The official mbed C/C SDK provides the software platform and libraries to build your applications.
Fork of mbed by
Serial.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_SERIAL_H 00017 #define MBED_SERIAL_H 00018 00019 #include "platform.h" 00020 00021 #if DEVICE_SERIAL 00022 00023 #include "Stream.h" 00024 #include "FunctionPointer.h" 00025 #include "serial_api.h" 00026 00027 namespace mbed { 00028 00029 /** A serial port (UART) for communication with other serial devices 00030 * 00031 * Can be used for Full Duplex communication, or Simplex by specifying 00032 * one pin as NC (Not Connected) 00033 * 00034 * Example: 00035 * @code 00036 * // Print "Hello World" to the PC 00037 * 00038 * #include "mbed.h" 00039 * 00040 * Serial pc(USBTX, USBRX); 00041 * 00042 * int main() { 00043 * pc.printf("Hello World\n"); 00044 * } 00045 * @endcode 00046 */ 00047 class Serial : public Stream { 00048 00049 public: 00050 /** Create a Serial port, connected to the specified transmit and receive pins 00051 * 00052 * @param tx Transmit pin 00053 * @param rx Receive pin 00054 * 00055 * @note 00056 * Either tx or rx may be specified as NC if unused 00057 */ 00058 Serial(PinName tx, PinName rx, const char *name=NULL); 00059 00060 /** Set the baud rate of the serial port 00061 * 00062 * @param baudrate The baudrate of the serial port (default = 9600). 00063 */ 00064 void baud(int baudrate); 00065 00066 enum Parity { 00067 None = 0, 00068 Odd, 00069 Even, 00070 Forced1, 00071 Forced0 00072 }; 00073 00074 enum IrqType { 00075 RxIrq = 0, 00076 TxIrq 00077 }; 00078 00079 /** Set the transmission format used by the Serial port 00080 * 00081 * @param bits The number of bits in a word (5-8; default = 8) 00082 * @param parity The parity used (Serial::None, Serial::Odd, Serial::Even, Serial::Forced1, Serial::Forced0; default = Serial::None) 00083 * @param stop The number of stop bits (1 or 2; default = 1) 00084 */ 00085 void format(int bits = 8, Parity parity=Serial::None, int stop_bits=1); 00086 00087 /** Determine if there is a character available to read 00088 * 00089 * @returns 00090 * 1 if there is a character available to read, 00091 * 0 otherwise 00092 */ 00093 int readable(); 00094 00095 /** Determine if there is space available to write a character 00096 * 00097 * @returns 00098 * 1 if there is space to write a character, 00099 * 0 otherwise 00100 */ 00101 int writeable(); 00102 00103 /** Attach a function to call whenever a serial interrupt is generated 00104 * 00105 * @param fptr A pointer to a void function, or 0 to set as none 00106 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00107 */ 00108 void attach(void (*fptr)(void), IrqType type=RxIrq); 00109 00110 /** Attach a member function to call whenever a serial interrupt is generated 00111 * 00112 * @param tptr pointer to the object to call the member function on 00113 * @param mptr pointer to the member function to be called 00114 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00115 */ 00116 template<typename T> 00117 void attach(T* tptr, void (T::*mptr)(void), IrqType type=RxIrq) { 00118 if((mptr != NULL) && (tptr != NULL)) { 00119 _irq[type].attach(tptr, mptr); 00120 serial_irq_set(&_serial, (SerialIrq)type, 1); 00121 } 00122 } 00123 00124 static void _irq_handler(uint32_t id, SerialIrq irq_type); 00125 00126 protected: 00127 virtual int _getc(); 00128 virtual int _putc(int c); 00129 00130 serial_t _serial; 00131 FunctionPointer _irq[2]; 00132 }; 00133 00134 } // namespace mbed 00135 00136 #endif 00137 00138 #endif
Generated on Tue Jul 12 2022 21:08:34 by
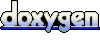