Implementation of the WifiPlusClick hardware module.
Dependents: WifiPlusKlickExample
WifiPlusClick.cpp
00001 #include "mbed.h" 00002 #include "WifiPlusClick.h" 00003 00004 00005 00006 WifiPlusClick::WifiPlusClick(PinName tx, PinName rx, PinName rst, bool dhcp, const char * ssid, WPA_SECURITY_t sec, const char* passphrase, int plen) 00007 : Wifi(tx, rx, rst) 00008 { 00009 char ChannelList[11] = {1,2,5,6,7,8,9,10,11, 12, 13}; 00010 Reset(); 00011 wait(.8); 00012 00013 // Set to european domain 00014 SetRegionalDomain(ETSI); 00015 SetChannelList(5, ChannelList); 00016 SetARPTime(1); 00017 SetRetryCount(20,20); 00018 00019 SetSSID(1, ssid); 00020 SetSecurityWPA(1, sec, plen, passphrase); 00021 } 00022 00023 bool WifiPlusClick::connect() 00024 { 00025 SetNetworkMode(1, INFRASTRUCTURE); 00026 SetIpAddress(true, NULL); 00027 00028 Connect(1); 00029 00030 return AwaitConnected(60); 00031 } 00032 00033 bool WifiPlusClick::connect(IPADDRESS_t *ipAddress, IPADDRESS_t *subnetMask, IPADDRESS_t *ipGateway) 00034 { 00035 if ((ipAddress == NULL) || (subnetMask == NULL) || (ipGateway == NULL)) 00036 return false; 00037 00038 SetNetworkMode(1, ADHOC); 00039 SetIpAddress(false, ipAddress); 00040 SetSubnetMask(subnetMask); 00041 SetGatewayIpAddress(ipGateway); 00042 00043 Connect(1); 00044 00045 return AwaitConnected(60); 00046 } 00047 00048 bool WifiPlusClick::disconnect() 00049 { 00050 Disconnect(); 00051 00052 return AwaitDisconnected(60); 00053 }
Generated on Tue Jul 12 2022 23:18:35 by
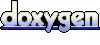