
Updated version of Jim Hamblen's Nokia LCD weather App. Updated to suport Yahoo!'s weather API instead of the Google API. Also removed extraneous Libraries.
Dependencies: NetServices mbed spxml
Fork of weather_Nokia_LCD_display by
NokiaLCD.h
00001 /* mbed NokiaLCD Library, for a 130x130 Nokia colour LCD 00002 * Copyright (c) 2007-2010, sford 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef MBED_NOKIALCD_H 00024 #define MBED_NOKIALCD_H 00025 00026 #include "mbed.h" 00027 00028 /** An interface for the 130x130 Nokia Mobile phone screens 00029 * 00030 * @code 00031 * #include "mbed.h" 00032 * #include "NokiaLCD.h" 00033 * 00034 * NokiaLCD lcd(p5, p7, p8, p9, NokiaLCD::6610); // mosi, sclk, cs, rst, type 00035 * 00036 * int main() { 00037 * lcd.printf("Hello World!"); 00038 * } 00039 * @endcode 00040 */ 00041 class NokiaLCD : public Stream { 00042 00043 public: 00044 /** LCD panel format */ 00045 enum LCDType { 00046 LCD6100 /**< Nokia 6100, as found on sparkfun board (default) */ 00047 , LCD6610 /**< Nokia 6610, as found on olimex board */ 00048 , PCF8833 00049 }; 00050 00051 /** Create and Nokia LCD interface, using a SPI and two DigitalOut interfaces 00052 * 00053 * @param mosi SPI data out 00054 * @param sclk SPI clock 00055 * @param cs Chip Select (DigitalOut) 00056 * @param rst Reset (DigitalOut) 00057 * @param type The LCDType to select driver chip variants 00058 */ 00059 NokiaLCD(PinName mosi, PinName sclk, PinName cs, PinName rst, LCDType type = LCD6100); 00060 00061 #if DOXYGEN_ONLY 00062 /** Write a character to the LCD 00063 * 00064 * @param c The character to write to the display 00065 */ 00066 int putc(int c); 00067 00068 /** Write a formated string to the LCD 00069 * 00070 * @param format A printf-style format string, followed by the 00071 * variables to use in formating the string. 00072 */ 00073 int printf(const char* format, ...); 00074 #endif 00075 00076 /** Locate to a screen column and row 00077 * 00078 * @param column The horizontal position from the left, indexed from 0 00079 * @param row The vertical position from the top, indexed from 0 00080 */ 00081 void locate(int column, int row); 00082 00083 /** Clear the screen and locate to 0,0 */ 00084 void cls(); 00085 00086 /** Set a pixel on te screen 00087 * 00088 * @param x horizontal position from left 00089 * @param y vertical position from top 00090 * @param colour 24-bit colour in format 0x00RRGGBB 00091 */ 00092 void pixel(int x, int y, int colour); 00093 00094 /** Fill an area of the screen 00095 * 00096 * @param x horizontal position from left 00097 * @param y vertical position from top 00098 * @param width width in pixels 00099 * @param height height in pixels 00100 * @param colour 24-bit colour in format 0x00RRGGBB 00101 */ 00102 void fill(int x, int y, int width, int height, int colour); 00103 00104 void blit(int x, int y, int width, int height, const int* colour); 00105 void bitblit(int x, int y, int width, int height, const char* bitstream); 00106 00107 int width(); 00108 int height(); 00109 int columns(); 00110 int rows(); 00111 00112 void reset(); 00113 00114 /** Set the foreground colour 00115 * 00116 * @param c 24-bit colour 00117 */ 00118 void foreground(int c); 00119 00120 /** Set the background colour 00121 * 00122 * @param c 24-bit colour 00123 */ 00124 void background(int c); 00125 00126 protected: 00127 virtual void _window(int x, int y, int width, int height); 00128 virtual void _putp(int colour); 00129 00130 void command(int value); 00131 void data(int value); 00132 00133 void newline(); 00134 virtual int _putc(int c); 00135 virtual int _getc() { 00136 return 0; 00137 } 00138 void putp(int v); 00139 void window(int x, int y, int width, int height); 00140 00141 SPI _spi; 00142 DigitalOut _rst; 00143 DigitalOut _cs; 00144 00145 LCDType _type; 00146 int _row, _column, _rows, _columns, _foreground, _background, _width, _height; 00147 }; 00148 00149 #endif 00150 00151
Generated on Thu Jul 28 2022 03:24:07 by
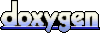