offer some APIs to control the movement of motor. Suitable with Seeed motor shield
Dependents: Seeed_Motor_Shield_HelloWorld Seeed_Motor_Shield
MotorDriver Class Reference
Motor Driver class. More...
#include <MotorDriver.h>
Public Member Functions | |
MotorDriver (PinName int1, PinName int2, PinName int3, PinName int4, PinName speedA, PinName speedB) | |
Create Motor Driver instance. | |
void | init () |
set motor to initialized state | |
void | configure (uint8_t position, uint8_t motorID) |
config position of motor | |
void | setSpeed (uint8_t speed, uint8_t motorID) |
set speed of motor | |
void | setDirection (uint8_t direction, uint8_t motorID) |
set direction of motor, | |
void | rotate (uint8_t direction, uint8_t motor_position) |
rotate motor | |
void | rotateWithID (uint8_t direction, uint8_t motorID) |
rotate motorA or motorB | |
void | goForward () |
make motor go forward | |
void | goBackward () |
make motor go backward | |
void | goLeft () |
make motor go left | |
void | goRight () |
make motor go right | |
void | stop () |
make motor stop | |
void | stop (uint8_t motorID) |
make motorA or motorB stop | |
Data Fields | |
MotorStruct | motorA |
motor A | |
MotorStruct | motorB |
motor B |
Detailed Description
Motor Driver class.
offer API to control the movement of motor
Definition at line 53 of file MotorDriver.h.
Constructor & Destructor Documentation
MotorDriver | ( | PinName | int1, |
PinName | int2, | ||
PinName | int3, | ||
PinName | int4, | ||
PinName | speedA, | ||
PinName | speedB | ||
) |
Create Motor Driver instance.
- Parameters:
-
int1 pin 1 of motor movement control int2 pin 2 of motor movement control int3 pin 3 of motor movement control int4 pin 4 of motor movement control speedA speed control of motorA speedB speed control of motorB
Definition at line 66 of file MotorDriver.h.
Member Function Documentation
void configure | ( | uint8_t | position, |
uint8_t | motorID | ||
) |
config position of motor
- Parameters:
-
position the position set to motor,MOTOR_POSITION_LEFT or MOTOR_POSITION_RIGHT will be allowed motorID the ID define which motor will be set, you can choose MOTORA or MOTORB
Definition at line 37 of file MotorDriver.cpp.
void goBackward | ( | ) |
make motor go backward
Definition at line 82 of file MotorDriver.cpp.
void goForward | ( | ) |
make motor go forward
Definition at line 77 of file MotorDriver.cpp.
void goLeft | ( | ) |
make motor go left
Definition at line 87 of file MotorDriver.cpp.
void goRight | ( | ) |
make motor go right
Definition at line 92 of file MotorDriver.cpp.
void init | ( | ) |
set motor to initialized state
Definition at line 25 of file MotorDriver.cpp.
void rotate | ( | uint8_t | direction, |
uint8_t | motor_position | ||
) |
rotate motor
- Parameters:
-
direction the direction set to motor, MOTOR_CLOCKWISE or MOTOR_ANTICLOCKWISE will be allowed motor_position the position set to motor,MOTOR_POSITION_LEFT or MOTOR_POSITION_RIGHT will be allowed
Definition at line 54 of file MotorDriver.cpp.
void rotateWithID | ( | uint8_t | direction, |
uint8_t | motorID | ||
) |
rotate motorA or motorB
- Parameters:
-
direction the direction set to motor, MOTOR_CLOCKWISE or MOTOR_ANTICLOCKWISE will be allowed motorID the ID define which motor will be set, you can choose MOTORA or MOTORB
Definition at line 64 of file MotorDriver.cpp.
void setDirection | ( | uint8_t | direction, |
uint8_t | motorID | ||
) |
set direction of motor,
- Parameters:
-
direction the direction of motor, MOTOR_CLOCKWISE or MOTOR_ANTICLOCKWISE will be allowed motorID the ID define which motor will be set, you can choose MOTORA or MOTORB
Definition at line 48 of file MotorDriver.cpp.
void setSpeed | ( | uint8_t | speed, |
uint8_t | motorID | ||
) |
set speed of motor
- Parameters:
-
speed speed value set to motor, [0,100] will be allowed motorID the ID define which motor will be set, you can choose MOTORA or MOTORB
Definition at line 43 of file MotorDriver.cpp.
void stop | ( | ) |
make motor stop
Definition at line 98 of file MotorDriver.cpp.
void stop | ( | uint8_t | motorID ) |
make motorA or motorB stop
- Parameters:
-
motorID the ID define which motor will be set, you can choose MOTORA or MOTORB
Definition at line 104 of file MotorDriver.cpp.
Field Documentation
MotorStruct motorA |
motor A
Definition at line 134 of file MotorDriver.h.
MotorStruct motorB |
motor B
Definition at line 138 of file MotorDriver.h.
Generated on Tue Jul 12 2022 21:12:16 by
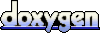