 char getc(); 00150 00151 /* 00152 * Peek a character 00153 * 00154 * @return the character but don't remove it from the queue 00155 */ 00156 char peek(); 00157 00158 /* 00159 * Flush the buffer 00160 */ 00161 void flush(); 00162 00163 /* 00164 * Write a character 00165 * 00166 * @param the character which will be written 00167 */ 00168 int putc(char c); 00169 00170 00171 /* 00172 * To enter in command mode (we can configure the module) 00173 * 00174 * @return true if successful, false otherwise 00175 */ 00176 bool cmdMode(); 00177 00178 /* 00179 * To exit the command mode 00180 * 00181 * @return true if successful, false otherwise 00182 */ 00183 bool exit(bool flush = true); 00184 00185 /* 00186 * Close a tcp connection 00187 * 00188 * @ returns true if successful 00189 */ 00190 bool close(); 00191 00192 /* 00193 * Send a string to the wifi module by serial port. This function desactivates the user interrupt handler when a character is received to analyze the response from the wifi module. 00194 * Useful to send a command to the module and wait a response. 00195 * 00196 * 00197 * @param str string to be sent 00198 * @param len string length 00199 * @param ACK string which must be acknowledge by the wifi module. If ACK == NULL, no string has to be acknoledged. (default: "NO") 00200 * @param res this field will contain the response from the wifi module, result of a command sent. This field is available only if ACK = "NO" AND res != NULL (default: NULL) 00201 * 00202 * @return true if ACK has been found in the response from the wifi module. False otherwise or if there is no response in 5s. 00203 */ 00204 virtual int send(const char * str, int len, const char * ACK = NULL, char * res = NULL, int timeout = DEFAULT_WAIT_RESP_TIMEOUT); 00205 00206 virtual int sendData(const char* str, int len, int timeout = DEFAULT_WAIT_RESP_TIMEOUT); 00207 00208 /* 00209 * Send a command to the wify module. Check if the module is in command mode. If not enter in command mode 00210 * 00211 * @param str string to be sent 00212 * @param ACK string which must be acknowledge by the wifi module. If ACK == NULL, no string has to be acknoledged. (default: "NO") 00213 * @param res this field will contain the response from the wifi module, result of a command sent. This field is available only if ACK = "NO" AND res != NULL (default: NULL) 00214 * 00215 * @returns true if successful 00216 */ 00217 bool sendCommand(const char * cmd, const char * ack = NULL, char * res = NULL, int timeout = DEFAULT_WAIT_RESP_TIMEOUT); 00218 00219 /* 00220 * Return true if the module is using dhcp 00221 * 00222 * @returns true if the module is using dhcp 00223 */ 00224 bool isDHCP() { 00225 return state.dhcp; 00226 } 00227 00228 /* 00229 * Enable Network time and RTC functionality 00230 * 00231 * @param minutes : frequency in minutes with which to query the NTP, or 0 to disable, or 1 to query just once after power-up 00232 * 00233 * @returns 0 if successful, -1 otherwise 00234 */ 00235 int enableTime(int minutes, const char* ntp_address = "65.55.56.206"); 00236 00237 /** Get the time from the Real Time Clock 00238 * 00239 * @ param uptime : if true, returns the uptime (time since last power-up. Returns the Real Time Clock's time otherwise 00240 * 00241 * @ returns the time from RTC 00242 */ 00243 std::string getTime(bool uptime); 00244 00245 bool gethostbyname(const char * host, char * ip); 00246 00247 static Wifly * getInstance() { 00248 return inst; 00249 }; 00250 00251 protected: 00252 00253 /** Set the baud rate for communication 00254 * 00255 * @param baud : sets the baud rate for communication between wifly and host computer 00256 * 00257 * @ returns true if successful, false otherwise 00258 */ 00259 bool setBaudRate(int baud); 00260 WiflyBaudrate_t m_baudrate; 00261 00262 Serial wifi; 00263 DigitalOut reset_pin; 00264 DigitalIn tcp_status; 00265 char phrase[30]; 00266 char ssid[30]; 00267 const char * ip; 00268 const char * netmask; 00269 const char * gateway; 00270 int channel; 00271 CircBuffer<char> buf_wifly; 00272 00273 static Wifly * inst; 00274 00275 virtual void attach_rx(bool null) = 0 ; 00276 virtual void handler_rx(void) = 0; 00277 00278 typedef struct STATE { 00279 bool associated; 00280 bool tcp; 00281 bool dhcp; 00282 Security sec; 00283 Protocol proto; 00284 bool cmd_mode; 00285 } State; 00286 00287 State state; 00288 char * getStringSecurity(); 00289 }; 00290 00291 #endif
Generated on Sat Jul 16 2022 16:48:24 by
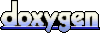