The official mbed C/C SDK provides the software platform and libraries to build your applications.
Fork of mbed by
InterruptIn.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_INTERRUPTIN_H 00017 #define MBED_INTERRUPTIN_H 00018 00019 #include "platform.h" 00020 00021 #if DEVICE_INTERRUPTIN 00022 00023 #include "gpio_api.h" 00024 #include "gpio_irq_api.h" 00025 00026 #include "FunctionPointer.h" 00027 00028 namespace mbed { 00029 00030 /** A digital interrupt input, used to call a function on a rising or falling edge 00031 * 00032 * Example: 00033 * @code 00034 * // Flash an LED while waiting for events 00035 * 00036 * #include "mbed.h" 00037 * 00038 * InterruptIn event(p16); 00039 * DigitalOut led(LED1); 00040 * 00041 * void trigger() { 00042 * printf("triggered!\n"); 00043 * } 00044 * 00045 * int main() { 00046 * event.rise(&trigger); 00047 * while(1) { 00048 * led = !led; 00049 * wait(0.25); 00050 * } 00051 * } 00052 * @endcode 00053 */ 00054 class InterruptIn { 00055 00056 public: 00057 00058 /** Create an InterruptIn connected to the specified pin 00059 * 00060 * @param pin InterruptIn pin to connect to 00061 * @param name (optional) A string to identify the object 00062 */ 00063 InterruptIn(PinName pin); 00064 virtual ~InterruptIn(); 00065 00066 int read(); 00067 #ifdef MBED_OPERATORS 00068 operator int(); 00069 00070 #endif 00071 00072 /** Attach a function to call when a rising edge occurs on the input 00073 * 00074 * @param fptr A pointer to a void function, or 0 to set as none 00075 */ 00076 void rise(void (*fptr)(void)); 00077 00078 /** Attach a member function to call when a rising edge occurs on the input 00079 * 00080 * @param tptr pointer to the object to call the member function on 00081 * @param mptr pointer to the member function to be called 00082 */ 00083 template<typename T> 00084 void rise(T* tptr, void (T::*mptr)(void)) { 00085 _rise.attach(tptr, mptr); 00086 gpio_irq_set(&gpio_irq, IRQ_RISE, 1); 00087 } 00088 00089 /** Attach a function to call when a falling edge occurs on the input 00090 * 00091 * @param fptr A pointer to a void function, or 0 to set as none 00092 */ 00093 void fall(void (*fptr)(void)); 00094 00095 /** Attach a member function to call when a falling edge occurs on the input 00096 * 00097 * @param tptr pointer to the object to call the member function on 00098 * @param mptr pointer to the member function to be called 00099 */ 00100 template<typename T> 00101 void fall(T* tptr, void (T::*mptr)(void)) { 00102 _fall.attach(tptr, mptr); 00103 gpio_irq_set(&gpio_irq, IRQ_FALL, 1); 00104 } 00105 00106 /** Set the input pin mode 00107 * 00108 * @param mode PullUp, PullDown, PullNone 00109 */ 00110 void mode(PinMode pull); 00111 00112 static void _irq_handler(uint32_t id, gpio_irq_event event); 00113 00114 protected: 00115 gpio_t gpio; 00116 gpio_irq_t gpio_irq; 00117 00118 FunctionPointer _rise; 00119 FunctionPointer _fall; 00120 }; 00121 00122 } // namespace mbed 00123 00124 #endif 00125 00126 #endif
Generated on Tue Jul 12 2022 21:08:34 by
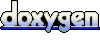