Working Version of the Real Time Clock module DS1307.
Dependents: Rtc_Ds1307_Sample TAREA_5_PROCESADORES Rtc_Ds1307_lcd_alarma Rtc_Ds1307_Reloj_con_alarma_aplazable ... more
Rtc_Ds1307.h
00001 /* Rtc_Ds1307.h */ 00002 /* 00003 Copyright (c) 2013 Henry Leinen (henry[dot]leinen [at] online [dot] de) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 #ifndef __RTC_DS1307_H__ 00024 #define __RTC_DS1307_H__ 00025 00026 00027 00028 /** Class Rtc_Ds1307 implements the real time clock module DS1307 00029 * 00030 * You can read the clock and set a new time and date. 00031 * It is also possible to start and stop the clock. 00032 * Rtc_Ds1307 allows you to display the time in a 12h or 24h format 00033 */ 00034 class Rtc_Ds1307 00035 { 00036 public: 00037 /** Structure which is used to exchange the time and date 00038 */ 00039 typedef struct { 00040 int sec ; /*!< seconds [0..59] */ 00041 int min ; /*!< minutes {0..59] */ 00042 int hour ; /*!< hours [0..23] */ 00043 int wday ; /*!< weekday [1..7, where 1 = sunday, 2 = monday, ... */ 00044 int date ; /*!< day of month [0..31] */ 00045 int mon ; /*!< month of year [1..12] */ 00046 int year ; /*!< year [2000..2255] */ 00047 } Time_rtc; 00048 00049 00050 /** RateSelect specifies the valid frequency values for the square wave output 00051 */ 00052 typedef enum { 00053 RS1Hz = 0, 00054 RS4kHz = 1, 00055 RS8kHz = 2, 00056 RS32kHz = 3 00057 } SqwRateSelect_t; 00058 00059 protected: 00060 I2C* m_rtc; 00061 00062 static const char *m_weekDays[]; 00063 00064 public: 00065 /** public constructor which creates the real time clock object 00066 * 00067 * @param sda : specifies the pin for the SDA communication line. 00068 * 00069 * @param scl : the pin for the serial clock 00070 * 00071 */ 00072 Rtc_Ds1307(PinName sda, PinName scl); 00073 00074 ~Rtc_Ds1307(); 00075 00076 /** Read the current time from RTC chip 00077 * 00078 * @param time : reference to a struct tm which will be filled with the time from rtc 00079 * 00080 * @returns true if successful, otherwise an acknowledge error occured 00081 */ 00082 virtual bool getTime(Time_rtc& time); 00083 00084 /** Write the given time onto the RTC chip 00085 * 00086 * @param time : refereence to a struct which contains valid date and time information 00087 * 00088 * @param start : contains true if the clock shall start (or keep on running). 00089 * 00090 * @param thm : 12-hour-mode if set to true, otherwise 24-hour-mode will be set. 00091 * 00092 * @returns true if successful, otherwise an acknowledge error occured 00093 */ 00094 virtual bool setTime(Time_rtc& time, bool start, bool thm); 00095 00096 /** Start the clock. Please note that the seconds register need to be read and 00097 * written in order to start or stop the clock. This can lead to an error 00098 * in the time value. The recommended way of starting and stoping the clock is 00099 * to write the actual date and time and set the start bit accordingly. 00100 * 00101 * @returns true if the clock was started, false if a communication error occured 00102 */ 00103 bool startClock(); 00104 00105 /** Stop the clock. Please note that the seconds register need to be read and 00106 * written in order to start or stop the clock. This can lead to an error 00107 * in the time value. The recommended way of starting and stoping the clock is 00108 * to write the actual date and time and set the start bit accordingly. 00109 * 00110 * @returns true if the clock was stopped, false if a communication error occured 00111 */ 00112 bool stopClock(); 00113 00114 /** Service function to convert a weekday into a string representation 00115 * 00116 * @param wday : day of week to convert (starting with sunday = 1, monday = 2, ..., saturday = 7 00117 * 00118 * @returns the corresponding string representation 00119 */ 00120 const char* weekdayToString( int wday ) { 00121 return m_weekDays[wday%7]; 00122 } 00123 00124 /** Enable Square Wave output. The function enables or disables the square wave output 00125 * of the module and sets the desired frequency. 00126 * 00127 * @param ena : if set to true, the square wave output is enabled. 00128 * 00129 * @param rs : rate select, can be either one of the four values defined by type /c RateSelect_t 00130 * 00131 * @return true if the operation was successful or false otherwise 00132 */ 00133 bool setSquareWaveOutput(bool ena, SqwRateSelect_t rs); 00134 00135 private: 00136 bool read(int address, char* buffer, int len); 00137 bool write(int address, char* buffer, int len); 00138 00139 static int bcdToDecimal(int bcd) { 00140 return ((bcd&0xF0)>>4)*10 + (bcd&0x0F); 00141 } 00142 00143 static int decimalToBcd(int dec) { 00144 return (dec%10) + ((dec/10)<<4); 00145 } 00146 }; 00147 00148 00149 00150 typedef void (*RtcCallback_t) (void); 00151 00152 00153 class RtcCls : public Rtc_Ds1307 00154 { 00155 protected: 00156 InterruptIn m_sqw; 00157 bool m_bUseSqw; 00158 time_t m_time; // Only used in case SQW is used 00159 00160 bool m_bAlarmEnabled; 00161 RtcCallback_t m_alarmfunc; 00162 time_t m_alarmTime; 00163 00164 public: 00165 RtcCls(PinName sda, PinName scl, PinName sqw, bool bUseSqw); 00166 00167 protected: 00168 void _callback(void); 00169 00170 public: 00171 time_t getTime(); 00172 virtual bool getTime(Time_rtc& time) { return Rtc_Ds1307::getTime(time); } 00173 void setTime(time_t time); 00174 virtual bool setTime(Time_rtc& time, bool start, bool thm) { return Rtc_Ds1307::setTime(time, start, thm); } 00175 public: 00176 void setAlarm(int nSeconds, RtcCallback_t alarmfunc) { 00177 m_alarmfunc = alarmfunc; 00178 m_alarmTime = m_time + nSeconds; 00179 m_bAlarmEnabled = (alarmfunc == NULL) ? false : true; 00180 } 00181 }; 00182 00183 #endif // __RTC_DS1307_H__
Generated on Thu Jul 14 2022 15:34:48 by
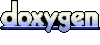